Horloge ESP32 - OLED
Dans ce tutoriel, nous vous guiderons à travers le processus de création d'une horloge OLED en utilisant un ESP32 en couvrant les étapes suivantes :
- Lecture de l'heure actuelle (heure, minute, seconde) à partir d'un module RTC DS3231 et affichage sur un OLED.
- Lecture de l'heure actuelle (heure, minute, seconde) à partir d'un module RTC DS1307 et affichage sur un OLED.
Vous avez la flexibilité de choisir entre deux modules RTC : DS3231 et DS1307. Pour vous aider à prendre une décision éclairée, vous pouvez consulter la comparaison détaillée dans DS3231 vs DS1307.
Ce tutoriel fournira un guide complet pour implémenter une horloge OLED, présentant l'intégration de l'ESP32 avec le module RTC DS3231 ou DS1307 pour afficher des informations horaires précises sur un écran OLED.
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos des modules OLED, DS3231 et DS1307 RTC
Non familiarisé avec les OLED, DS3231 et DS1307, y compris leurs brochages, fonctionnalités et programmation ? Explorez des tutoriels complets sur ces sujets ci-dessous :
Installez les bibliothèques OLED et RTC
- Cliquez sur l'icône Libraries dans la barre de gauche de l'IDE Arduino.
- Recherchez "SSD1306", puis trouvez la bibliothèque SSD1306 par Adafruit.
- Cliquez sur le bouton Install pour installer la bibliothèque.
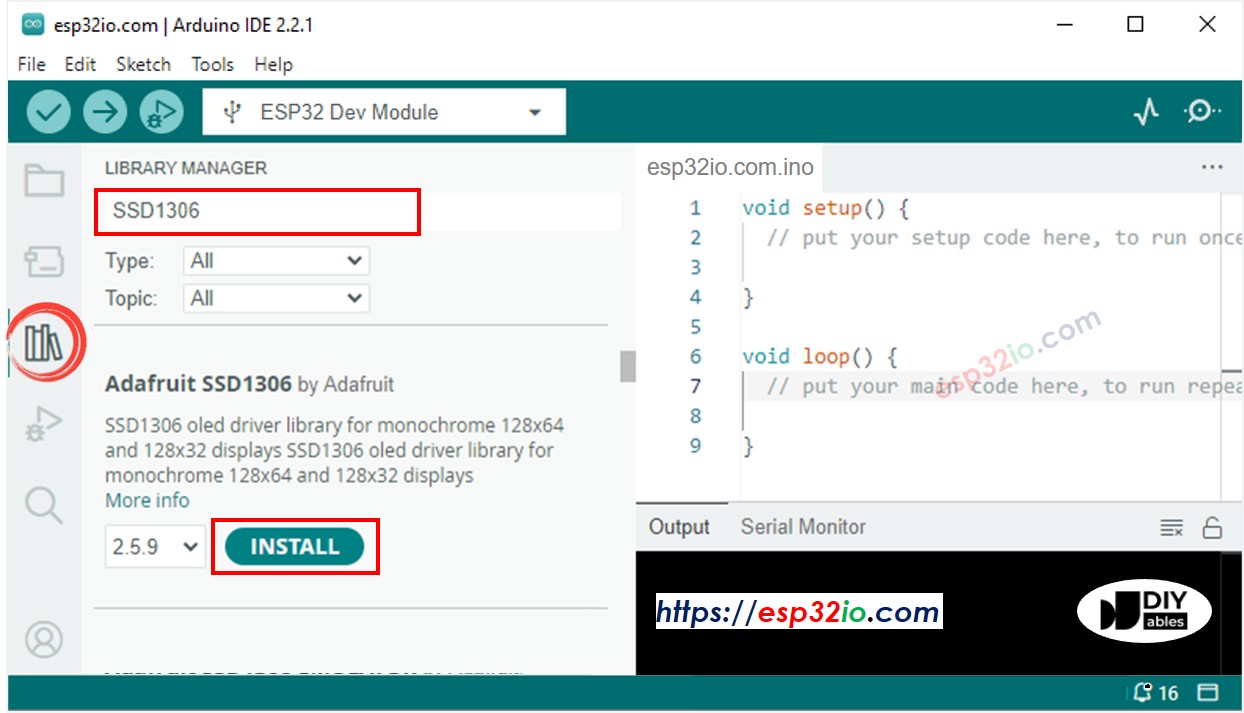
- On vous demandera d'installer d'autres dépendances de bibliothèques.
- Cliquez sur le bouton Install All pour installer toutes les dépendances des bibliothèques.
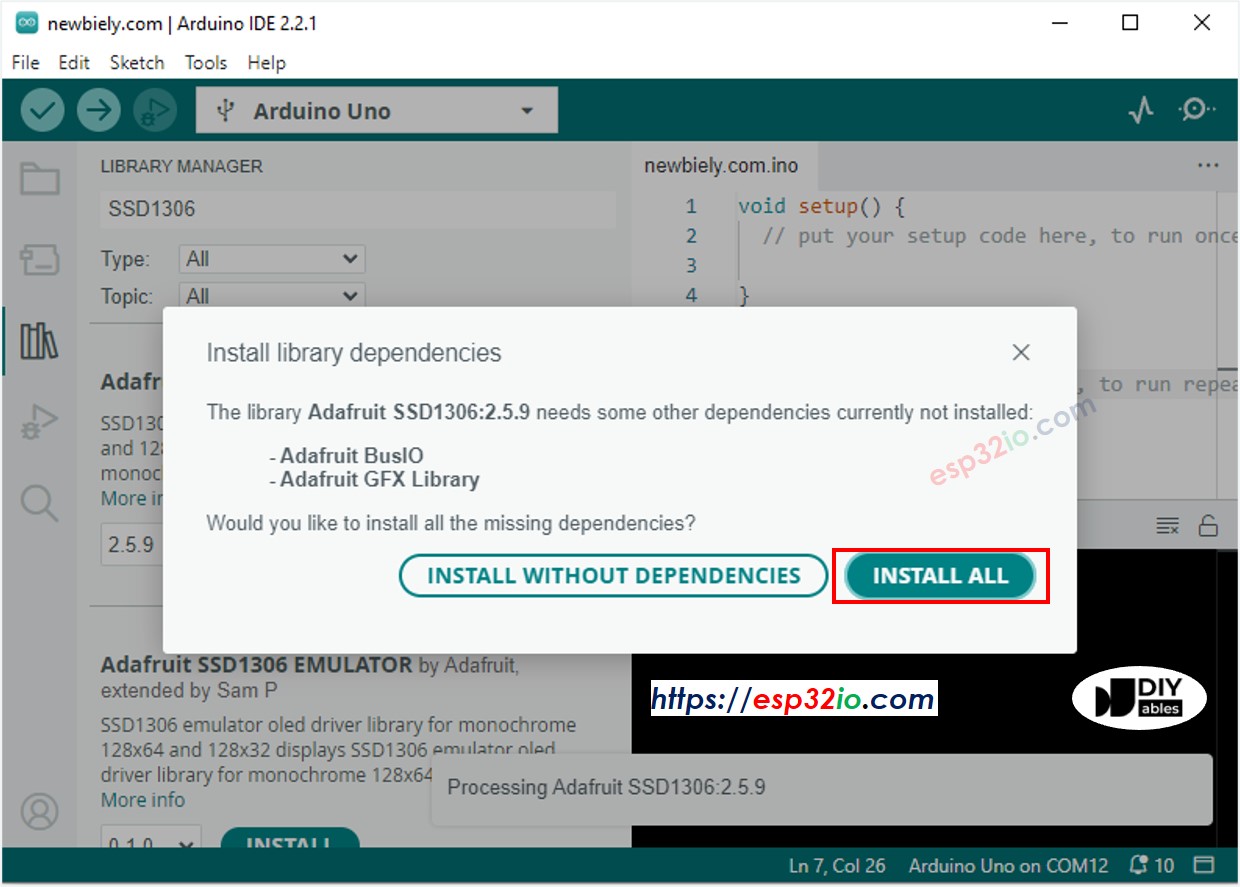
- Recherchez « RTClib », puis trouvez la bibliothèque RTC d'Adafruit.
- Cliquez sur le bouton Install pour installer la bibliothèque RTC.
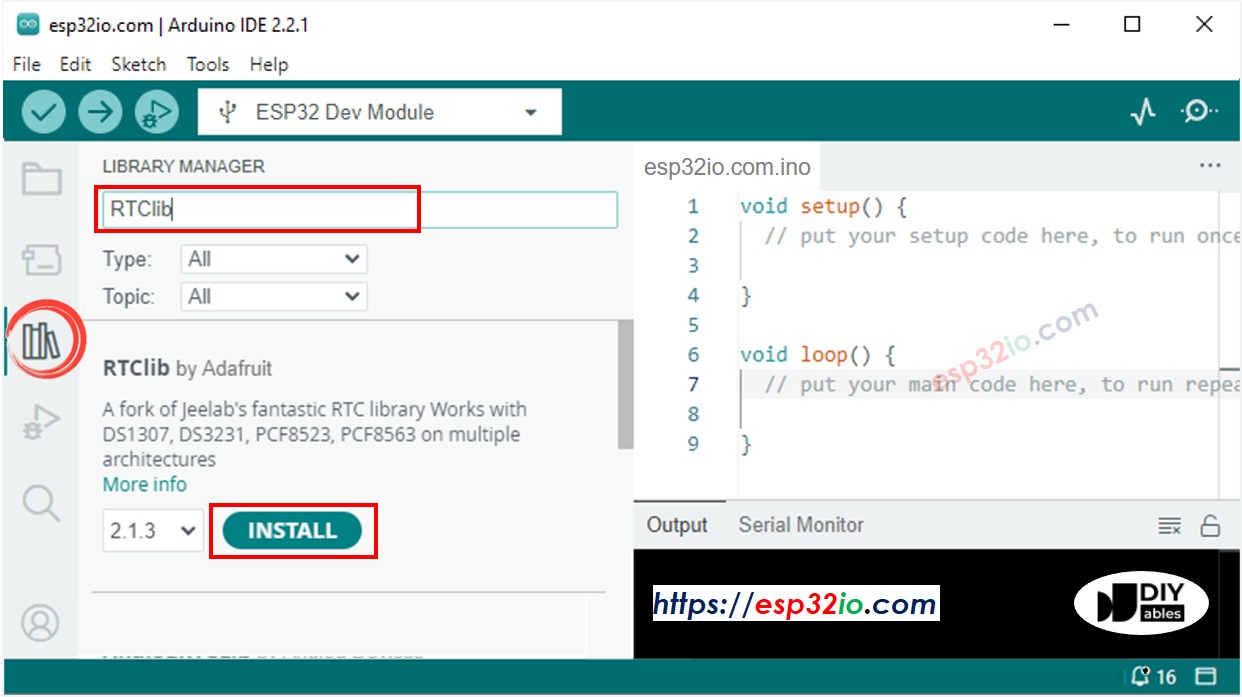
- Il se peut qu'on vous demande d'installer les dépendances de la bibliothèque.
- Installez toutes les dépendances de la bibliothèque en cliquant sur le bouton Install All.
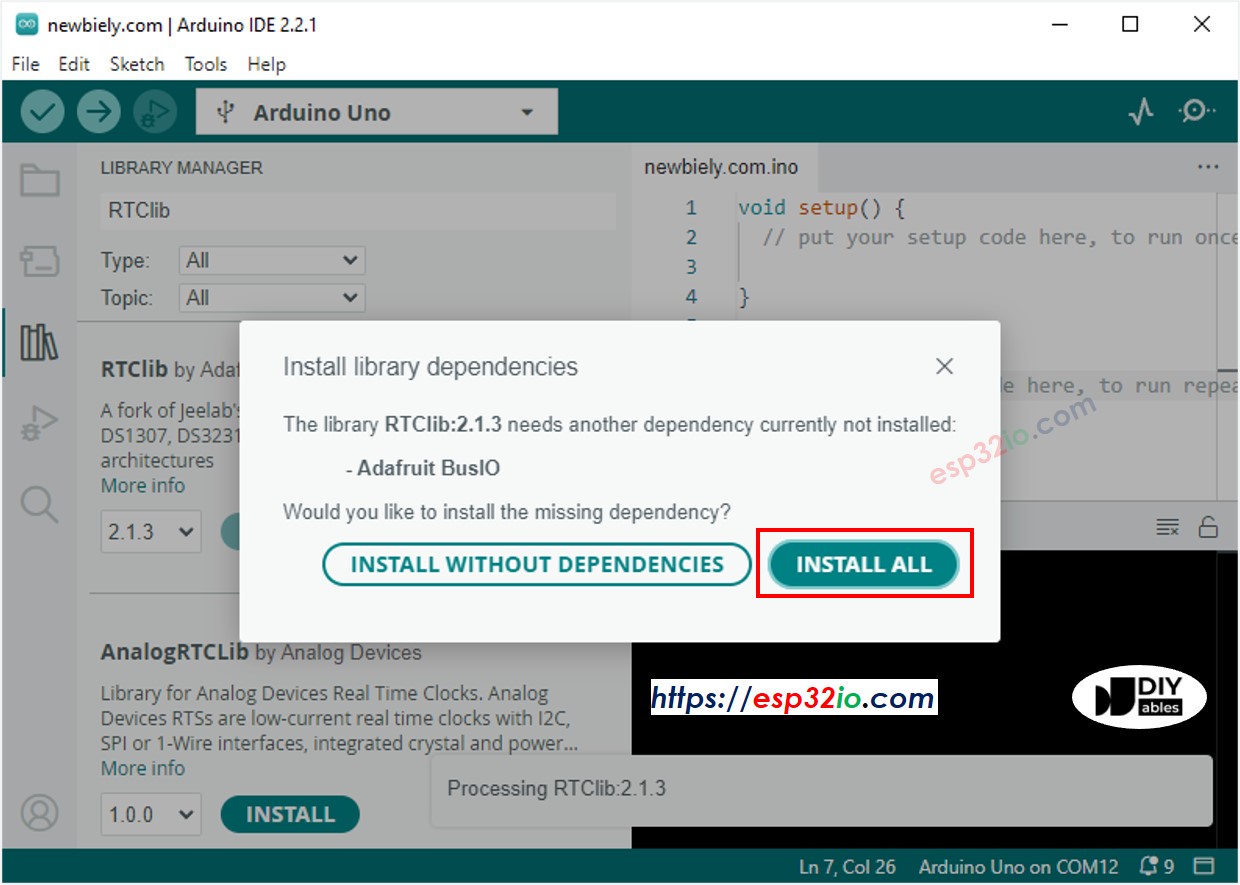
Lire l'heure depuis le module RTC DS3231 et l'afficher sur un OLED
Schéma de câblage
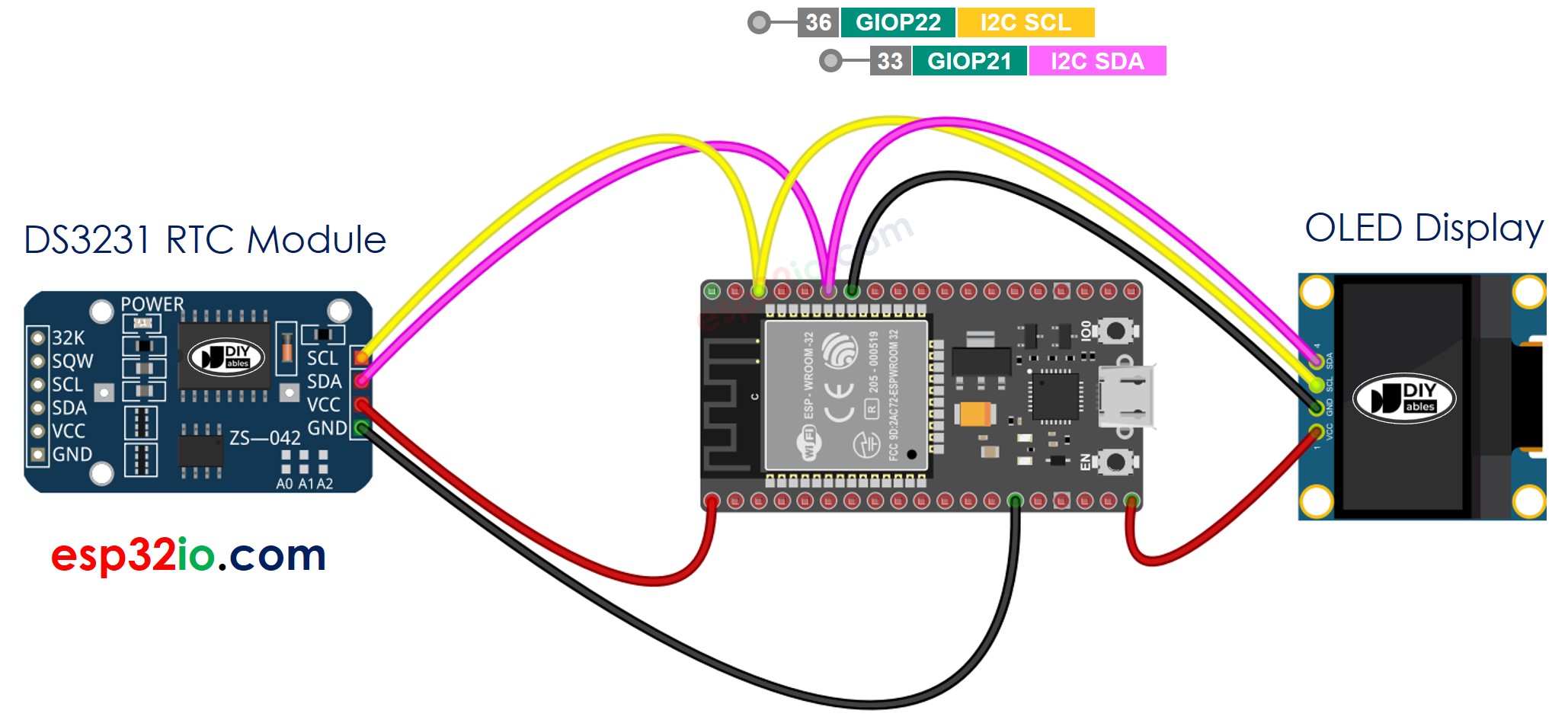
This image is created using Fritzing. Click to enlarge image
Si vous ne savez pas comment alimenter l'ESP32 et d'autres composants, vous pouvez trouver des conseils dans le tutoriel suivant : Comment alimenter l'ESP32.
Code ESP32 - DS3231 et OLED
Étapes rapides
- Si c'est la première fois que vous utilisez ESP32, consultez Installation du logiciel ESP32..
- Réalisez le câblage comme sur l'image ci-dessus.
- Connectez la carte ESP32 à votre PC via un câble micro USB.
- Ouvrez Arduino IDE sur votre PC.
- Sélectionnez la bonne carte ESP32 (par exemple, ESP32 Dev Module) et le port COM.
- Copiez le code ci-dessus et ouvrez-le avec Arduino IDE.
- Cliquez sur le bouton Upload sur Arduino IDE pour télécharger le code sur ESP32.
- Vérifiez le résultat sur l'OLED.
Lire l'heure depuis le module RTC DS1307 et l'afficher sur un OLED
Schéma de câblage
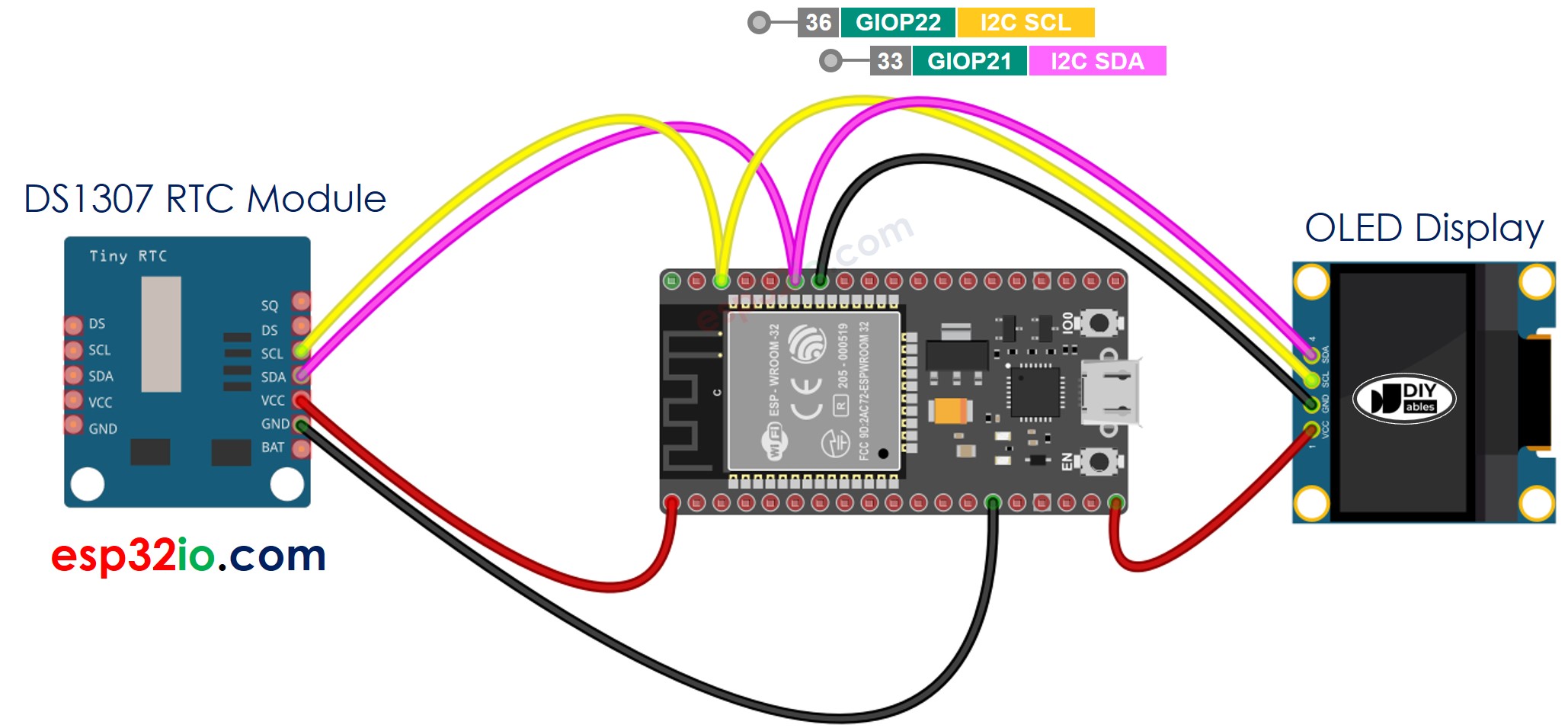
This image is created using Fritzing. Click to enlarge image
Code ESP32 - DS1307 et OLED
Étapes rapides
- Si c'est la première fois que vous utilisez un ESP32, consultez Installation du logiciel ESP32..
- Faites le câblage comme sur l'image ci-dessus.
- Connectez la carte ESP32 à votre PC via un câble micro USB
- Ouvrez Arduino IDE sur votre PC.
- Sélectionnez la bonne carte ESP32 (par exemple, Module de développement ESP32) et le port COM.
- Copiez le code ci-dessus et ouvrez-le avec Arduino IDE
- Cliquez sur le bouton Upload sur Arduino IDE pour téléverser le code sur l'ESP32
- Voir le résultat sur l'OLED