Arduino Nano ESP32 - OLED
Ce tutoriel fournit des instructions sur comment utiliser Arduino Nano ESP32 avec un affichage OLED. En détail, nous apprendrons :
- Comment connecter un écran OLED avec Arduino Nano ESP32.
- Comment programmer l'Arduino Nano ESP32 pour afficher du texte et des nombres sur l'OLED.
- Comment programmer l'Arduino Nano ESP32 pour aligner centralement le texte et les nombres verticalement et horizontalement sur l'OLED.
- Comment programmer l'Arduino Nano ESP32 pour dessiner sur l'OLED.
- Comment programmer l'Arduino Nano ESP32 pour afficher une image sur l'OLED.
Préparation du matériel
1 | × | Arduino Nano ESP32 | |
1 | × | USB Cable Type-C | |
1 | × | SSD1306 I2C OLED Display 128x64 | |
1 | × | Jumper Wires | |
1 | × | (Optional) 9V Power Adapter for Arduino Nano ESP32 | |
1 | × | (Recommended) Screw Terminal Adapter for Arduino Nano |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos de l'écran OLED
Il existe différents types d'écrans OLED disponibles. L'OLED le plus couramment utilisé avec Arduino Nano ESP32 est l'écran OLED I2C SSD1306 128x64 et 128x32.
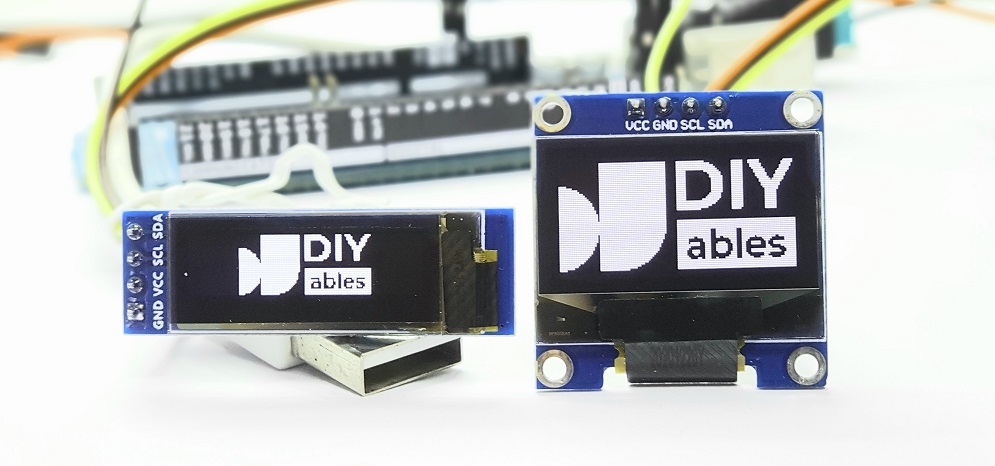
Brochage de l'affichage OLED I2C
- Broche GND : Celle-ci doit être connectée à la masse de l'Arduino Nano ESP32.
- Broche VCC : C'est l'alimentation pour l'affichage qui doit être connectée à 3,3V ou 5V.
- Broche SCL : C'est une broche d'horloge série pour l'interface I2C.
- Broche SDA : C'est une broche de données série pour l'interface I2C.
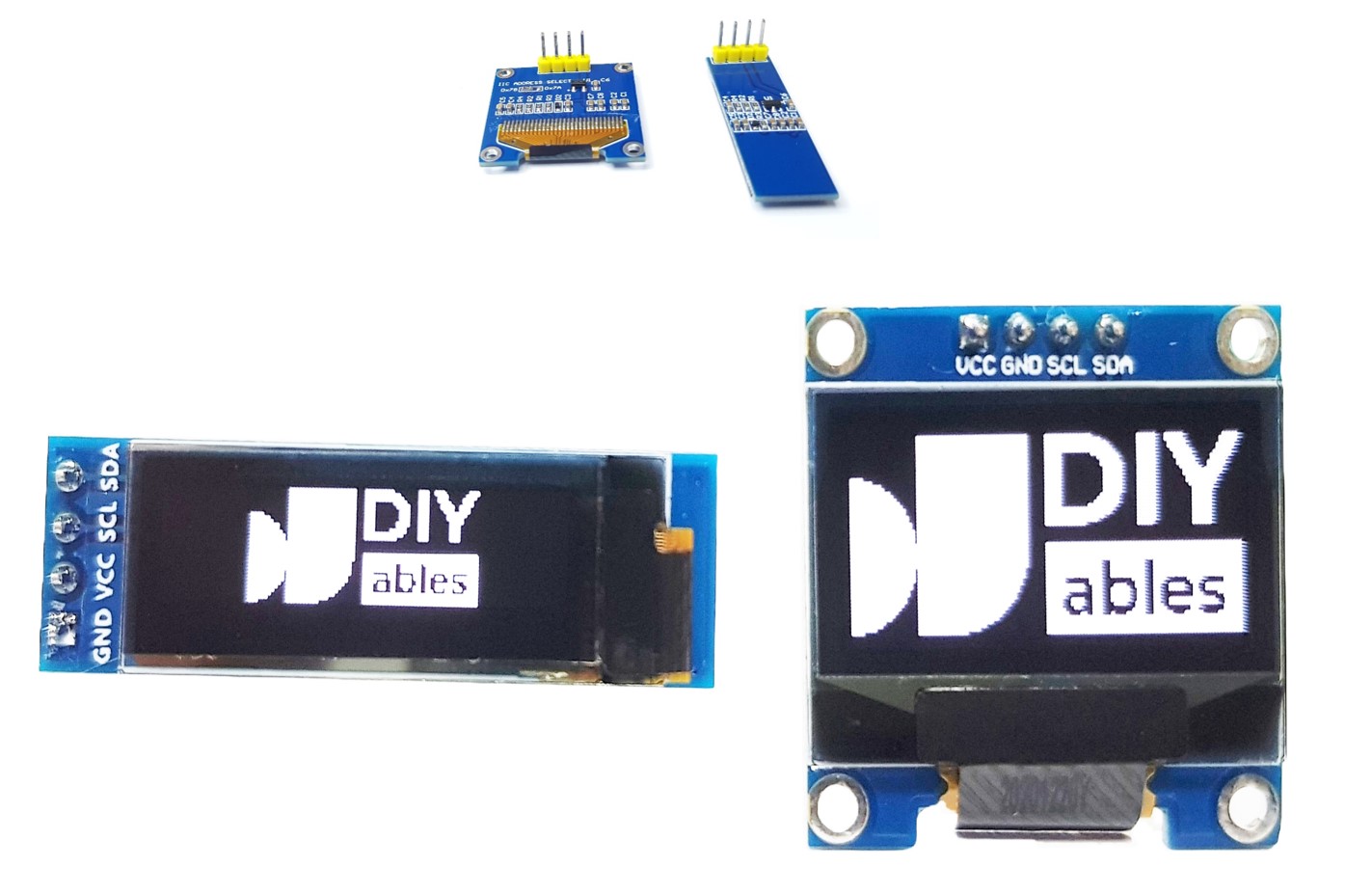
※ NOTE THAT:
- Les broches du module OLED peuvent varier selon le fabricant et le type. Veuillez utiliser les étiquettes imprimées sur le module OLED. Veuillez regarder de près !
- Ce tutoriel utilise l'écran OLED qui est piloté par le pilote I2C SSD1306. Nous l'avons testé avec l'écran OLED de DIYables et il fonctionne parfaitement.
Diagramme de câblage
- Schéma de câblage entre Arduino Nano ESP32 et OLED 128x64
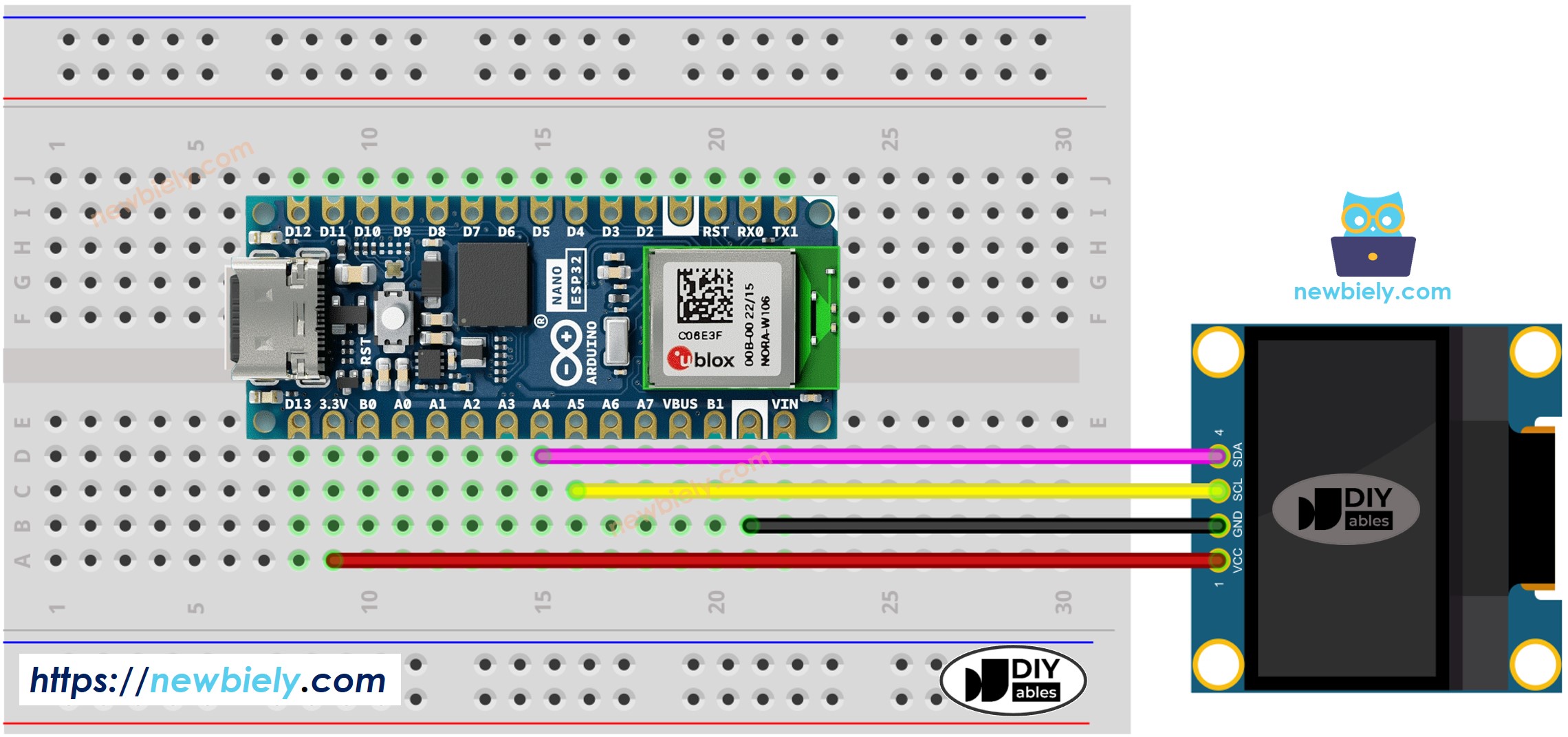
This image is created using Fritzing. Click to enlarge image
- Schéma de câblage entre Arduino Nano ESP32 et OLED 128x32
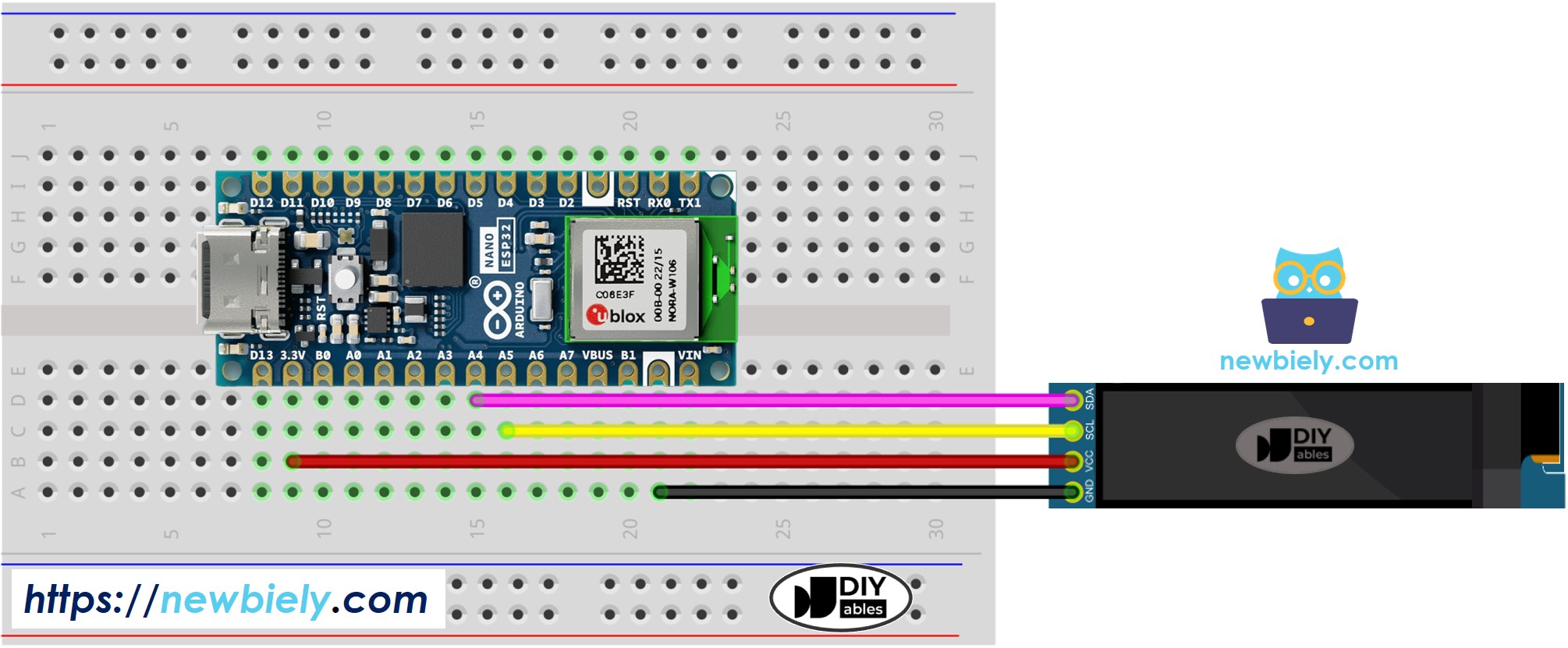
This image is created using Fritzing. Click to enlarge image
Le tableau de câblage entre Arduino Nano ESP32 et l'écran OLED :
OLED Module | Arduino Nano ESP32 |
---|---|
Vin | 3.3V |
GND | GND |
SDA | A4 |
SCL | A5 |
Comment utiliser OLED avec Arduino Nano ESP32
Installer la bibliothèque SSD1306 OLED
- Cliquez sur l'icône Libraries située sur la barre de gauche de l'IDE Arduino.
- Recherchez "SSD1306" et localisez la bibliothèque SSD1306 d'Adafruit.
- Ensuite, appuyez sur le bouton Install pour terminer l'installation.
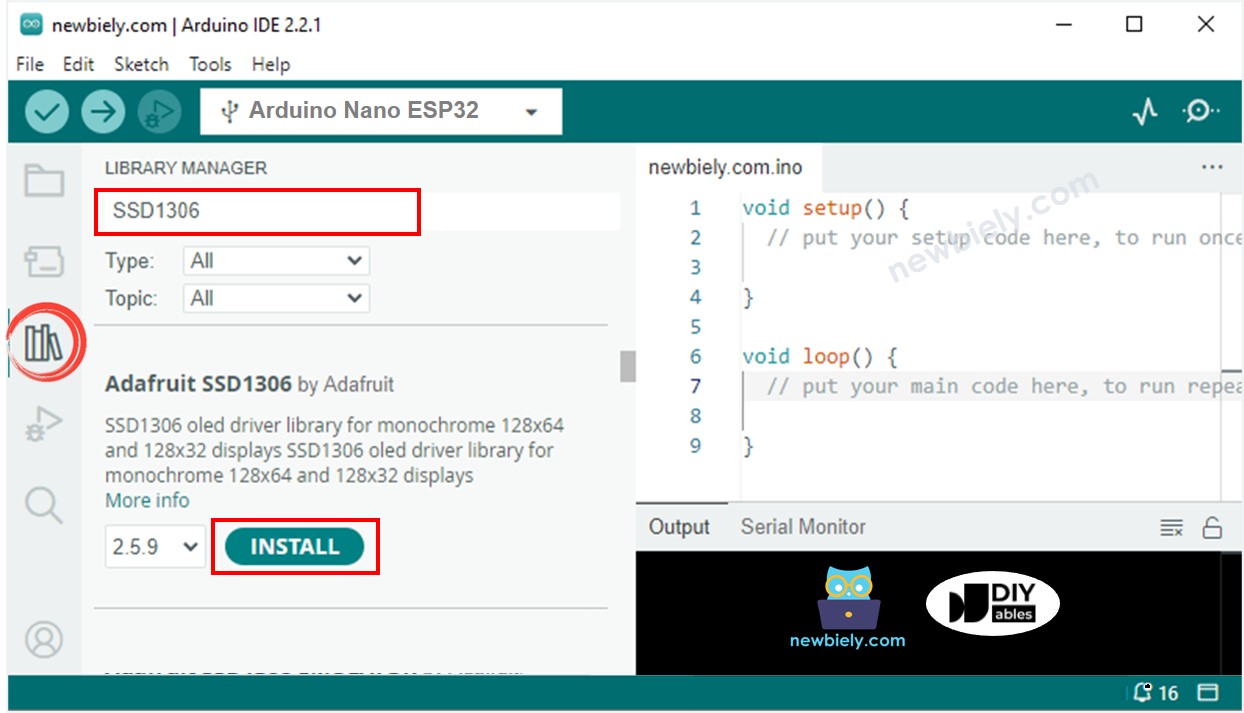
- Vous serez invité à installer des dépendances de bibliothèque supplémentaires.
- Pour les installer toutes, cliquez sur le bouton Installer Tout.
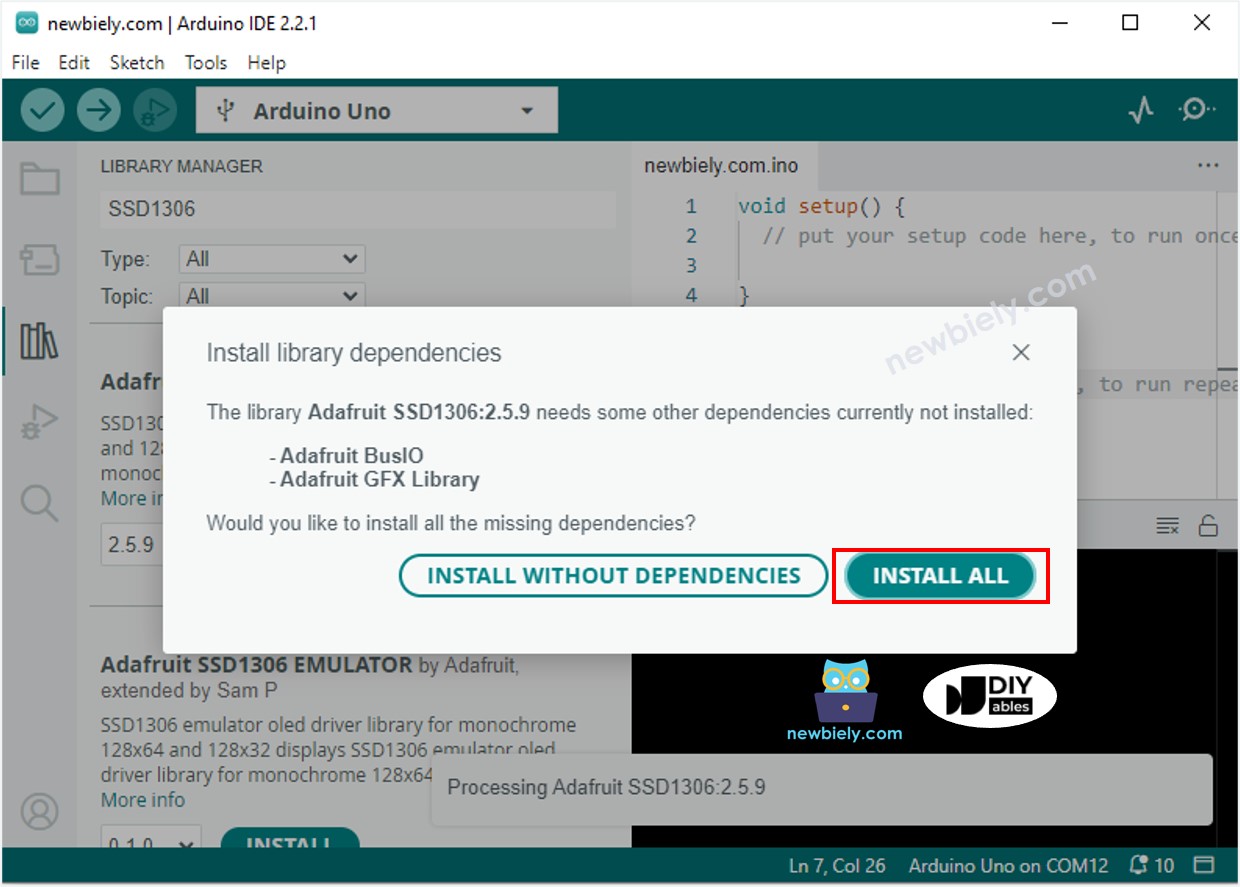
Comment programmer pour OLED
- Inclure la bibliothèque
- Spécifiez les dimensions de l'écran OLED comme étant de 128 x 64.
- Ou spécifiez les dimensions d'un écran OLED qui est de 128x32.
- Créez un objet de type SSD1306 OLED.
- Dans la fonction setup(), initialisez l'affichage OLED.
- Ensuite, vous pouvez afficher du texte, des images, et tracer une ligne...
À partir de maintenant, tous les codes seront pour OLED 128x64. Cependant, il est facile de les modifier pour OLED 128x32 en modifiant la taille de l'écran et en ajustant les coordonnées si nécessaire.
Code Arduino Nano ESP32 - Afficher du texte sur OLED
Voici quelques fonctions qui peuvent être utilisées pour afficher du texte sur l'OLED :
- oled.clearDisplay(): tous les pixels sont éteints.
- oled.drawPixel(x,y, couleur): trace un pixel aux coordonnées x, y.
- oled.setTextSize(n): définit la taille de la police, prend en charge les tailles de 1 à 8.
- oled.setCursor(x,y): définit les coordonnées pour commencer à écrire du texte.
- oled.setTextColor(WHITE): définit la couleur du texte.
- oled.setTextColor(BLACK, WHITE): définit la couleur du texte, couleur de fond.
- oled.println("message"): imprime les caractères.
- oled.println(nombre): imprime un nombre.
- oled.println(nombre, HEX): imprime un nombre au format hexadécimal.
- oled.display(): appelez cette méthode pour que les modifications prennent effet.
- oled.startscrollright(start, stop): fait défiler le texte de gauche à droite.
- oled.startscrollleft(start, stop): fait défiler le texte de droite à gauche.
- oled.startscrolldiagright(start, stop): fait défiler le texte du coin inférieur gauche au coin supérieur droit.
- oled.startscrolldiagleft(start, stop): fait défiler le texte du coin inférieur droit au coin supérieur gauche.
- oled.stopscroll(): arrête le défilement.
Comment centrer verticalement et horizontalement du texte ou des chiffres sur un OLED
Pour des informations sur la façon d'aligner verticalement et horizontalement des textes et des chiffres sur un affichage OLED, veuillez consulter Comment centrer verticalement/horizontalement sur OLED.
Code Arduino Nano ESP32 - Dessin sur OLED
Code Arduino Nano ESP32 – Afficher une image
Afin d'afficher une image sur un OLED, nous devons d'abord convertir l'image (dans n'importe quel format) en un tableau de bitmap. Cela peut être réalisé en utilisant l'outil en ligne. L'image suivante montre comment convertir une image en tableau de bitmap ; j'ai utilisé l'icône Arduino Nano ESP32 comme exemple.
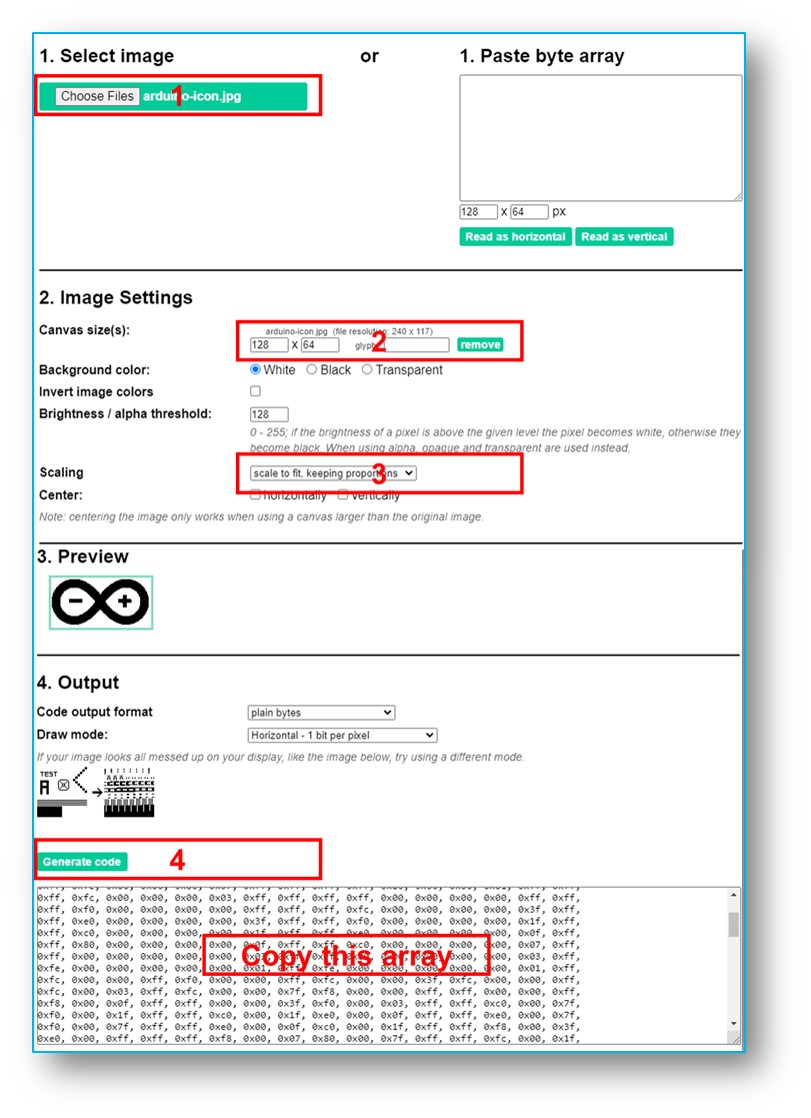
Après la conversion, prenez le code du tableau et mettez-le à la place du tableau ArduinoIcon dans le code ci-dessous.
※ NOTE THAT:
- La taille de l'image ne doit pas être plus grande que celle de l'écran.
- Si vous souhaitez utiliser le code pour un OLED 128x32, vous devez redimensionner l'image et ajuster les paramètres de largeur et de hauteur dans la fonction oled.drawBitmap();.
#Dépannage OLED
Vérifiez que l'OLED fonctionne correctement en procédant comme suit :
- Assurez-vous que votre câblage est correct.
- Confirmez que votre OLED utilise le pilote SSD1306.
- Utilisez le code Scanner d'adresse I2C sur Arduino Nano ESP32 pour vérifier l'adresse I2C de l'OLED.
La sortie affichée sur le moniteur série est la suivante :