Arduino - Température via le Web
Dans ce tutoriel, nous allons apprendre à programmer un Arduino pour qu'il devienne un serveur web qui vous fournira la température via le web. Vous pouvez accéder à la page web fournie par l'Arduino pour vérifier la température à partir d'un capteur de température DS18B20. Voici comment cela fonctionne :
Arduino est programmé en tant que serveur web.
Vous saisissez l'adresse IP de l'Arduino dans un navigateur web sur votre smartphone ou PC.
Arduino répond à la requête du navigateur web avec une page web qui affiche la température relevée par le capteur DS18B20.
Nous passerons en revue deux exemples de code :
Code Arduino qui fournit une page web très simple affichant la température du capteur DS18B20. Cela vous permet de comprendre facilement son fonctionnement.
Code Arduino qui fournit une page web graphique affichant la température du capteur DS18B20.
Or you can buy the following sensor kits:
Divulgation : Certains des liens fournis dans cette section sont des liens affiliés Amazon. Nous pouvons recevoir une commission pour tout achat effectué via ces liens, sans coût supplémentaire pour vous. Nous vous remercions de votre soutien.
Si vous ne connaissez pas l'Arduino Uno R4 et le capteur de température DS18B20 (brochage, fonctionnement, programmation...), renseignez-vous sur ces derniers dans les tutoriels suivants :
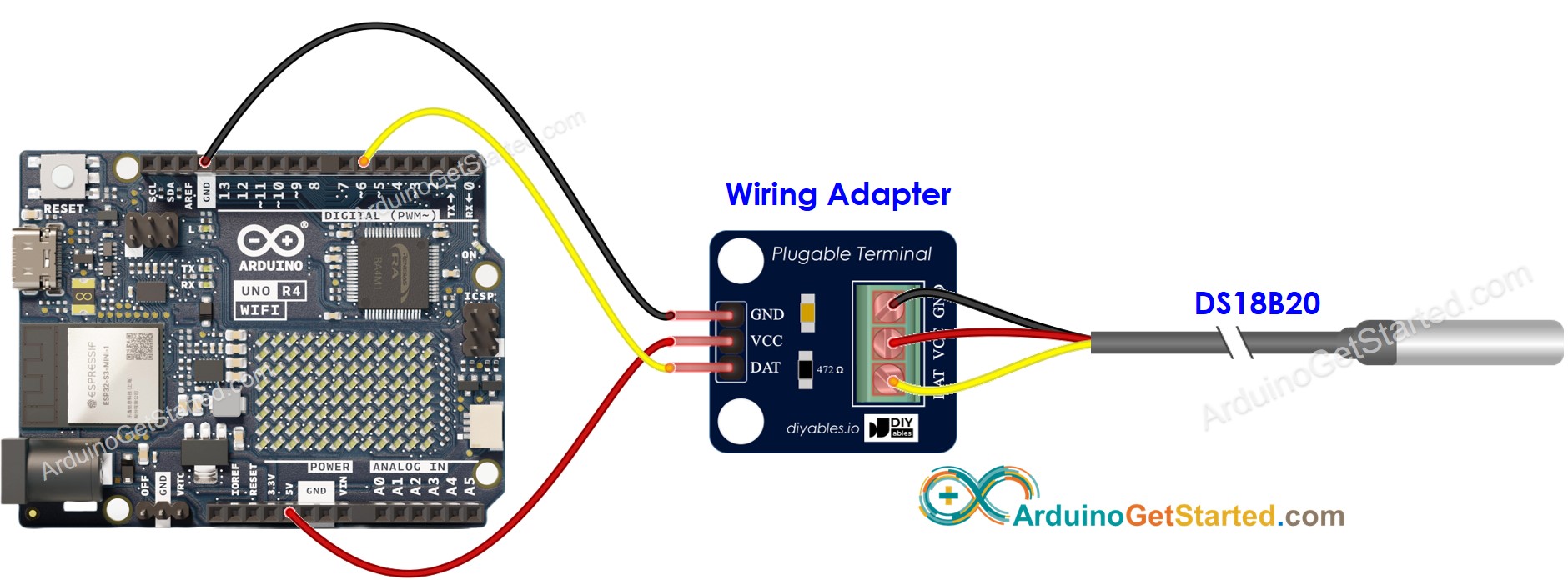
This image is created using Fritzing. Click to enlarge image
#include <WiFiS3.h>
#include <OneWire.h>
#include <DallasTemperature.h>
const char ssid[] = "YOUR_WIFI";
const char pass[] = "YOUR_WIFI_PASSWORD";
const int SENSOR_PIN = 6;
OneWire oneWire(SENSOR_PIN);
DallasTemperature tempSensor(&oneWire);
int status = WL_IDLE_STATUS;
WiFiServer server(80);
float getTemperature() {
tempSensor.requestTemperatures();
float tempCelsius = tempSensor.getTempCByIndex(0);
return tempCelsius;
}
void setup() {
Serial.begin(9600);
tempSensor.begin();
String fv = WiFi.firmwareVersion();
if (fv < WIFI_FIRMWARE_LATEST_VERSION)
Serial.println("Please upgrade the firmware");
while (status != WL_CONNECTED) {
Serial.print("Attempting to connect to SSID: ");
Serial.println(ssid);
status = WiFi.begin(ssid, pass);
delay(10000);
}
server.begin();
printWifiStatus();
}
void loop() {
WiFiClient client = server.available();
if (client) {
while (client.connected()) {
if (client.available()) {
String HTTP_header = client.readStringUntil('\n');
if (HTTP_header.equals("\r"))
break;
Serial.print("<< ");
Serial.println(HTTP_header);
}
}
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close");
client.println();
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<head>");
client.println("<link rel=\"icon\" href=\"data:,\">");
client.println("</head>");
client.println("<p>");
client.print("Temperature: <span style=\"color: red;\">");
float temperature = getTemperature();
client.print(temperature, 2);
client.println("°C</span>");
client.println("</p>");
client.println("</html>");
client.flush();
delay(10);
client.stop();
}
}
void printWifiStatus() {
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
Serial.print("signal strength (RSSI):");
Serial.print(WiFi.RSSI());
Serial.println(" dBm");
}
Copiez le code ci-dessus et ouvrez-le avec Arduino IDE
Modifiez les informations wifi (SSID et mot de passe) dans le code par les vôtres
Cliquez sur le bouton Upload de l'Arduino IDE pour téléverser le code sur Arduino
Ouvrez le moniteur série
Consultez le résultat sur le moniteur série.
Attempting to connect to SSID: YOUR_WIFI
IP Address: 192.168.0.2
signal strength (RSSI):-39 dBm
Attempting to connect to SSID: YOUR_WIFI
IP Address: 192.168.0.2
signal strength (RSSI):-41 dBm
New HTTP Request
<< GET / HTTP/1.1
<< Host: 192.168.0.2
<< Connection: keep-alive
<< Cache-Control: max-age=0
<< Upgrade-Insecure-Requests: 1
<< User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64)
<< Accept: text/html,application/xhtml+xml,application/xml
<< Accept-Encoding: gzip, deflate
<< Accept-Language: en-US,en;q=0.9
Étant donné qu'une page web graphique contient une grande quantité de contenu HTML, l'intégrer dans le code Arduino comme auparavant devient peu pratique. Pour résoudre cela, nous devons séparer le code Arduino et le code HTML dans différents fichiers :
Le code Arduino sera placé dans un fichier .ino.
Le code HTML (y compris HTML, CSS et Javascript) sera placé dans un fichier .h.
Ouvrez l'IDE Arduino et créez un nouveau sketch, donnez-lui un nom, par exemple ArduinoGetStarted.com.ino
Copiez le code ci-dessous et ouvrez-le avec l'IDE Arduino
#include <WiFiS3.h>
#include "index.h"
#include <OneWire.h>
#include <DallasTemperature.h>
const char ssid[] = "YOUR_WIFI";
const char pass[] = "YOUR_WIFI_PASSWORD";
const int SENSOR_PIN = 6;
OneWire oneWire(SENSOR_PIN);
DallasTemperature tempSensor(&oneWire);
int status = WL_IDLE_STATUS;
WiFiServer server(80);
float getTemperature() {
tempSensor.requestTemperatures();
float tempCelsius = tempSensor.getTempCByIndex(0);
return tempCelsius;
}
void setup() {
Serial.begin(9600);
tempSensor.begin();
String fv = WiFi.firmwareVersion();
if (fv < WIFI_FIRMWARE_LATEST_VERSION)
Serial.println("Please upgrade the firmware");
while (status != WL_CONNECTED) {
Serial.print("Attempting to connect to SSID: ");
Serial.println(ssid);
status = WiFi.begin(ssid, pass);
delay(10000);
}
server.begin();
printWifiStatus();
}
void loop() {
WiFiClient client = server.available();
if (client) {
while (client.connected()) {
if (client.available()) {
String HTTP_header = client.readStringUntil('\n');
if (HTTP_header.equals("\r"))
break;
Serial.print("<< ");
Serial.println(HTTP_header);
}
}
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close");
client.println();
float temp = getTemperature();
String html = String(HTML_CONTENT);
html.replace("TEMPERATURE_MARKER", String(temp, 2));
client.println(html);
client.flush();
delay(10);
client.stop();
}
}
void printWifiStatus() {
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
Serial.print("signal strength (RSSI):");
Serial.print(WiFi.RSSI());
Serial.println(" dBm");
}
Changez les informations WiFi (SSID et mot de passe) dans le code pour les vôtres
Créez le fichier index.h sur Arduino IDE en :
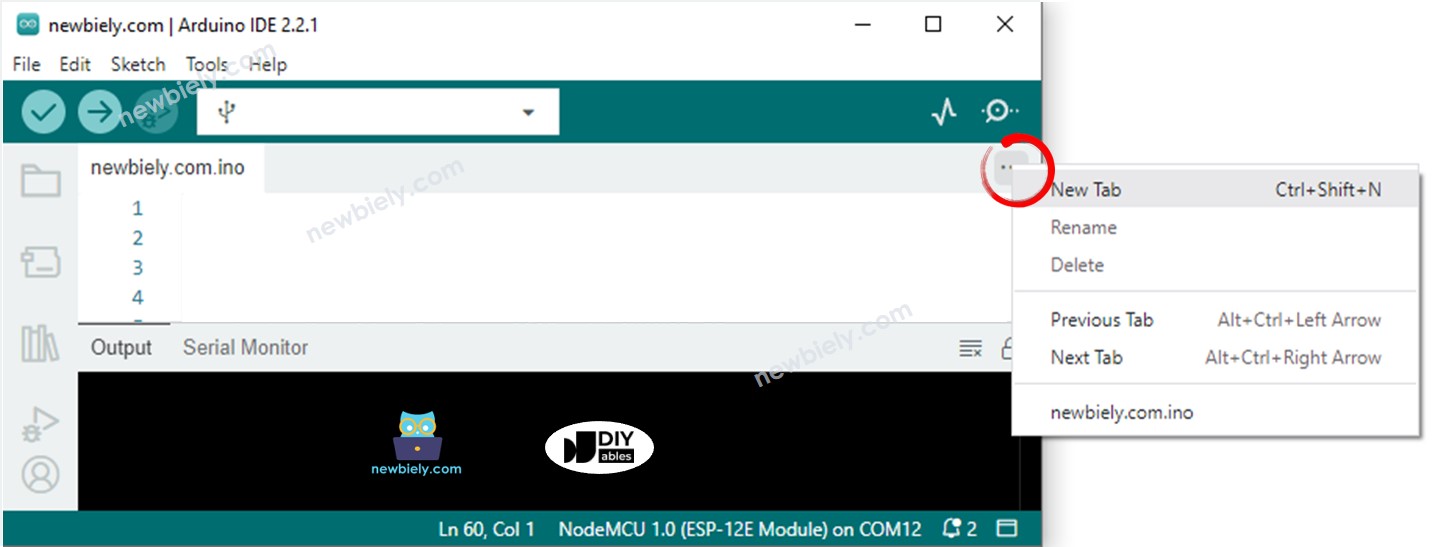
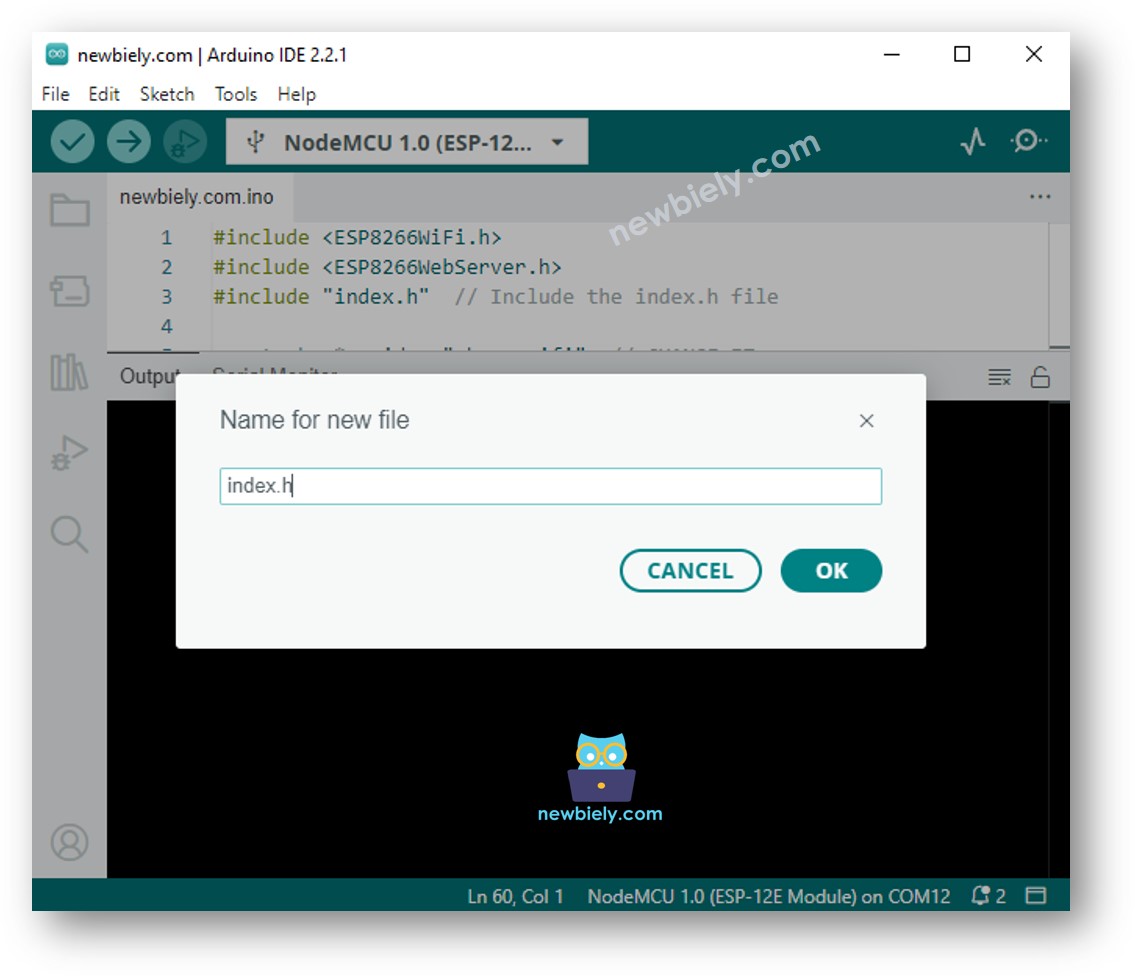
const char *HTML_CONTENT = R""""(
<!DOCTYPE html>
<html>
<head>
<title>Arduino - Web Temperature</title>
<meta name="viewport" content="width=device-width, initial-scale=0.7, maximum-scale=0.7">
<meta charset="utf-8">
<link rel="icon" href="https://diyables.io/images/page/diyables.svg">
<style>
body { font-family: "Georgia"; text-align: center; font-size: width/2pt;}
h1 { font-weight: bold; font-size: width/2pt;}
h2 { font-weight: bold; font-size: width/2pt;}
button { font-weight: bold; font-size: width/2pt;}
</style>
<script>
var cvs_width = 200, cvs_height = 450;
function init() {
var canvas = document.getElementById("cvs");
canvas.width = cvs_width;
canvas.height = cvs_height + 50;
var ctx = canvas.getContext("2d");
ctx.translate(cvs_width/2, cvs_height - 80);
update_view(TEMPERATURE_MARKER);
}
function update_view(temp) {
var canvas = document.getElementById("cvs");
var ctx = canvas.getContext("2d");
var radius = 70;
var offset = 5;
var width = 45;
var height = 330;
ctx.clearRect(-cvs_width/2, -350, cvs_width, cvs_height);
ctx.strokeStyle="blue";
ctx.fillStyle="blue";
var x = -width/2;
ctx.lineWidth=2;
for (var i = 0; i <= 100; i+=5) {
var y = -(height - radius)*i/100 - radius - 5;
ctx.beginPath();
ctx.lineTo(x, y);
ctx.lineTo(x - 20, y);
ctx.stroke();
}
ctx.lineWidth=5;
for (var i = 0; i <= 100; i+=20) {
var y = -(height - radius)*i/100 - radius - 5;
ctx.beginPath();
ctx.lineTo(x, y);
ctx.lineTo(x - 25, y);
ctx.stroke();
ctx.font="20px Georgia";
ctx.textBaseline="middle";
ctx.textAlign="right";
ctx.fillText(i.toString(), x - 35, y);
}
ctx.lineWidth=16;
ctx.beginPath();
ctx.arc(0, 0, radius, 0, 2 * Math.PI);
ctx.stroke();
ctx.beginPath();
ctx.rect(-width/2, -height, width, height);
ctx.stroke();
ctx.beginPath();
ctx.arc(0, -height, width/2, 0, 2 * Math.PI);
ctx.stroke();
ctx.fillStyle="#e6e6ff";
ctx.beginPath();
ctx.arc(0, 0, radius, 0, 2 * Math.PI);
ctx.fill();
ctx.beginPath();
ctx.rect(-width/2, -height, width, height);
ctx.fill();
ctx.beginPath();
ctx.arc(0, -height, width/2, 0, 2 * Math.PI);
ctx.fill();
ctx.fillStyle="#ff1a1a";
ctx.beginPath();
ctx.arc(0, 0, radius - offset, 0, 2 * Math.PI);
ctx.fill();
temp = Math.round(temp * 100) / 100;
var y = (height - radius)*temp/100.0 + radius + 5;
ctx.beginPath();
ctx.rect(-width/2 + offset, -y, width - 2*offset, y);
ctx.fill();
ctx.fillStyle="red";
ctx.font="bold 34px Georgia";
ctx.textBaseline="middle";
ctx.textAlign="center";
ctx.fillText(temp.toString() + "°C", 0, 100);
}
window.onload = init;
</script>
</head>
<body>
<h1>Arduino - Web Temperature</h1>
<canvas id="cvs"></canvas>
</body>
</html>
)"""";
Vous avez maintenant le code dans deux fichiers : ArduinoGetStarted.com.ino et index.h
Cliquez sur le bouton Upload dans l'IDE Arduino pour téléverser le code vers Arduino
Accédez à la page web de la carte Arduino via un navigateur web comme auparavant. Vous le verrez comme ci-dessous :
※ Note:
Si vous apportez des modifications au contenu HTML dans le fichier index.h mais que vous ne modifiez rien dans le fichier ArduinoGetStarted.com.ino, l'IDE Arduino ne rafraîchira ni ne mettra à jour le contenu HTML lorsque vous compilerez et téléverserez le code sur l'ESP32. Pour forcer l'IDE Arduino à mettre à jour le contenu HTML dans cette situation, vous devez apporter une modification dans le fichier ArduinoGetStarted.com.ino. Par exemple, vous pouvez ajouter une ligne vide ou insérer un commentaire. Cette action déclenche l'IDE pour reconnaître qu'il y a eu des changements dans le projet, garantissant que votre contenu HTML mis à jour soit inclus dans le téléversement.