ESP32 - OLED
Ce tutoriel vous apprend à utiliser l'ESP32 pour afficher des données sur un OLED. En détail, nous apprendrons comment imprimer des caractères et des nombres, dessiner des lignes et des formes, et imprimer des images sur l'affichage OLED.
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos de l'écran OLED
Il existe plusieurs types différents d'écrans OLED compatibles avec l'ESP32. Ils se distinguent par leur interface de communication (I2C, SPI), leurs couleurs (blanc, bleu, bicolore...) et leurs tailles (128x64, 128x32...).
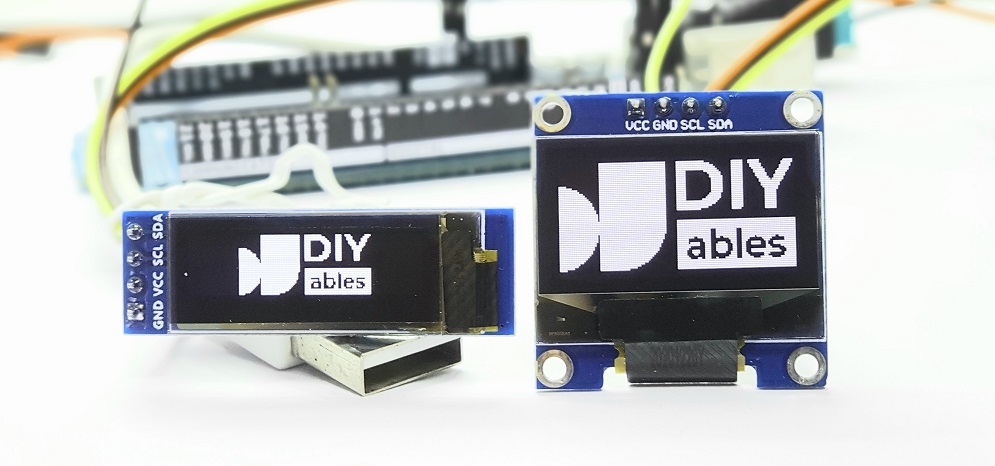
Ce tutoriel utilise un écran OLED I2C SSD1306 de 128x64.
Brochage de l'affichage OLED I2C
L'affichage OLED I2C comprend 4 broches :
- Broche GND : doit être connectée à la masse de l'ESP32.
- Broche VCC : doit être connectée à la broche 5 volts de l'ESP32.
- Broche SCL : est une broche d'horloge I2C.
- Broche SDA : est une broche de données I2C.
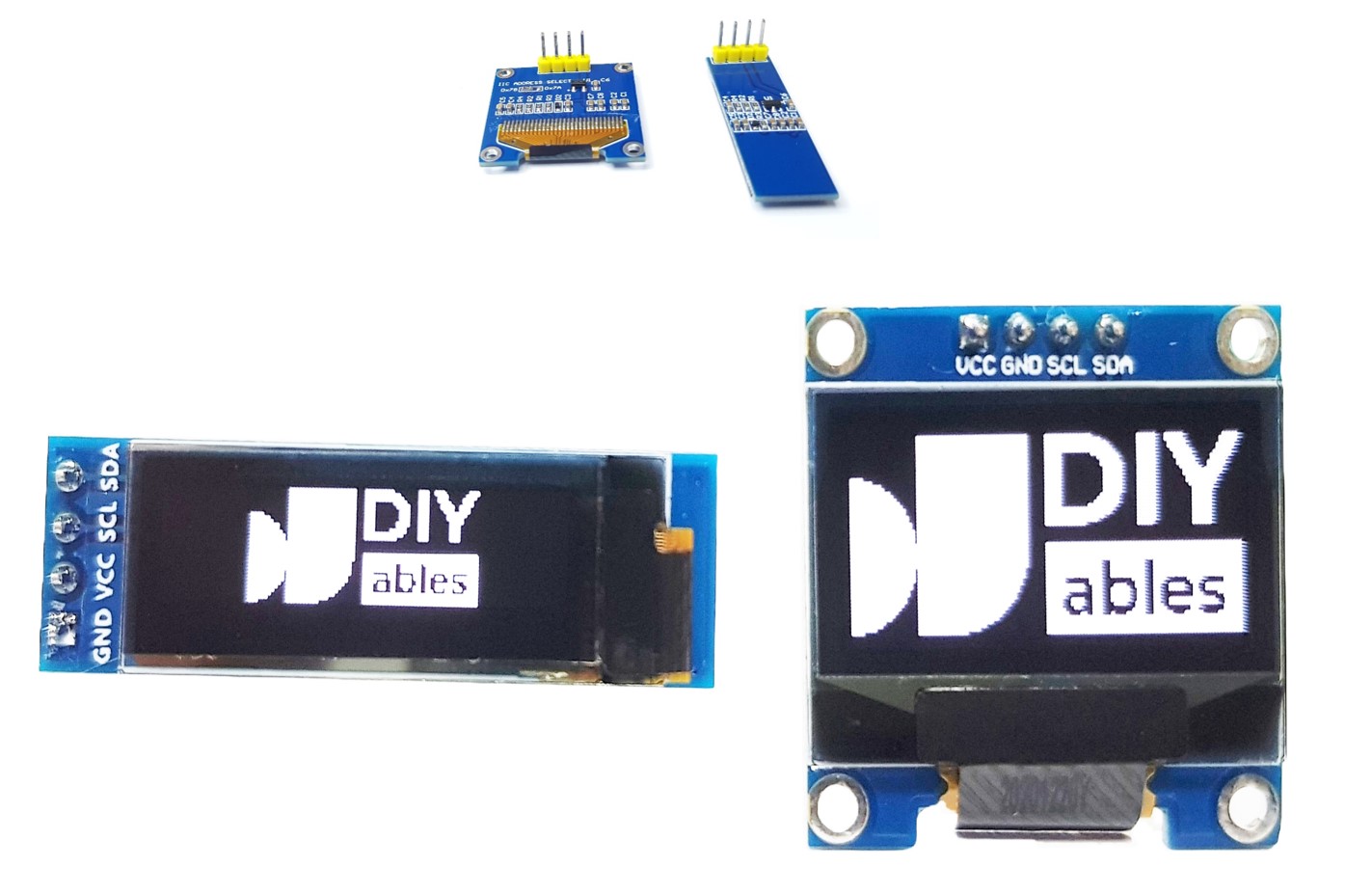
Diagramme de câblage
- Le schéma de câblage avec alimentation par câble USB
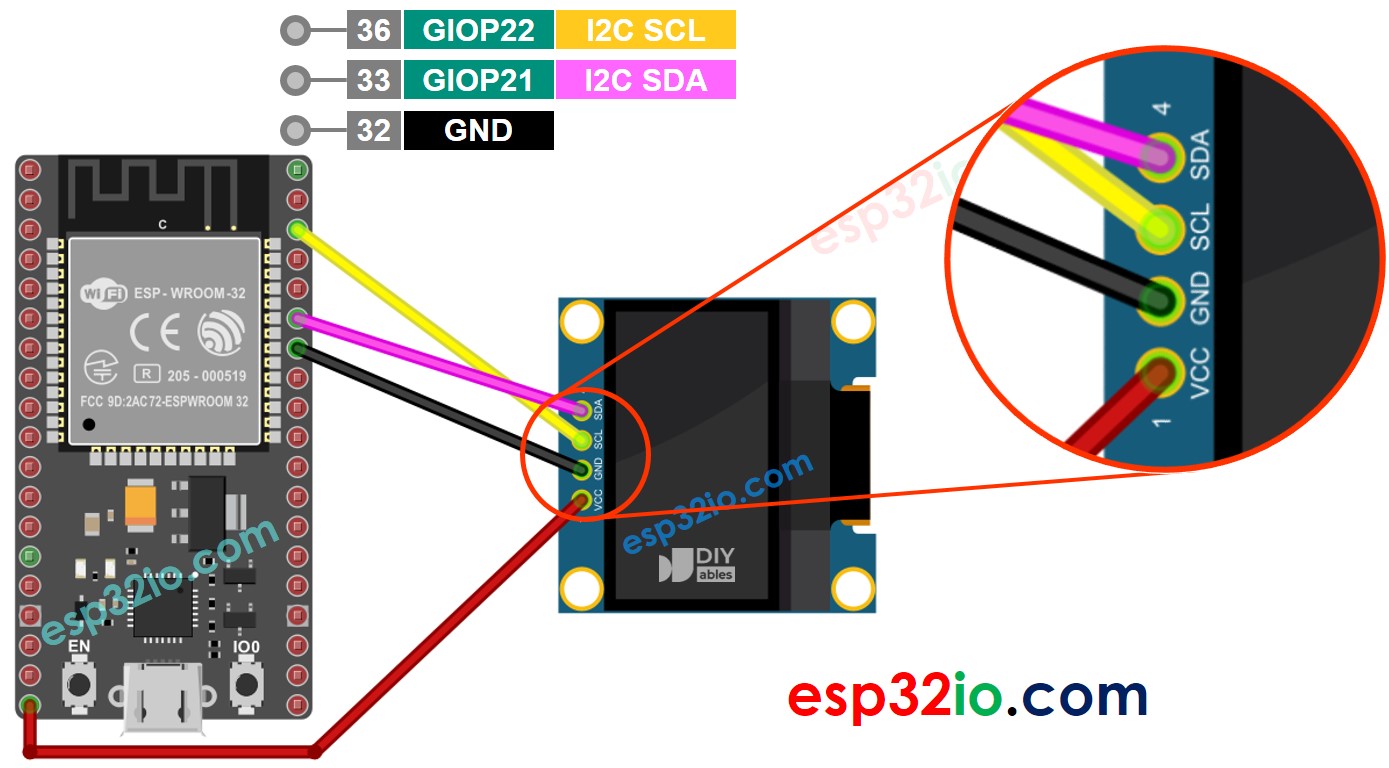
This image is created using Fritzing. Click to enlarge image
Si vous ne savez pas comment alimenter l'ESP32 et d'autres composants, vous pouvez trouver des conseils dans le tutoriel suivant : Comment alimenter l'ESP32.
- Le schéma de câblage avec alimentation par adaptateur 5v
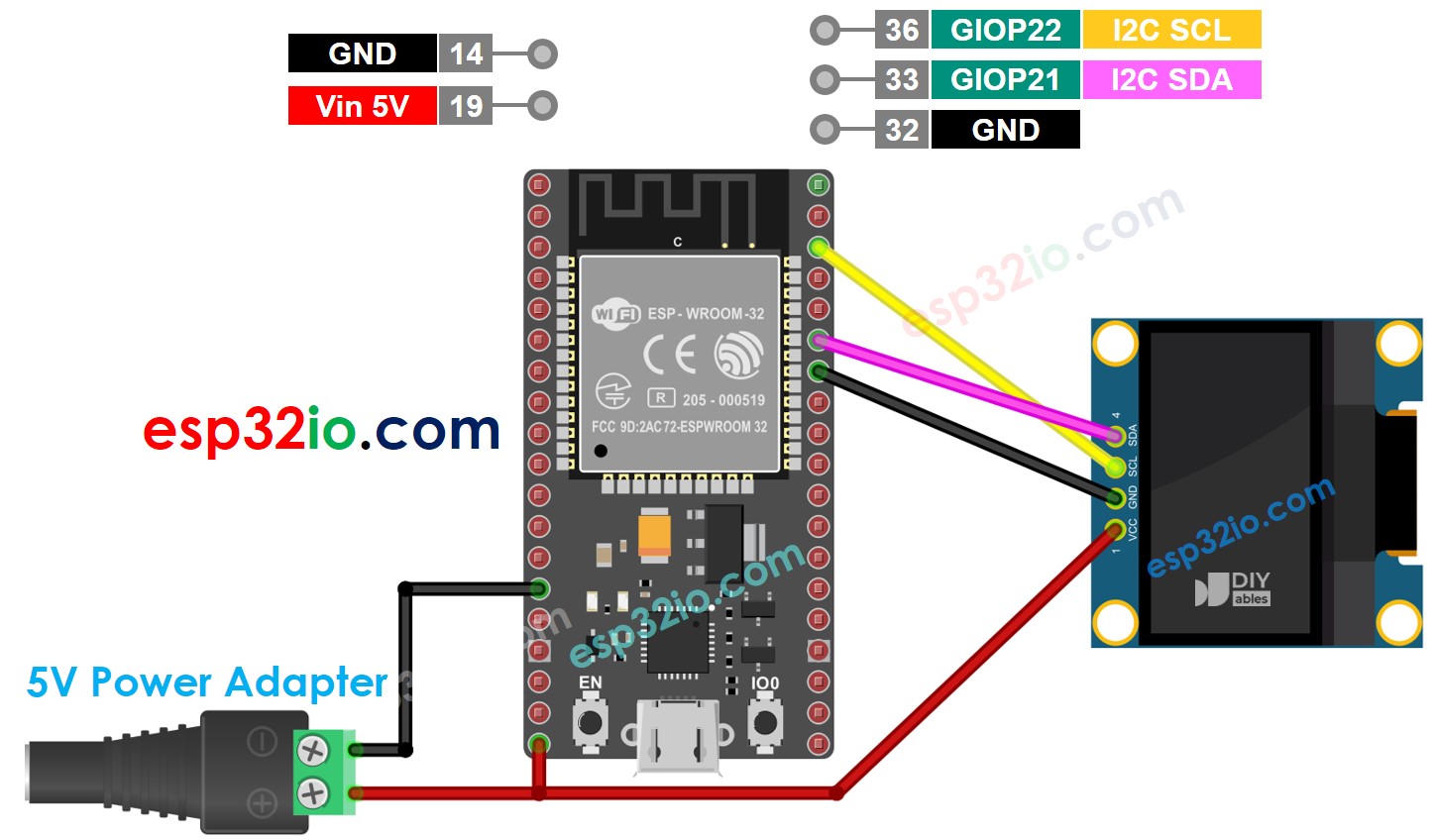
This image is created using Fritzing. Click to enlarge image
※ Note:
L'ordre des broches du module OLED peut varier d'un fabricant à l'autre. Utilisez TOUJOURS les étiquettes imprimées sur le module OLED. Regardez de près !
Comment utiliser un OLED avec l'ESP32
Installez la bibliothèque OLED SSD1306
- Cliquez sur l'icône Libraries sur la barre gauche de l'IDE Arduino.
- Tapez SSD1306 dans la boîte de recherche, puis recherchez la bibliothèque SSD1306 par Adafruit.
- Installez la bibliothèque en cliquant sur le bouton Install.
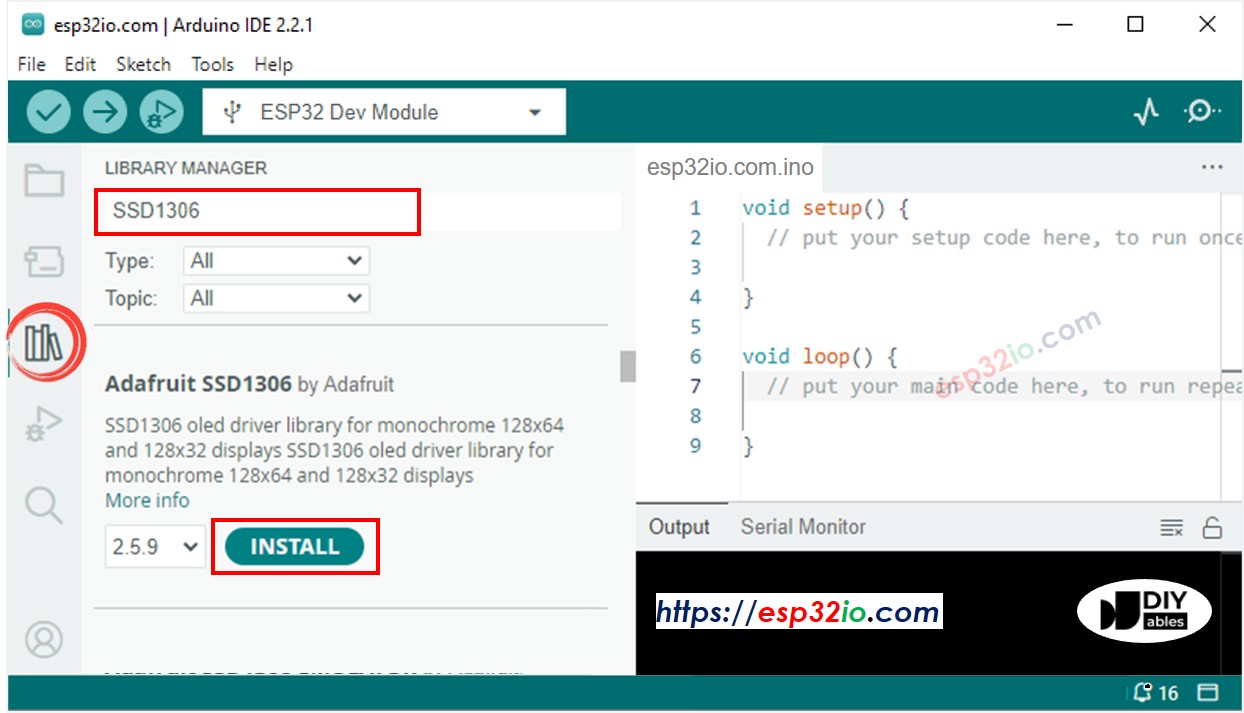
- Une fenêtre apparaît pour vous demander d'installer les dépendances pour la bibliothèque
- Installez toutes les dépendances pour la bibliothèque en cliquant sur le bouton Install All.
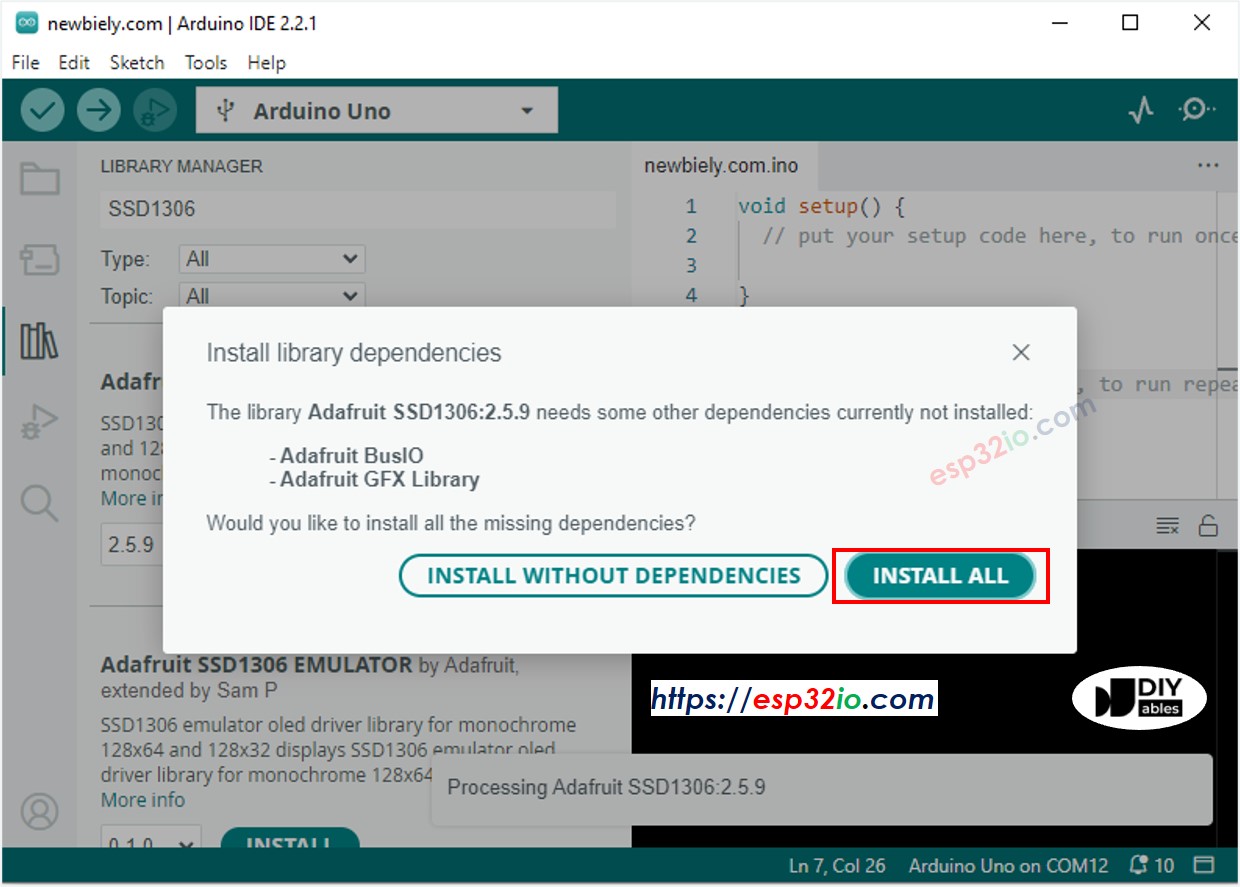
Comment programmer pour OLED
- Inclure la bibliothèque
- Définissez la taille de l'écran (123x64 ou 128x32)
- Déclarez un objet OLED SSD1306
- Dans la fonction setup(), initialisez l'affichage OLED.
- Et ensuite, vous pouvez afficher du texte, des images, tracer des lignes ...
Code ESP32 - Afficher du texte sur OLED
Le code ESP32 ci-dessous affiche un texte sur OLED.
Nous pouvons faire plus en utilisant les fonctions suivantes :
- oled.clearDisplay() : cette fonction est utilisée pour effacer l'affichage
- oled.setTextSize(n) : cette fonction est utilisée pour définir la taille de la police, elle prend en charge les tailles de 1 à 8
- oled.setTextColor(WHITE) : cette fonction est utilisée pour définir la couleur du texte
- oled.setCursor(x,y) : cette fonction est utilisée pour définir les coordonnées pour commencer à afficher le texte
- oled.drawPixel(x,y, color) : cette fonction est utilisée pour tracer un pixel aux coordonnées x,y
- oled.setTextColor(BLACK, WHITE) : cette fonction est utilisée pour définir respectivement la couleur du texte et la couleur de fond
- oled.println("message") : cette fonction est utilisée pour imprimer une chaîne de caractères
- oled.println(number) : cette fonction est utilisée pour imprimer un nombre
- oled.println(number, HEX) : cette fonction est utilisée pour imprimer un nombre au format hexadécimal
- oled.display() : cette fonction est utilisée pour appliquer les modifications
- oled.startscrollright(start, stop) : cette fonction est utilisée pour faire défiler le texte de gauche à droite
- oled.startscrollleft(start, stop) : cette fonction est utilisée pour faire défiler le texte de droite à gauche
- oled.startscrolldiagright(start, stop) : cette fonction est utilisée pour faire défiler le texte du coin inférieur gauche au coin supérieur droit
- oled.startscrolldiagleft(start, stop) : cette fonction est utilisée pour faire défiler le texte du coin inférieur droit au coin supérieur gauche
- oled.stopscroll() : cette fonction est utilisée pour arrêter le défilement
Code ESP32 - Dessiner des formes sur OLED
Le code ci-dessous montre comment dessiner plusieurs formes sur OLED.
Code ESP32 - Afficher une image sur OLED
Pour afficher une image sur un OLED, il est nécessaire de convertir l'image en tableau de bitmap. Il existe un outil en ligne pour convertir l'image en tableau de bitmap. L'image ci-dessous montre comment procéder. J'ai converti l'icône ESP32 en tableau de bitmap.
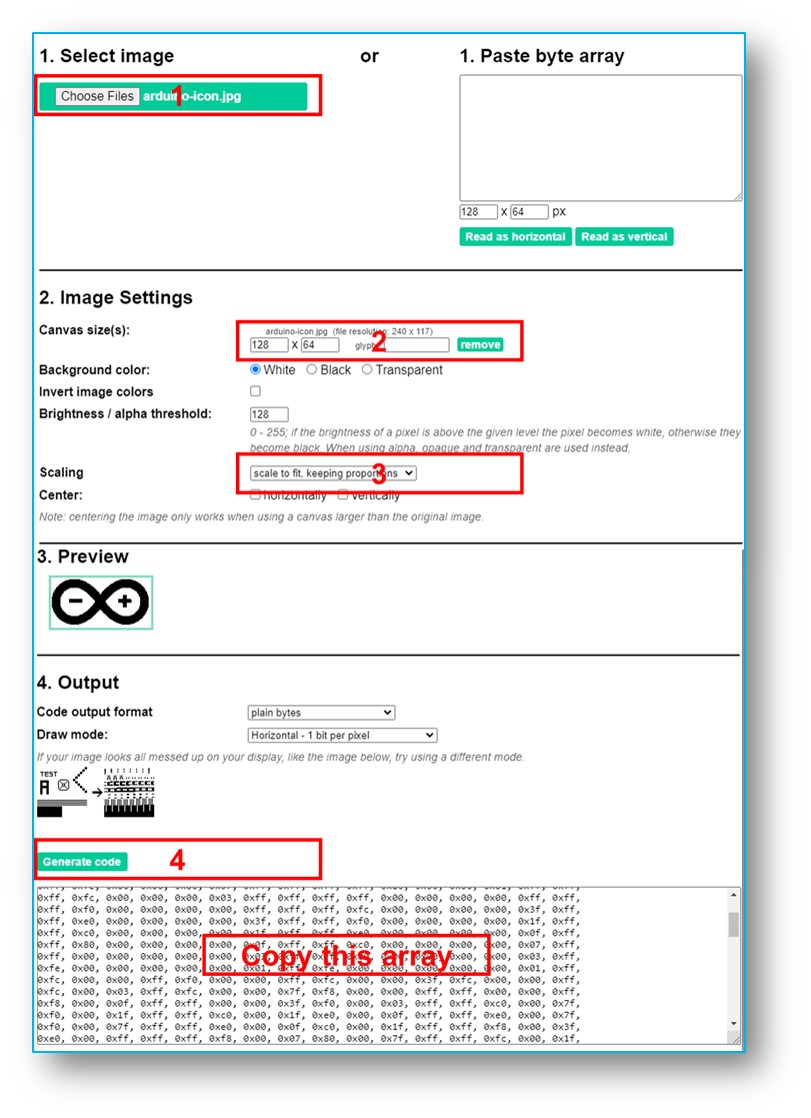
Après la conversion, copiez le code du tableau et mettez-le à jour dans le tableau arduino_icon dans le code suivant :
Dépannage OLED
Consultez le guide de dépannage OLED Dépannage OLED