Arduino - OLED
L'écran OLED (diode électroluminescente organique) est une alternative à l'écran LCD. L'OLED est ultra-léger, presque aussi fin que du papier, flexible et produit une image plus lumineuse et plus nette.
Dans ce tutoriel, nous allons apprendre :
- Comment utiliser un afficheur OLED avec Arduino.
- Comment afficher du texte, des nombres sur un OLED en utilisant Arduino
- Comment aligner verticalement et horizontalement du texte, des nombres sur un OLED
- Comment dessiner sur un OLED en utilisant Arduino
- Comment afficher une image sur un OLED en utilisant Arduino
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos de l'écran OLED
Il existe de nombreux types d'écrans OLED. Ils se différencient les uns des autres par leur interface de communication, leurs tailles et leurs couleurs :
- Interface de communication : I2C, SPI
- Taille : 128x64, 128x32...
- Couleur : blanc, bleu, bicolore...
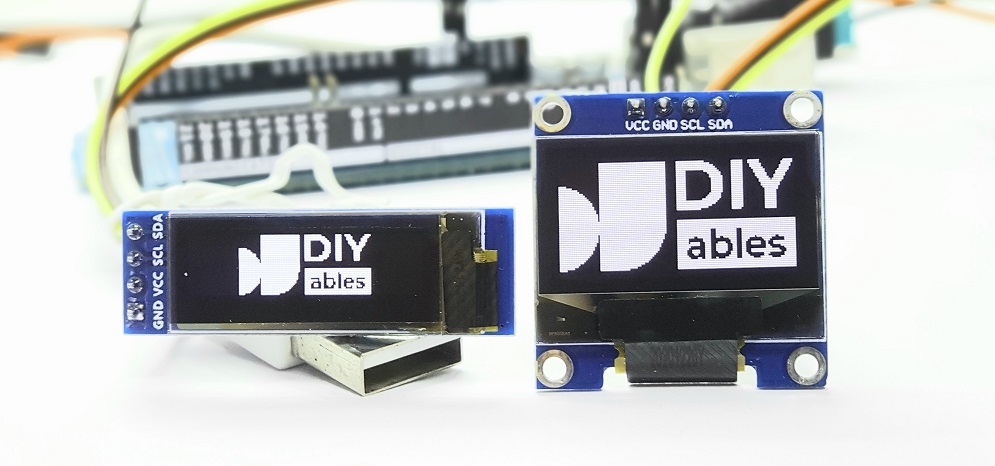
SPI est généralement plus rapide que I2C mais nécessite plus de broches Arduino. Tandis que I2C nécessite seulement deux broches et peut être partagé avec d'autres périphériques I2C. C'est un compromis entre le nombre de broches et la vitesse de communication. Le choix vous appartient. Pour les OLED avec interface I2C, il existe plusieurs types de pilotes tels que SSD1306, pilote SH1106. Ce tutoriel utilise l'affichage OLED I2C SSD1306 128x64 et 128x32.
Brochage de l'afficheur OLED I2C
- Broche GND : doit être connectée à la masse de l'Arduino
- Broche VCC : est l'alimentation électrique de l'affichage à laquelle nous connectons la broche de 5 volts de l'Arduino.
- Broche SCL : est une broche d'horloge série pour l'interface I2C.
- Broche SDA : est une broche de données série pour l'interface I2C.
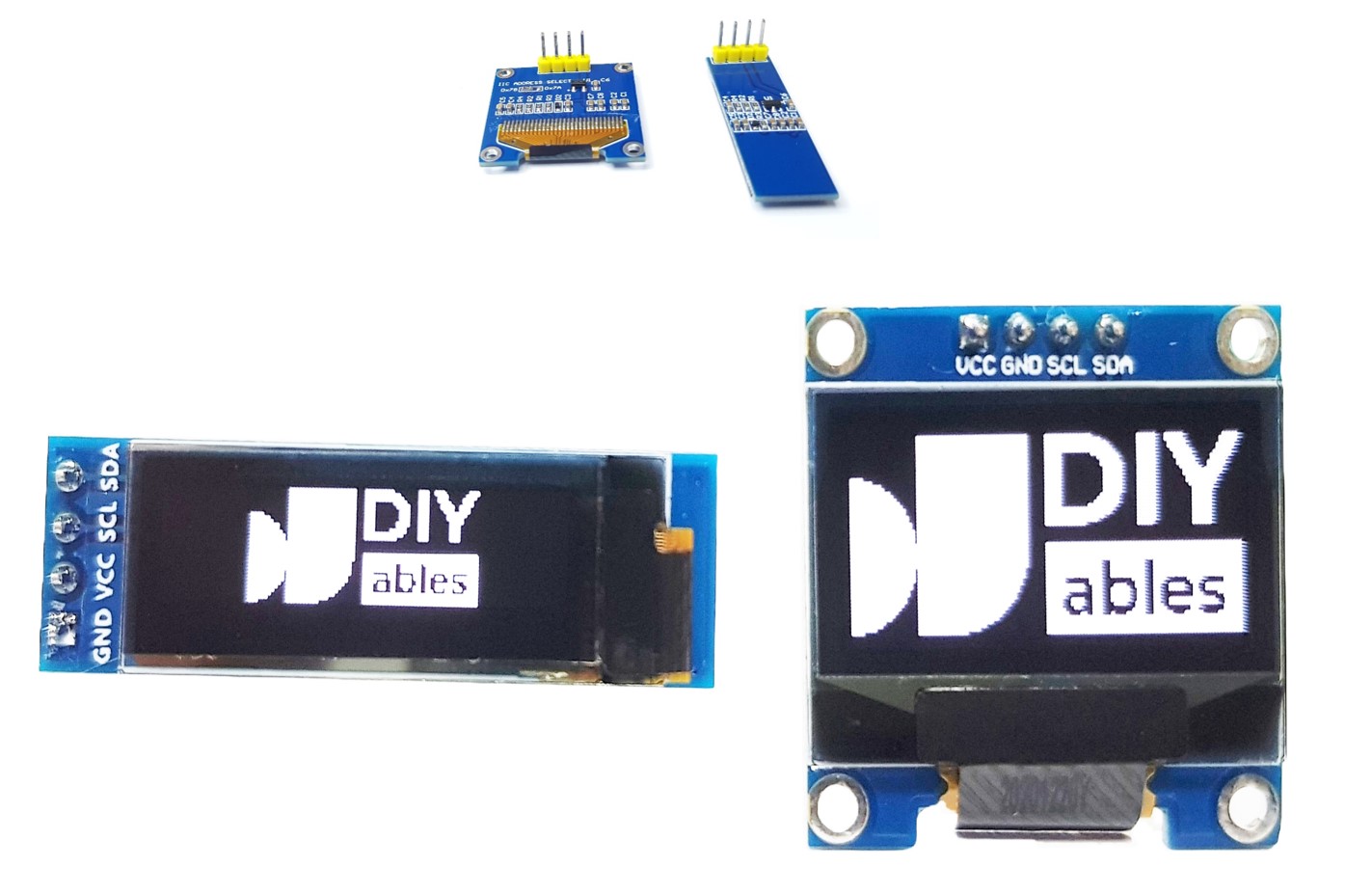
※ Note:
- L'ordre des broches du module OLED peut varier entre les fabricants et les types de modules. Utilisez TOUJOURS les étiquettes imprimées sur le module OLED. Regardez attentivement !
- Ce tutoriel utilise l'écran OLED qui utilise le pilote I2C SSD1306. Nous avons testé avec l'écran OLED de DIYables. Il fonctionne sans aucun problème.
Diagramme de câblage
- Schéma de câblage entre Arduino et OLED 128x64
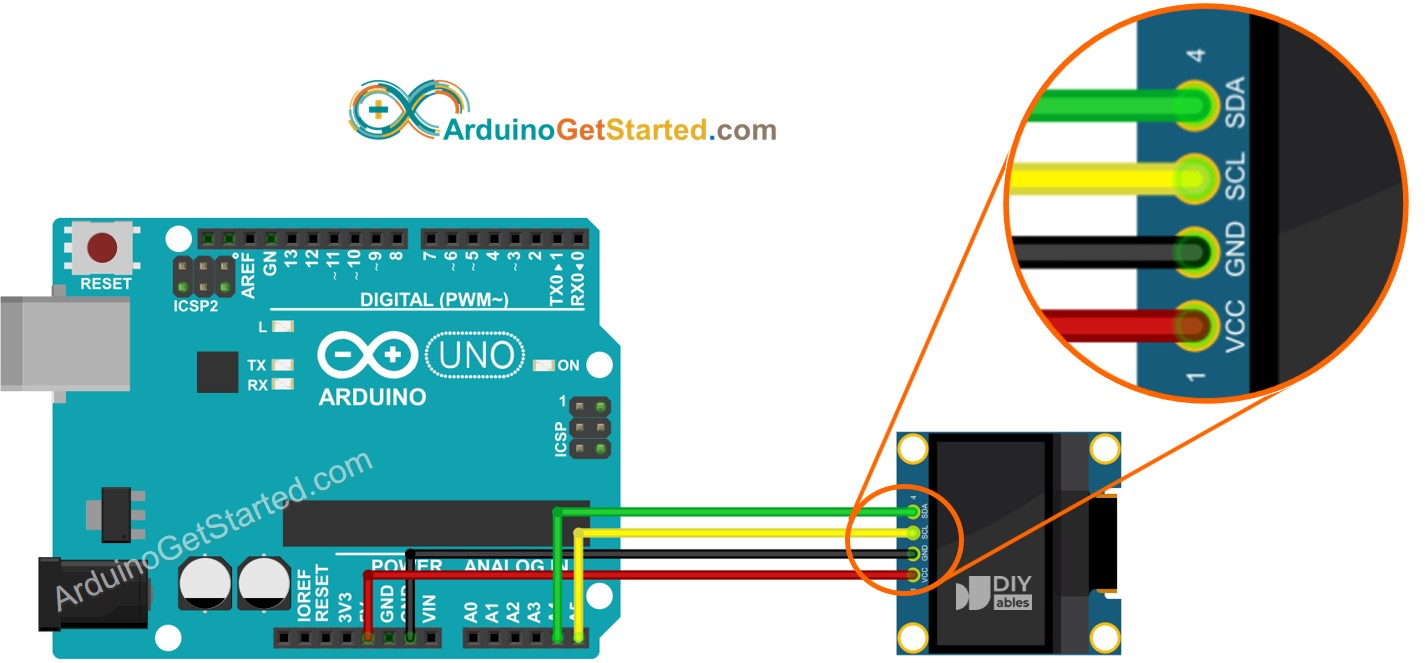
This image is created using Fritzing. Click to enlarge image
- Schéma de câblage entre Arduino et OLED 128x32
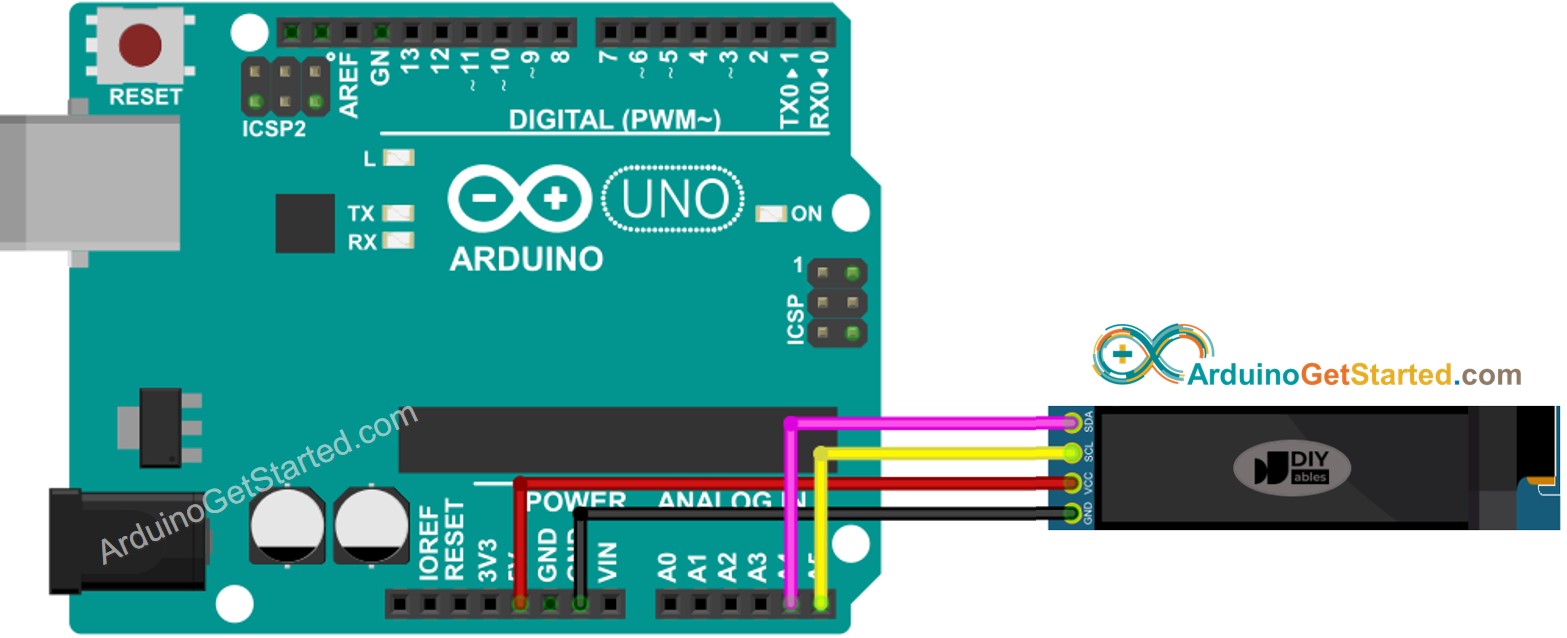
This image is created using Fritzing. Click to enlarge image
- Le véritable schéma de câblage entre l'Arduino et l'OLED 128x64.
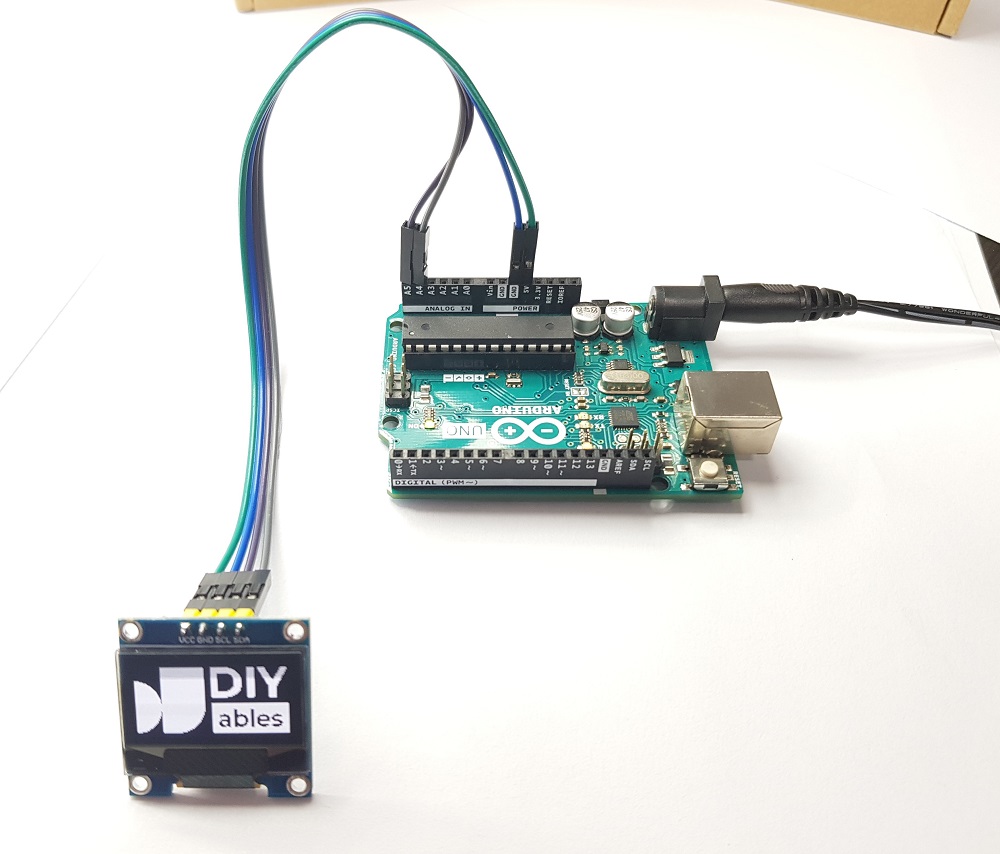
This image is created using Fritzing. Click to enlarge image
- Le véritable schéma de câblage entre Arduino et OLED 128x32
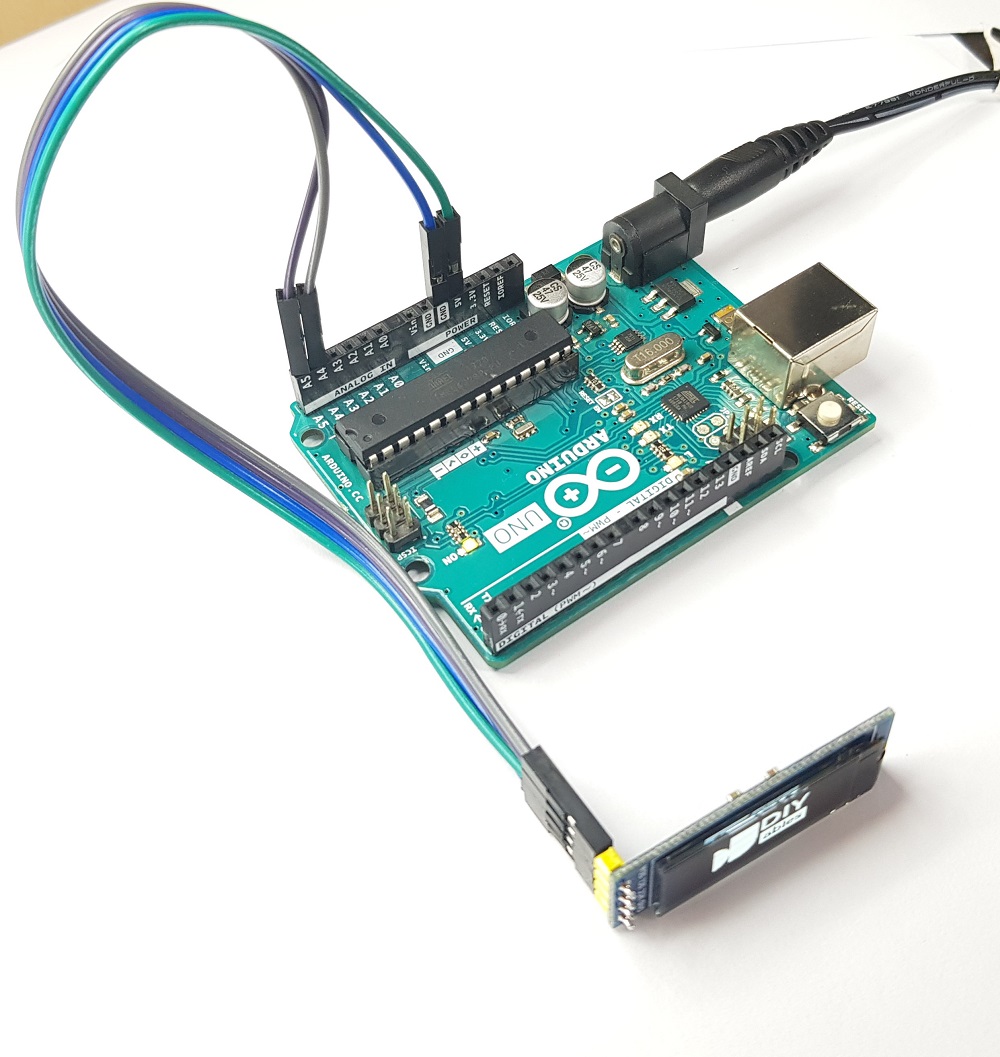
This image is created using Fritzing. Click to enlarge image
Si vous utilisez un autre Arduino que l'Uno, les broches sont différentes. Voir le tableau ci-dessous pour les autres Arduino.
OLED Module | Arduino Uno, Nano | Arduino Mega |
---|---|---|
Vin | 5V | 5V |
GND | GND | GND |
SDA | A4 | 20 |
SCL | A5 | 21 |
Comment utiliser OLED avec Arduino
Installez la bibliothèque OLED SSD1306
- Accédez à l'icône Libraries sur la barre gauche de l'IDE Arduino.
- Recherchez "SSD1306", puis trouvez la bibliothèque SSD1306 par Adafruit.
- Cliquez sur le bouton Install pour installer la bibliothèque.
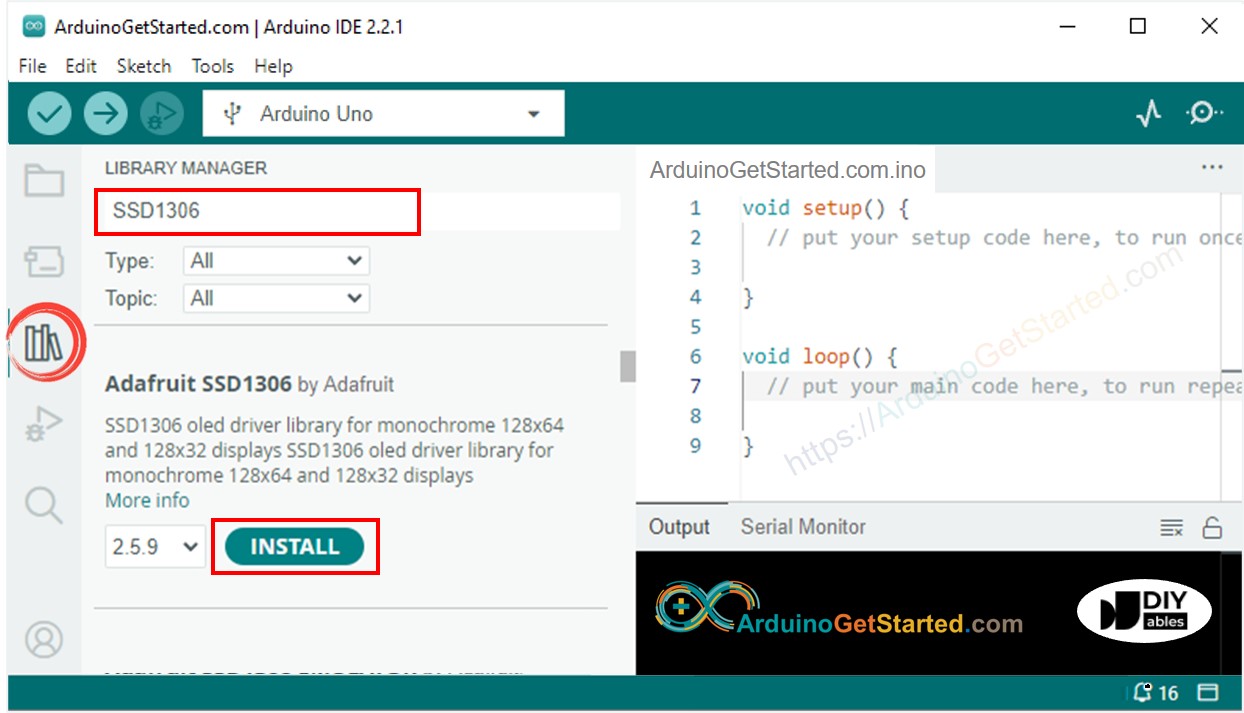
- On vous demandera d'installer d'autres dépendances de bibliothèques.
- Cliquez sur le bouton Install All pour installer toutes les dépendances de la bibliothèque.
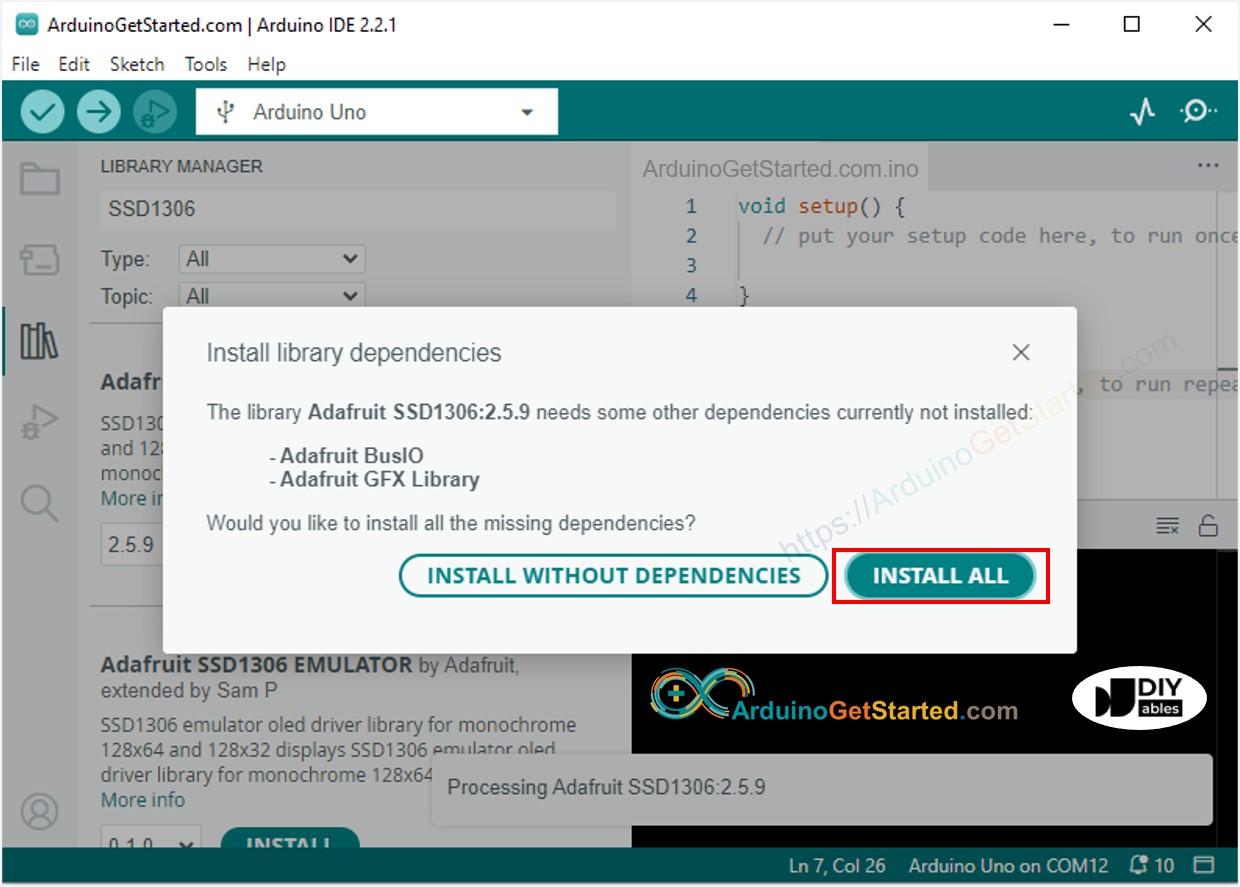
Comment programmer pour OLED
- Inclure la bibliothèque
- Définir la taille de l'écran si OLED 123x64
- Définissez la taille de l'écran si OLED 128x32
- Déclarer un objet SSD1306 OLED
- Dans la fonction setup(), initialisez l'affichage OLED.
- Et ensuite, vous pouvez afficher du texte, des images, tracer des lignes...
※ Note:
À partir de ce point, tous les codes sont fournis pour l'OLED 128x64, mais vous pouvez facilement les adapter pour un OLED 128x32 en changeant la taille de l'écran et en ajustant les coordonnées si nécessaire.
Code Arduino - Afficher du texte sur OLED
Voici quelques fonctions que vous pouvez utiliser pour afficher du texte sur l'OLED :
- oled.clearDisplay(): tous les pixels sont éteints
- oled.drawPixel(x,y, couleur): trace un pixel aux coordonnées x,y
- oled.setTextSize(n): définit la taille de la police, prend en charge les tailles de 1 à 8
- oled.setCursor(x,y): définit les coordonnées pour commencer à écrire du texte
- oled.setTextColor(BLANC): définit la couleur du texte
- oled.setTextColor(NOIR, BLANC): définit la couleur du texte, couleur de fond
- oled.println(“message”): imprime les caractères
- oled.println(nombre): imprime un nombre
- oled.println(nombre, HEX): imprime un nombre au format hexadécimal
- oled.display(): appeler cette méthode pour que les modifications prennent effet
- oled.startscrollright(start, stop): fait défiler le texte de gauche à droite
- oled.startscrollleft(start, stop): fait défiler le texte de droite à gauche
- oled.startscrolldiagright(start, stop): fait défiler le texte du coin inférieur gauche au coin supérieur droit
- oled.startscrolldiagleft(start, stop): fait défiler le texte du coin inférieur droit au coin supérieur gauche
- oled.stopscroll(): arrête le défilement
Comment aligner verticalement et horizontalement du texte/numéro sur un OLED
Code Arduino - Dessin sur OLED
Code Arduino – Afficher une image
Pour dessiner une image sur un OLED, nous devons d'abord convertir l'image (quel que soit le format) en tableau de bitmap. La conversion peut être effectuée en utilisant cet outil en ligne. Veuillez voir comment convertir une image en tableau de bitmap sur l'image ci-dessous. J'ai converti l'icône Arduino en tableau de bitmap.
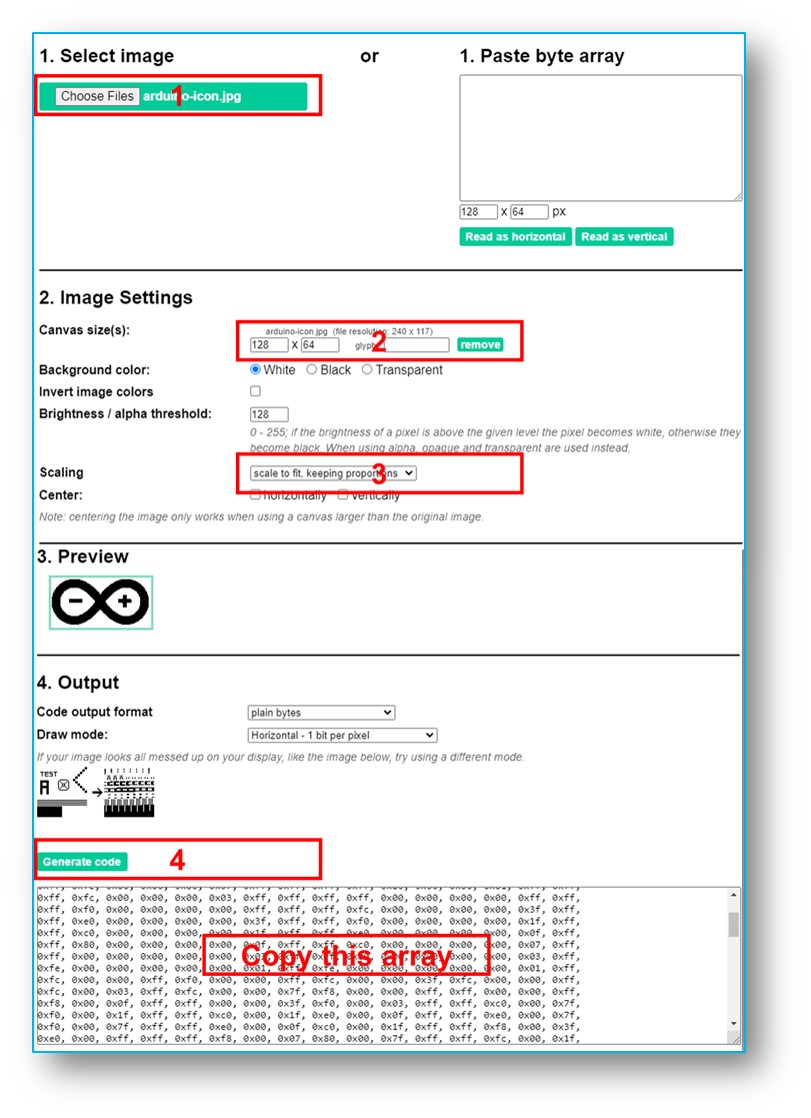
Après la conversion, copiez le code du tableau et mettez à jour le code du tableau dans le tableau ArduinoIcon dans le code ci-dessous
※ Note:
- La taille de l'image doit être inférieure ou égale à celle de l'écran.
- Si vous souhaitez adapter le code ci-dessus pour un OLED 128x32, vous devez redimensionner l'image et modifier la largeur/hauteur dans la fonction oled.drawBitmap();.
Dépannage des OLED
Si l'OLED n'affiche rien, veuillez suivre la liste de vérification suivante :
- Assurez-vous que votre câblage est correct.
- Assurez-vous que votre OLED I2C utilise le pilote SSD1306.
- Vérifiez l'adresse I2C de l'OLED en exécutant le code ci-dessous de scanner d'adresse I2C sur Arduino.
Le résultat sur le moniteur série :