Arduino - Ethernet
Ce tutoriel vous montre comment utiliser le module Arduino et Ethernet pour vous connecter à Internet ou à votre réseau local (LAN). Voici ce que nous aborderons :
Connecter l'Arduino à un module Ethernet W5500
Programmer l'Arduino pour envoyer des requêtes HTTP sur Ethernet
Programmer l'Arduino pour créer un serveur web via Ethernet
Or you can buy the following sensor kits:
Divulgation : Certains des liens fournis dans cette section sont des liens affiliés Amazon. Nous pouvons recevoir une commission pour tout achat effectué via ces liens, sans coût supplémentaire pour vous. Nous vous remercions de votre soutien.
Le module Ethernet W5500 a deux façons de se connecter :
Interface Ethernet RJ45 : Utilisez un câble Ethernet pour vous connecter à un routeur ou un commutateur.
Interface SPI : Utilisez ceci pour vous connecter à une carte Arduino. Elle a 10 broches. Ces broches doivent être connectées à l'Arduino comme indiqué dans le tableau ci-dessous :
Ethenet Module Pins | Arduino Pins |
NC pin | NOT connected |
INT pin | NOT connected |
RST pin | Reset pin |
GND pin | GND pin |
5V pin | 5V pin |
3.3V pin | NOT connected |
MISO pin | 12 (MISO) |
MOSI pin | 11 (MOSI) |
SCS pin | 10 (SS) |
SCLK pin | 13 (SCK) |
image source: diyables.io
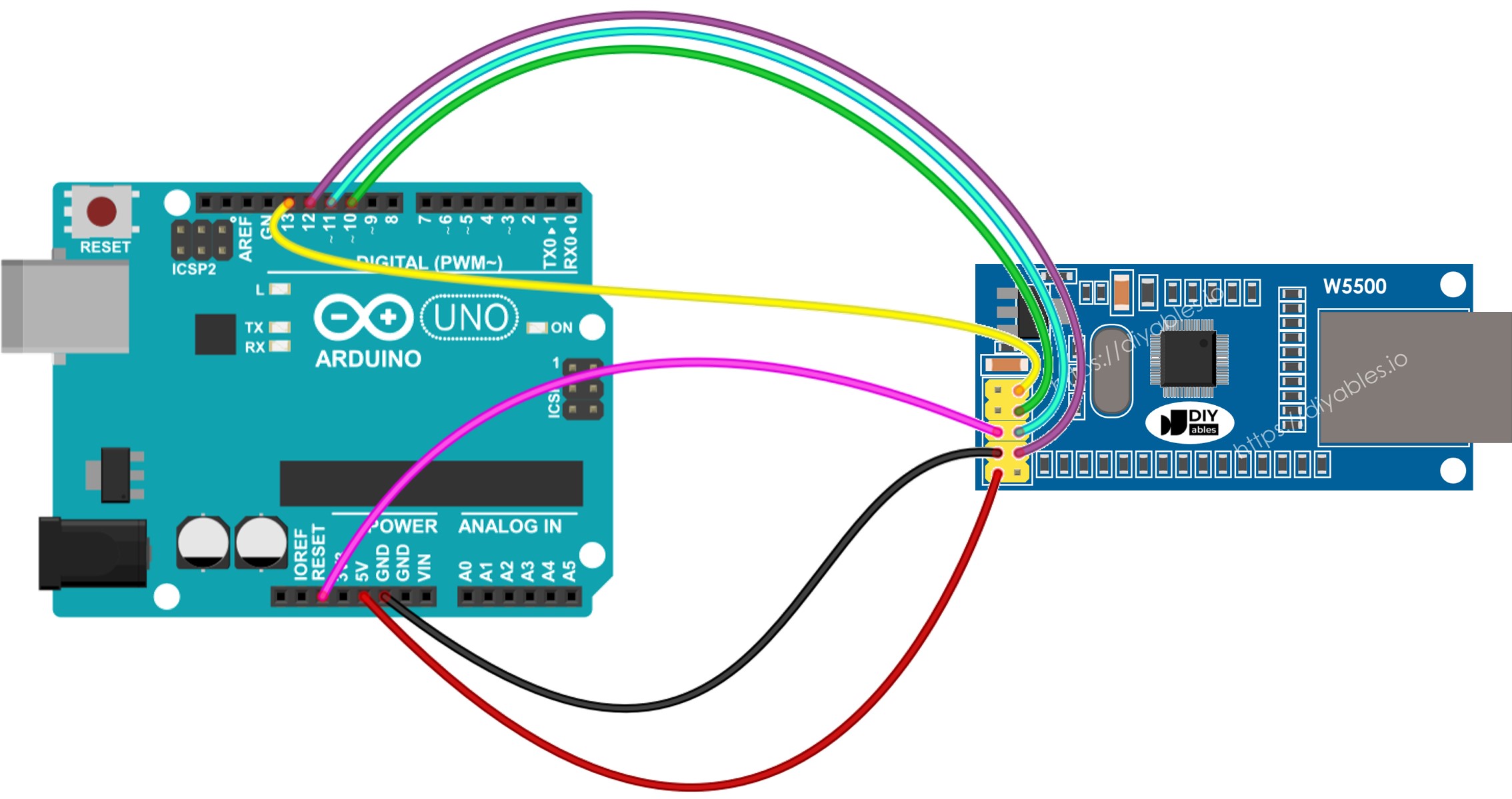
This image is created using Fritzing. Click to enlarge image
La connexion réelle ci-dessous entre Arduino et le module Ethernet W5500.
Ce code agit comme un client web. Il envoie des requêtes HTTP au serveur web situé à http://example.com/.
#include <SPI.h>
#include <Ethernet.h>
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
EthernetClient client;
int HTTP_PORT = 80;
String HTTP_METHOD = "GET";
char HOST_NAME[] = "example.com";
String PATH_NAME = "/";
void setup() {
Serial.begin(9600);
delay(1000);
Serial.println("Arduino - Ethernet Tutorial");
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to obtaining an IP address");
if (Ethernet.hardwareStatus() == EthernetNoHardware)
Serial.println("Ethernet shield was not found");
if (Ethernet.linkStatus() == LinkOFF)
Serial.println("Ethernet cable is not connected.");
while (true)
;
}
if (client.connect(HOST_NAME, HTTP_PORT)) {
Serial.println("Connected to server");
client.println(HTTP_METHOD + " " + PATH_NAME + " HTTP/1.1");
client.println("Host: " + String(HOST_NAME));
client.println("Connection: close");
client.println();
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.print(c);
}
}
client.stop();
Serial.println();
Serial.println("disconnected");
} else {
Serial.println("connection failed");
}
}
void loop() {
}
Veuillez suivre ces étapes une par une :
Connectez le module Ethernet à votre Arduino comme indiqué dans le schéma fourni.
Utilisez un câble Ethernet pour connecter le module Ethernet à votre routeur ou commutateur.
Utilisez un câble USB pour connecter la carte Arduino à votre ordinateur.
Ouvrez l'IDE Arduino sur votre ordinateur.
Choisissez la bonne carte Arduino et le port COM.
Cliquez sur l'icône Libraries sur le côté gauche de l'IDE Arduino.
Dans la zone de recherche, tapez “Ethernet” et trouvez la bibliothèque Ethernet par Various.
Cliquez sur le bouton Install pour ajouter la bibliothèque Ethernet.
Ouvrez le Moniteur Série dans l'IDE Arduino.
Copiez le code fourni et collez-le dans l'IDE Arduino.
Cliquez sur le bouton Upload dans l'IDE Arduino pour transférer le code vers l'Arduino.
Consultez le Moniteur Série où les résultats apparaîtront comme ci-dessous.
Arduino - Ethernet Tutorial
Connected to server
HTTP/1.1 200 OK
Accept-Ranges: bytes
Age: 208425
Cache-Control: max-age=604800
Content-Type: text/html; charset=UTF-8
Date: Fri, 12 Jul 2024 07:08:42 GMT
Etag: "3147526947"
Expires: Fri, 19 Jul 2024 07:08:42 GMT
Last-Modified: Thu, 17 Oct 2019 07:18:26 GMT
Server: ECAcc (lac/55B8)
Vary: Accept-Encoding
X-Cache: HIT
Content-Length: 1256
Connection: close
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8" />
<meta http-equiv="Content-type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this
domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
disconnected
※ Note:
Si un autre appareil sur le même réseau partage votre adresse MAC, il peut y avoir des problèmes.
Le code ci-dessous crée un serveur web sur l'Arduino. Ce serveur web envoie une page web simple aux navigateurs.
#include <SPI.h>
#include <Ethernet.h>
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
EthernetServer server(80);
void setup() {
Serial.begin(9600);
delay(1000);
Serial.println("Arduino - Ethernet Tutorial");
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to obtaining an IP address");
if (Ethernet.hardwareStatus() == EthernetNoHardware)
Serial.println("Ethernet shield was not found");
if (Ethernet.linkStatus() == LinkOFF)
Serial.println("Ethernet cable is not connected.");
while (true)
;
}
server.begin();
Serial.print("Arduino - Web Server IP Address: ");
Serial.println(Ethernet.localIP());
}
void loop() {
EthernetClient client = server.available();
if (client) {
Serial.println("new client");
bool currentLineIsBlank = true;
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.write(c);
if (c == '\n' && currentLineIsBlank) {
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close");
client.println();
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<body>");
client.println("<h1>Arduino - Web Server with Ethernet</h1>");
client.println("</body>");
client.println("</html>");
break;
}
if (c == '\n') {
currentLineIsBlank = true;
} else if (c != '\r') {
currentLineIsBlank = false;
}
}
}
delay(1);
client.stop();
Serial.println("client disconnected");
}
}
Copiez le code ci-dessus et collez-le dans l'IDE Arduino.
Cliquez sur le bouton Upload dans l'IDE Arduino pour téléverser le code sur Arduino.
Vérifiez le résultat sur le Moniteur Série, il s'affichera comme suit :
Arduino - Ethernet Tutorial
Arduino - Web Server IP Address: 192.168.0.2