ESP8266 - Feu de circulation
Ce tutoriel vous explique comment utiliser un ESP8266 pour contrôler un module de feu de circulation. Plus précisément, nous aborderons les aspects suivants :
- Établissement de la connexion entre le module de feu de signalisation et l'ESP8266
- Programmation de l'ESP8266 pour superviser le module de feu de circulation RGB
- Mise en œuvre de la programmation ESP8266 pour gérer le module de feu de circulation RGB sans dépendre de la fonction delay()
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos du module de feu de circulation
Brochage
Un module de feu de circulation est équipé de quatre broches :
- Broche GND : Connectez cette broche à la GND de l'ESP8266.
- Broche R : Gère la lumière rouge ; connectez cette broche à une sortie numérique de l'ESP8266.
- Broche Y : Contrôle la lumière jaune ; connectez cette broche à une sortie numérique de l'ESP8266.
- Broche G : Régule la lumière verte ; connectez cette broche à une sortie numérique de l'ESP8266.
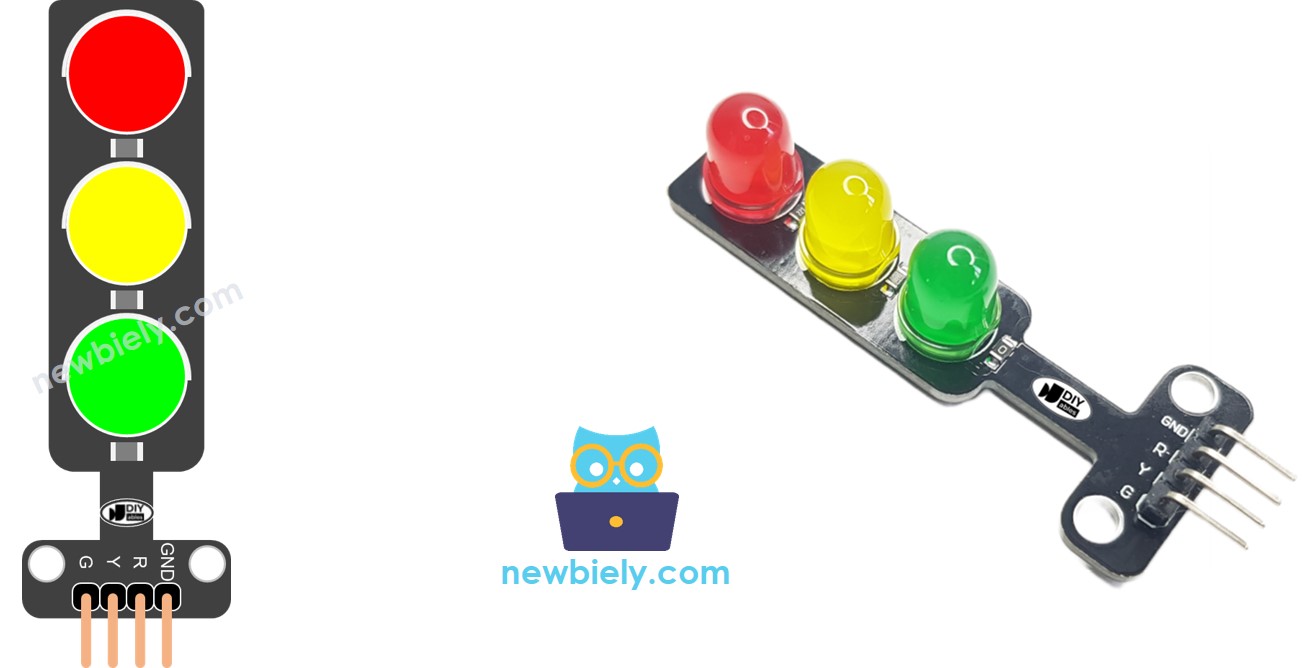
Comment ça fonctionne
Diagramme de câblage
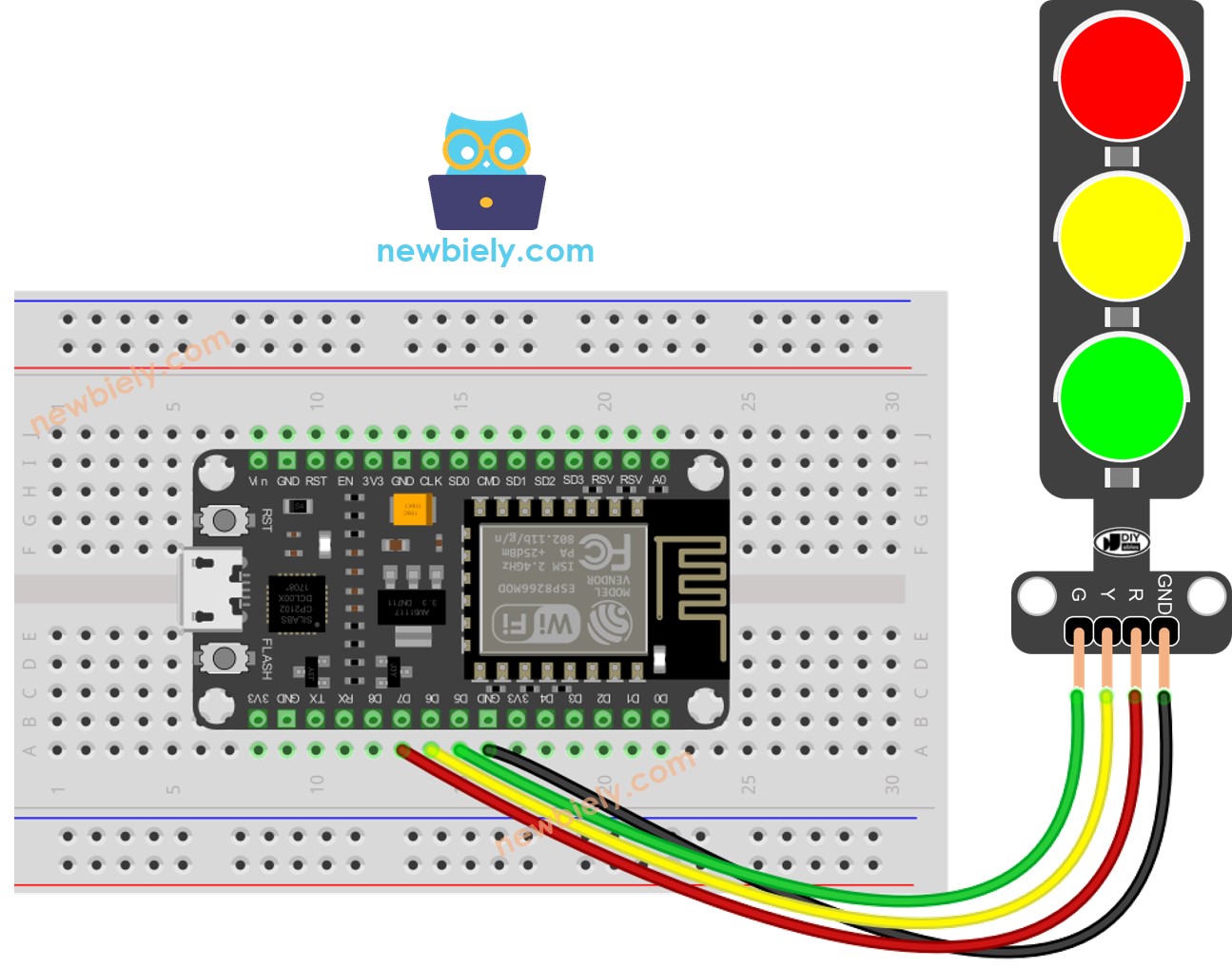
This image is created using Fritzing. Click to enlarge image
Voir plus dans Brochage ESP8266. et Comment alimenter l'ESP8266..
Comment programmer le module de feu de signalisation
- Configurez les broches d'un ESP8266 en mode sortie numérique en utilisant la fonction pinMode().
- Programme pour allumer une lumière rouge en utilisant la fonction digitalWrite() :
Code ESP8266
Étapes rapides
Pour commencer avec l'ESP8266 sur Arduino IDE, suivez ces étapes :
- Consultez le tutoriel Installation du logiciel ESP8266. si c'est votre première utilisation de l'ESP8266.
- Connectez les composants comme indiqué dans le schéma.
- Branchez la carte ESP8266 à votre ordinateur via un câble USB.
- Ouvrez Arduino IDE sur votre ordinateur.
- Sélectionnez la bonne carte ESP8266, comme (par exemple NodeMCU 1.0 (Module ESP-12E)), et son port COM respectif.
- Copiez le code ci-dessus et ouvrez-le avec Arduino IDE
- Cliquez sur le bouton Upload sur Arduino IDE pour téléverser le code vers ESP8266
- Consultez le module de feu de circulation
Il est important de noter que le fonctionnement exact d'un feu de circulation peut varier en fonction du design spécifique et de la technologie utilisée dans différentes régions et intersections. Les principes décrits ci-dessus fournissent une compréhension générale de la manière dont les feux de circulation fonctionnent pour gérer le trafic et améliorer la sécurité sur les routes.
Le code ci-dessus démontre le contrôle individuel des lumières. Maintenant, améliorons le code pour une meilleure optimisation.
Optimisation du code ESP8266
- Améliorons le code en implémentant une fonction de contrôle de la lumière.
- Améliorons le code en utilisant une boucle for.
- Améliorons le code en utilisant la fonction millis() au lieu de delay().