Arduino - Compteur de Bouton - OLED
Dans ce tutoriel, nous allons utiliser Arduino :
- Comptez le nombre de fois qu'un bouton est pressé
- Affichez le nombre de pressions sur un écran OLED.
- Alignez automatiquement le nombre de pressions verticalement et horizontalement au centre de l'écran OLED.
Dans ce tutoriel, le bouton est également déparasité sans utiliser la fonction delay(). Voir Pourquoi avons-nous besoin de déparasiter?
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos de l'OLED et du bouton
Si vous ne connaissez pas les OLED et les boutons (brochage, fonctionnement, programmation...), renseignez-vous à leur sujet dans les tutoriels suivants :
Diagramme de câblage
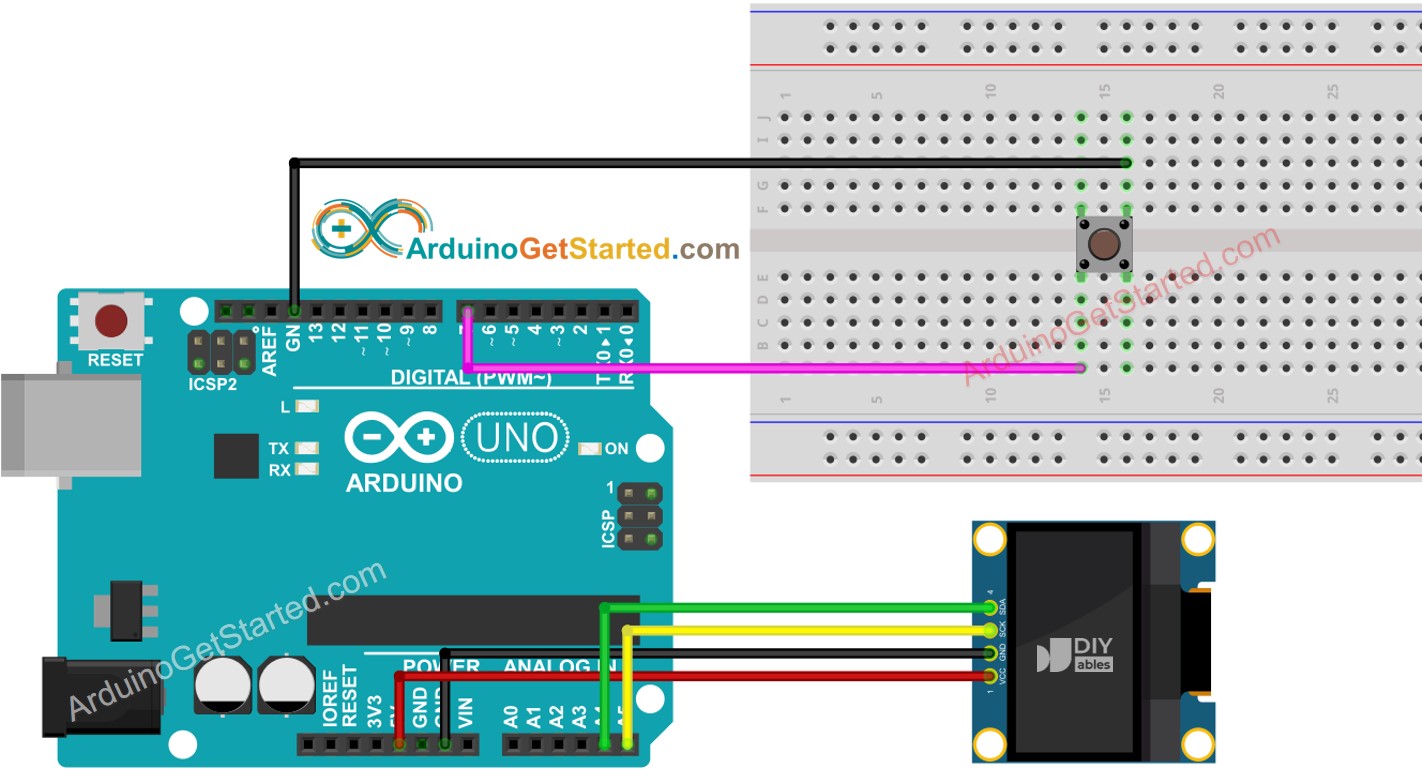
This image is created using Fritzing. Click to enlarge image
Code Arduino - affichage du nombre de clics sur l'OLED
Étapes rapides
- Naviguez vers l'icône Libraries sur la barre latérale gauche de l'IDE Arduino.
- Recherchez "ezButton", puis trouvez la bibliothèque de boutons par ArduinoGetStarted
- Cliquez sur le bouton Install pour installer la bibliothèque ezButton.
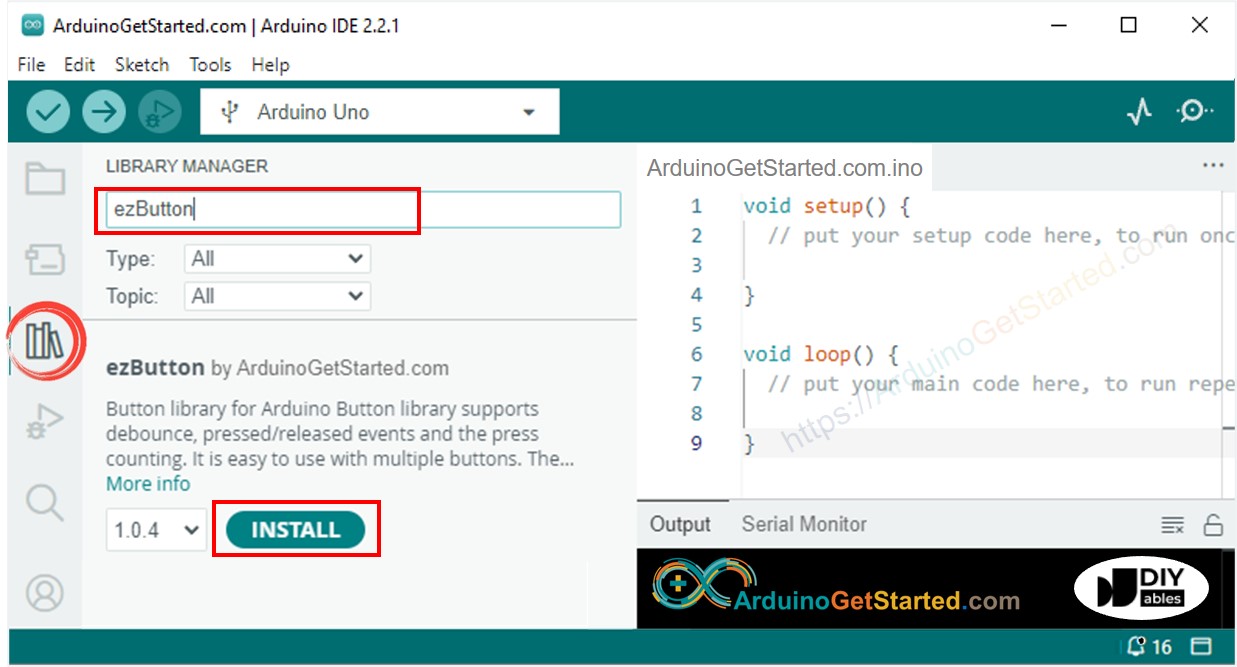
- Recherchez "SSD1306", puis trouvez la bibliothèque SSD1306 par Adafruit.
- Cliquez sur le bouton Install pour installer la bibliothèque.
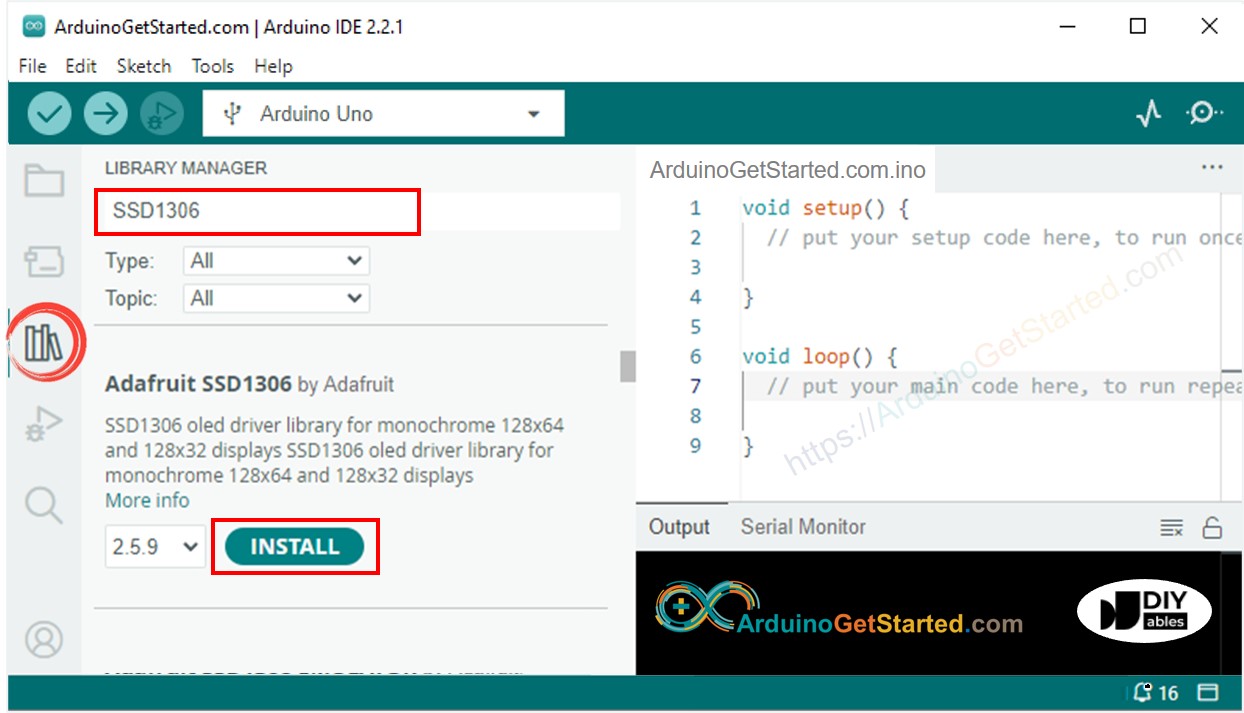
- On vous demandera d'installer d'autres dépendances de bibliothèques
- Cliquez sur le bouton Install All pour installer toutes les dépendances des bibliothèques.
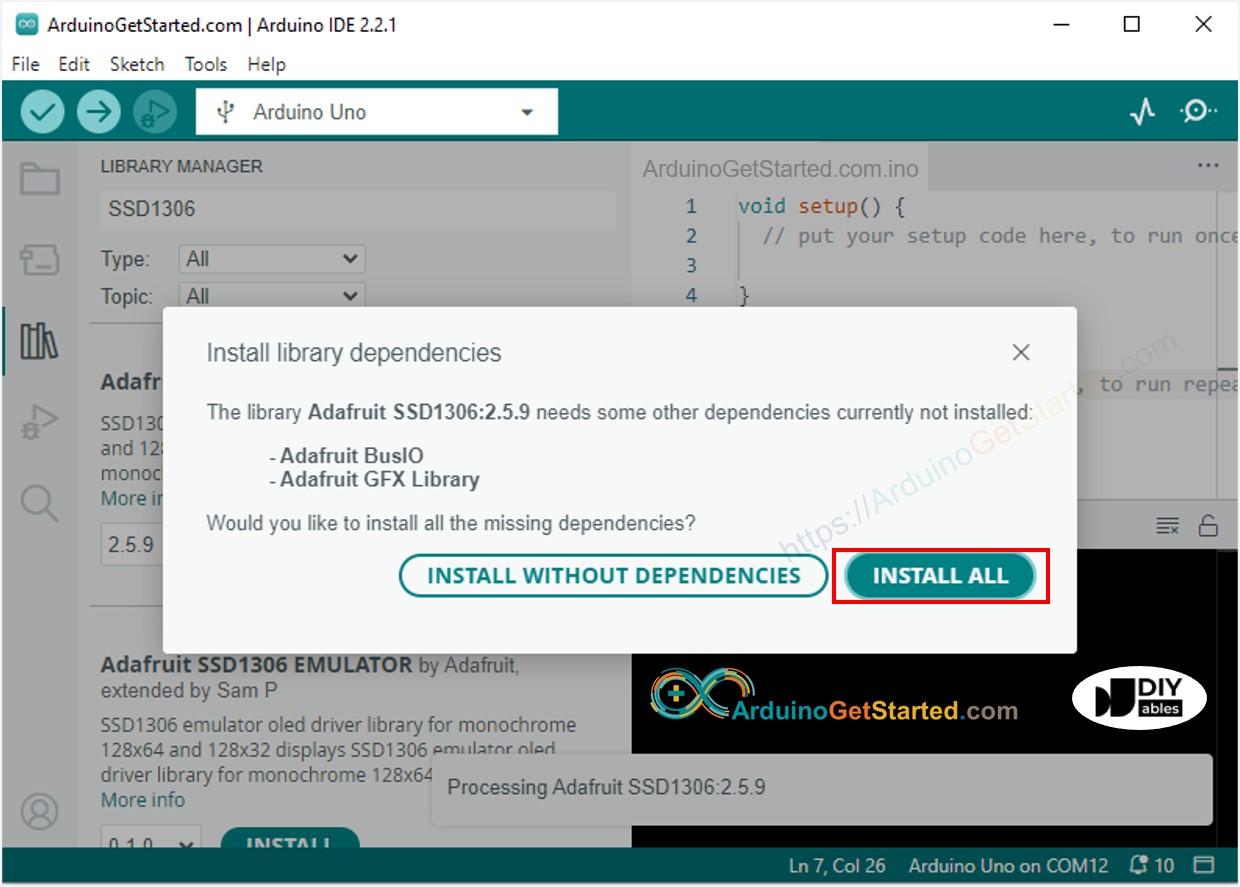
- Copiez le code ci-dessus et ouvrez-le avec l'IDE Arduino
- Cliquez sur le bouton Upload sur l'IDE Arduino pour téléverser le code sur l'Arduino
- Appuyez plusieurs fois sur le bouton
- Observez le changement du nombre de comptage sur l'OLED
Le code ci-dessus affiche simplement le compteur de pressions sur le bouton dans le coin supérieur gauche. Modifions le code pour le centraliser !
Code Arduino - Alignement vertical et horizontal au centre sur OLED
※ Note:
Le code ci-dessus permet de centrer automatiquement le texte horizontalement et verticalement sur l'affichage OLED. Consultez Comment centrer verticalement/horizontalement sur OLED pour plus de détails.