Arduino - Horloge OLED
Dans ce tutoriel, nous allons apprendre à fabriquer une horloge OLED en :
- Lecture de l'heure (heure, minute, seconde) à partir du module RTC DS3231 et affichage sur un OLED
- Lecture de l'heure (heure, minute, seconde) à partir du module RTC DS1307 et affichage sur un OLED
Vous pouvez choisir l'un des deux modules RTC : DS3231 et DS1307. Voir DS3231 vs DS1307
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Divulgation : Certains des liens fournis dans cette section sont des liens affiliés Amazon. Nous pouvons recevoir une commission pour tout achat effectué via ces liens, sans coût supplémentaire pour vous. Nous vous remercions de votre soutien.
À propos des modules OLED, DS3231 et DS1307 RTC
Si vous ne connaissez pas les OLED, DS3231 et DS1307 (brochage, fonctionnement, programmation...), renseignez-vous sur ces sujets dans les tutoriels suivants :
Installez les bibliothèques OLED et RTC
- Naviguez vers l'icône Libraries sur la barre gauche de l'IDE Arduino.
- Recherchez "SSD1306", puis trouvez la bibliothèque SSD1306 par Adafruit
- Cliquez sur le bouton Install pour installer la bibliothèque.
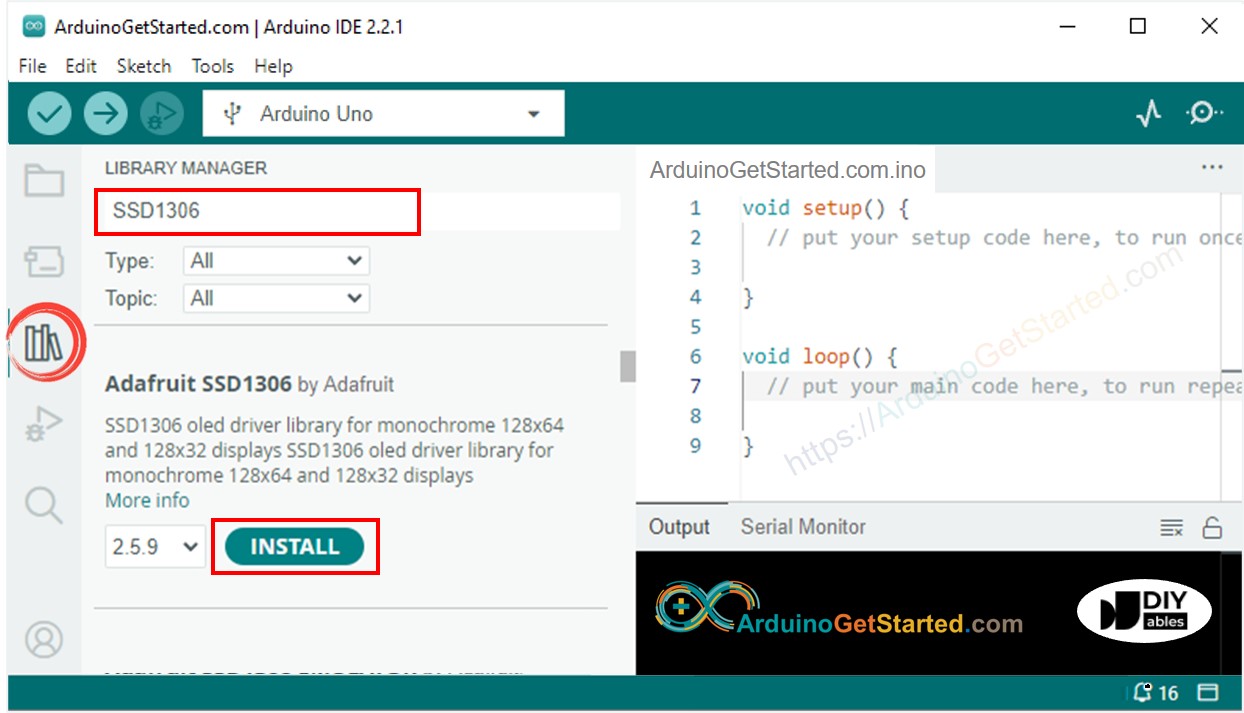
- On vous demandera d'installer d'autres dépendances de bibliothèques.
- Cliquez sur le bouton Install All pour installer toutes les dépendances de bibliothèque.
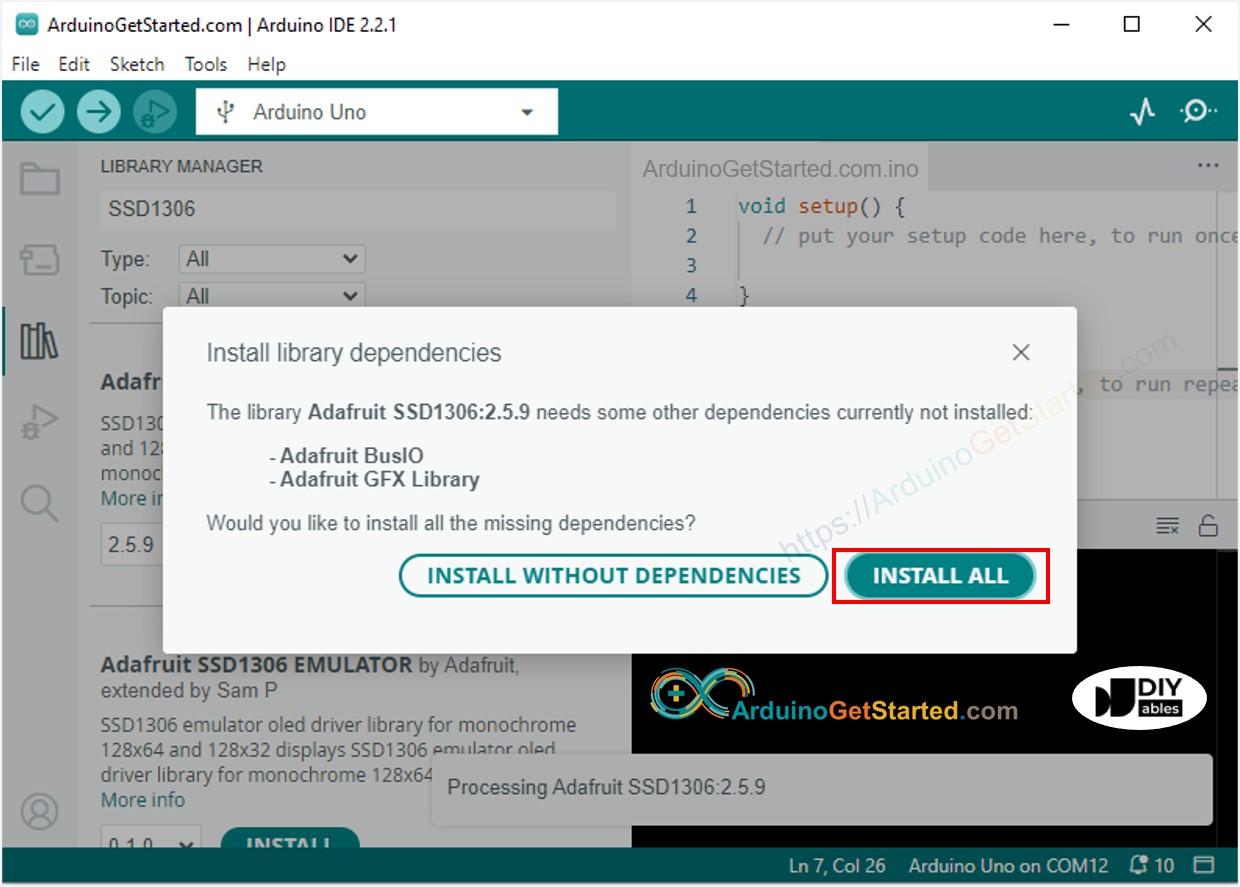
- Recherchez « RTClib », puis trouvez la bibliothèque RTC par Adafruit. Cette bibliothèque fonctionne avec les DS3231 et DS1307.
- Cliquez sur le bouton Install pour installer la bibliothèque RTC.
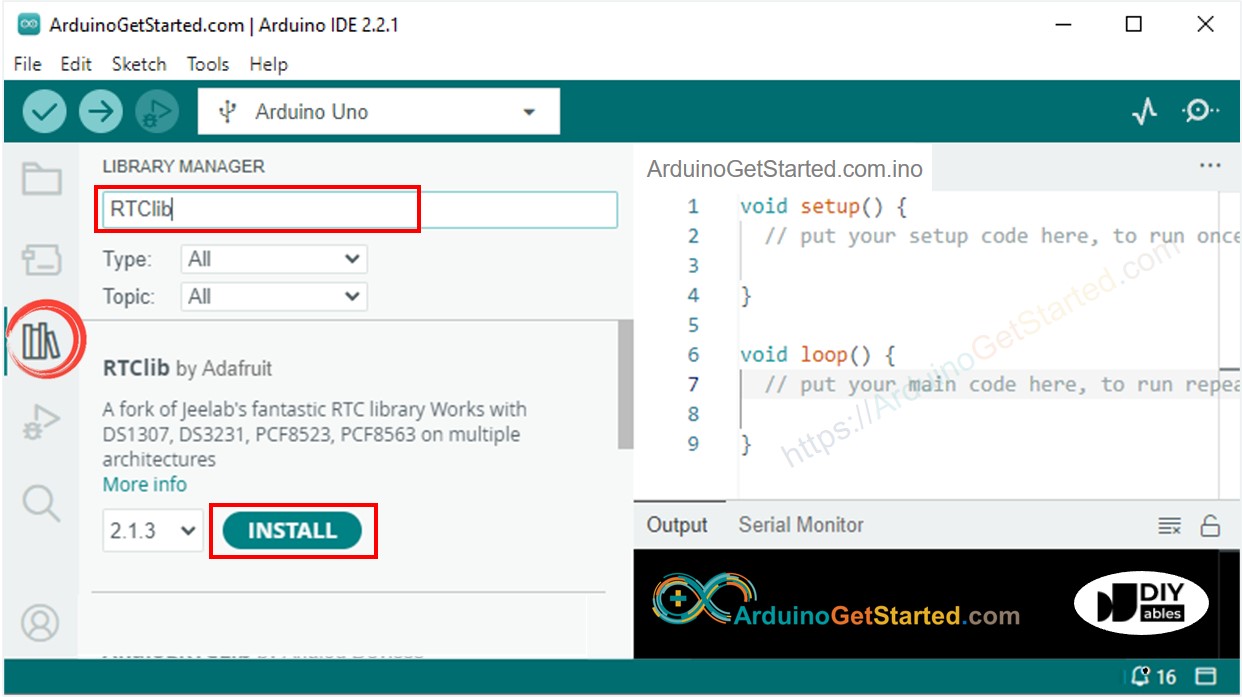
- Il se peut qu'on vous demande d'installer d'autres dépendances de bibliothèques
- Cliquez sur le bouton Install All pour installer toutes les dépendances des bibliothèques.
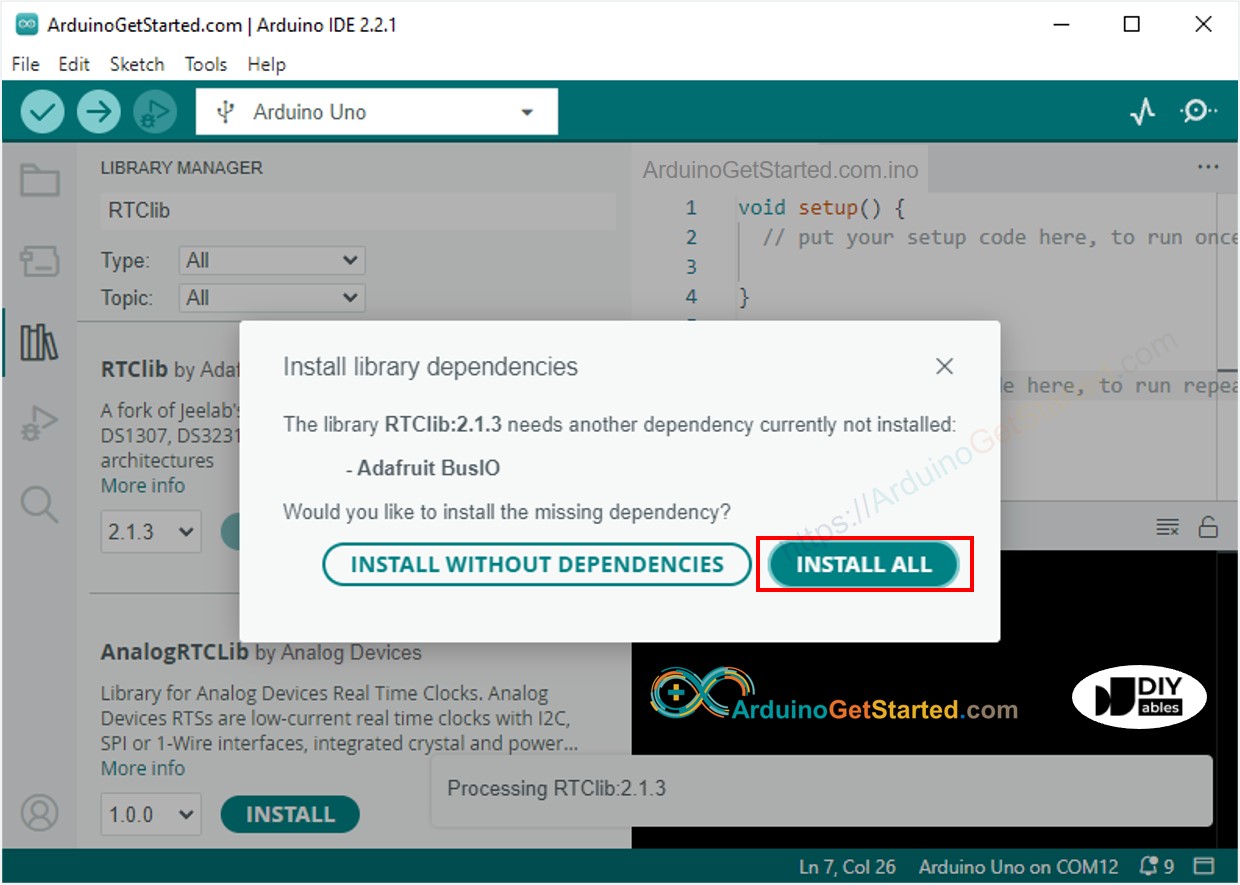
Lire l'heure depuis le module RTC DS3231 et l'afficher sur OLED
Schéma de câblage
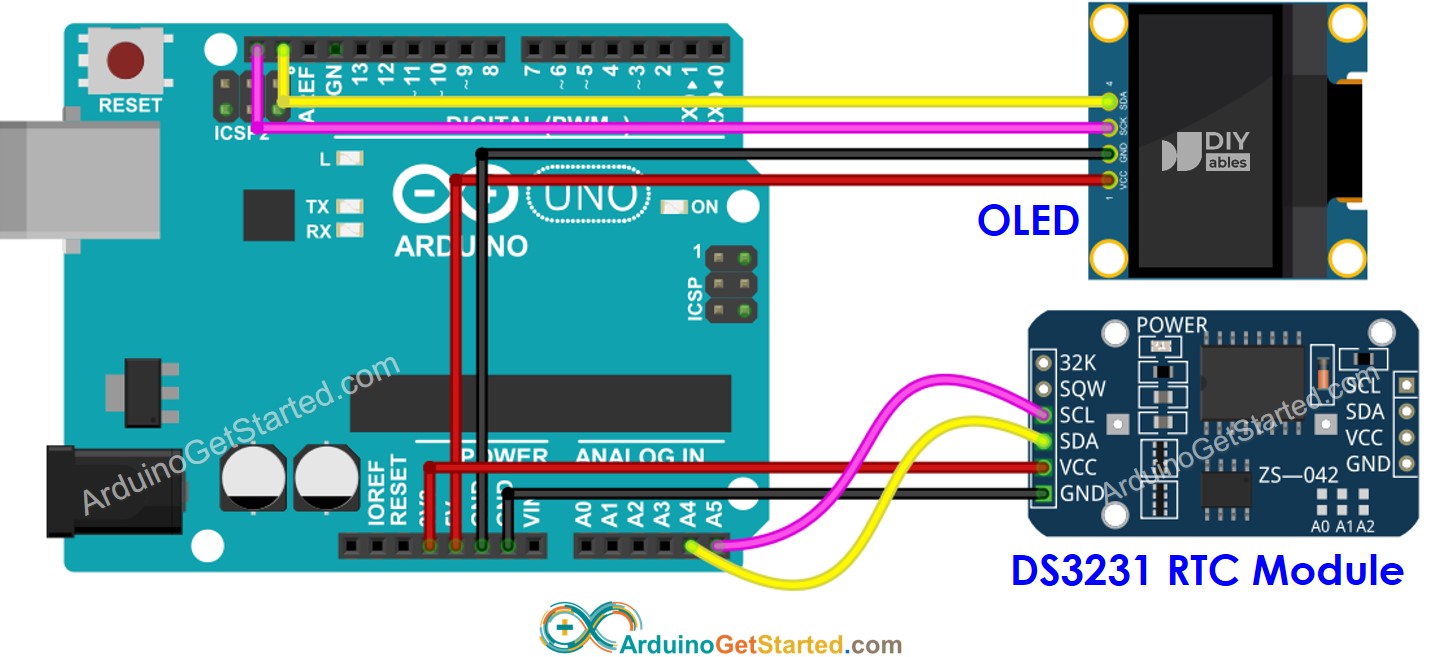
This image is created using Fritzing. Click to enlarge image
Code Arduino - DS3231 et OLED
/*
* Ce code Arduino a été développé par newbiely.fr
* Ce code Arduino est mis à disposition du public sans aucune restriction.
* Pour des instructions complètes et des schémas de câblage, veuillez visiter:
* https://newbiely.fr/tutorials/arduino/arduino-oled-clock
*/
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <RTClib.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // // create SSD1306 display object connected to I2C
RTC_DS3231 rtc;
String time;
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(1); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
// SETUP RTC MODULE
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
Serial.flush();
while (true);
}
// automatically sets the RTC to the date & time on PC this sketch was compiled
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
time.reserve(10); // to avoid fragmenting memory when using String
}
void loop() {
DateTime now = rtc.now();
time = "";
time += now.hour();
time += ':';
time += now.minute();
time += ':';
time += now.second();
oledDisplayCenter(time);
}
void oledDisplayCenter(String text) {
int16_t x1;
int16_t y1;
uint16_t width;
uint16_t height;
oled.getTextBounds(text, 0, 0, &x1, &y1, &width, &height);
// display on horizontal and vertical center
oled.clearDisplay(); // clear display
oled.setCursor((SCREEN_WIDTH - width) / 2, (SCREEN_HEIGHT - height) / 2);
oled.println(text); // text to display
oled.display();
}
Étapes rapides
- Copiez le code ci-dessus et ouvrez-le avec Arduino IDE
- Cliquez sur le bouton Upload dans Arduino IDE pour téléverser le code vers l'Arduino
- Voyez le résultat sur l'OLED
Lecture de l'heure à partir du module RTC DS1307 et affichage sur OLED.
Schéma de câblage
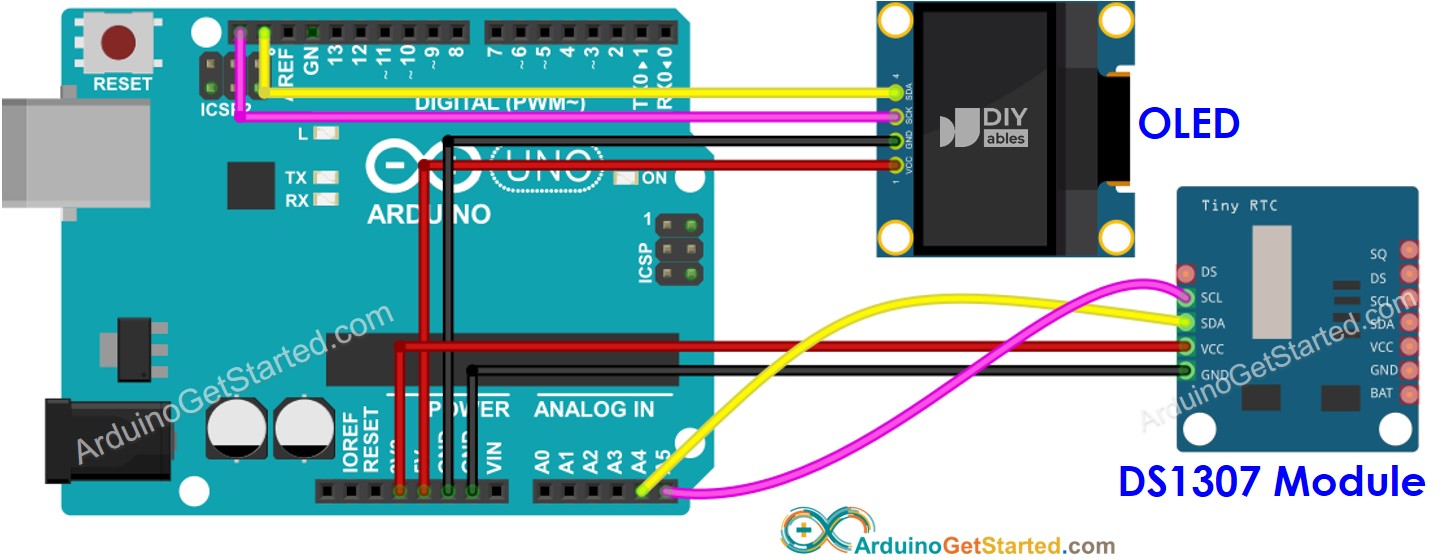
This image is created using Fritzing. Click to enlarge image
Code Arduino - DS1307 et OLED
/*
* Ce code Arduino a été développé par newbiely.fr
* Ce code Arduino est mis à disposition du public sans aucune restriction.
* Pour des instructions complètes et des schémas de câblage, veuillez visiter:
* https://newbiely.fr/tutorials/arduino/arduino-oled-clock
*/
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <RTClib.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // // create SSD1306 display object connected to I2C
RTC_DS1307 rtc;
String time;
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(1); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
// SETUP RTC MODULE
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
Serial.flush();
while (true);
}
// automatically sets the RTC to the date & time on PC this sketch was compiled
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
time.reserve(10); // to avoid fragmenting memory when using String
}
void loop() {
DateTime now = rtc.now();
time = "";
time += now.hour();
time += ':';
time += now.minute();
time += ':';
time += now.second();
oledDisplayCenter(time);
}
void oledDisplayCenter(String text) {
int16_t x1;
int16_t y1;
uint16_t width;
uint16_t height;
oled.getTextBounds(text, 0, 0, &x1, &y1, &width, &height);
// display on horizontal and vertical center
oled.clearDisplay(); // clear display
oled.setCursor((SCREEN_WIDTH - width) / 2, (SCREEN_HEIGHT - height) / 2);
oled.println(text); // text to display
oled.display();
}
Étapes rapides
- Copiez le code ci-dessus et ouvrez-le avec Arduino IDE
- Cliquez sur le bouton Upload dans l'IDE Arduino pour téléverser le code vers Arduino
- Voir le résultat sur l'OLED