ESP8266 - GPS
Ce tutoriel vous explique comment utiliser l'ESP8266 avec un module GPS NEO-6M. En détail, nous allons apprendre :
- Comment connecter un ESP8266 à un module GPS NEO-6M
- Comment programmer l'ESP8266 pour lire les coordonnées GPS (longitude, latitude et altitude) à partir d'un module GPS NEO-6M
- Comment programmer l'ESP8266 pour calculer la distance entre la position GPS actuelle et une coordonnée GPS prédéfinie (par exemple, les coordonnées de Londres).
Outre la longitude, la latitude et l'altitude, l'ESP8266 peut également lire la vitesse GPS (km/h) et l'heure à partir d'un module GPS NEO-6M.
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos du module GPS NEO-6M
Le module GPS NEO-6M est un module GPS qui peut fournir les informations suivantes :
- Longitude
- Latitude
- Altitude
- Vitesse GPS (km/h)
- Date et heure
Brochage du module GPS NEO-6M
Le module GPS NEO-6M dispose de 4 broches :
- Broche VCC : Celle-ci doit être connectée à VCC (5V)
- Broche GND : Celle-ci doit être connectée à GND (0V)
- Broche TX : Elle est destinée à la communication série et doit être connectée à la broche RX de Serial (ou SoftwareSerial) sur ESP8266.
- Broche RX : Elle est destinée à la communication série et doit être connectée à la broche TX de Serial (ou SoftwareSerial) sur ESP8266.
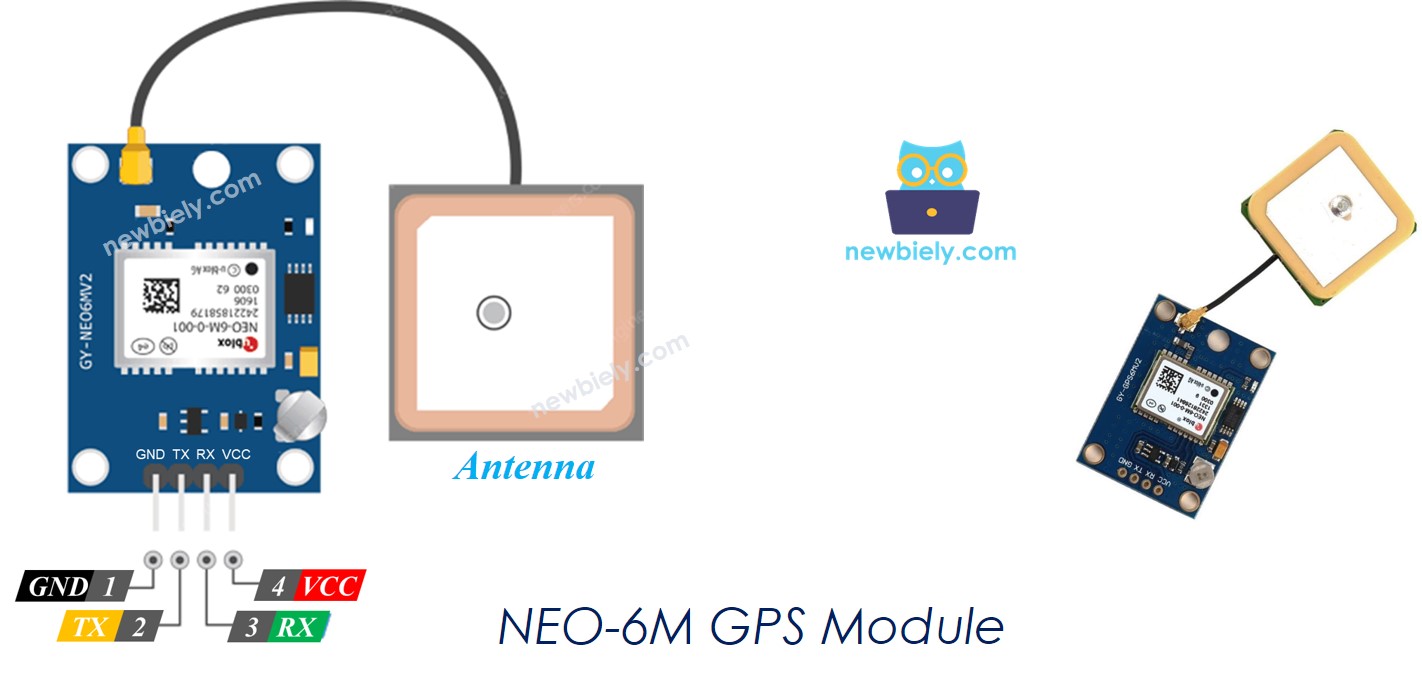
Diagramme de câblage
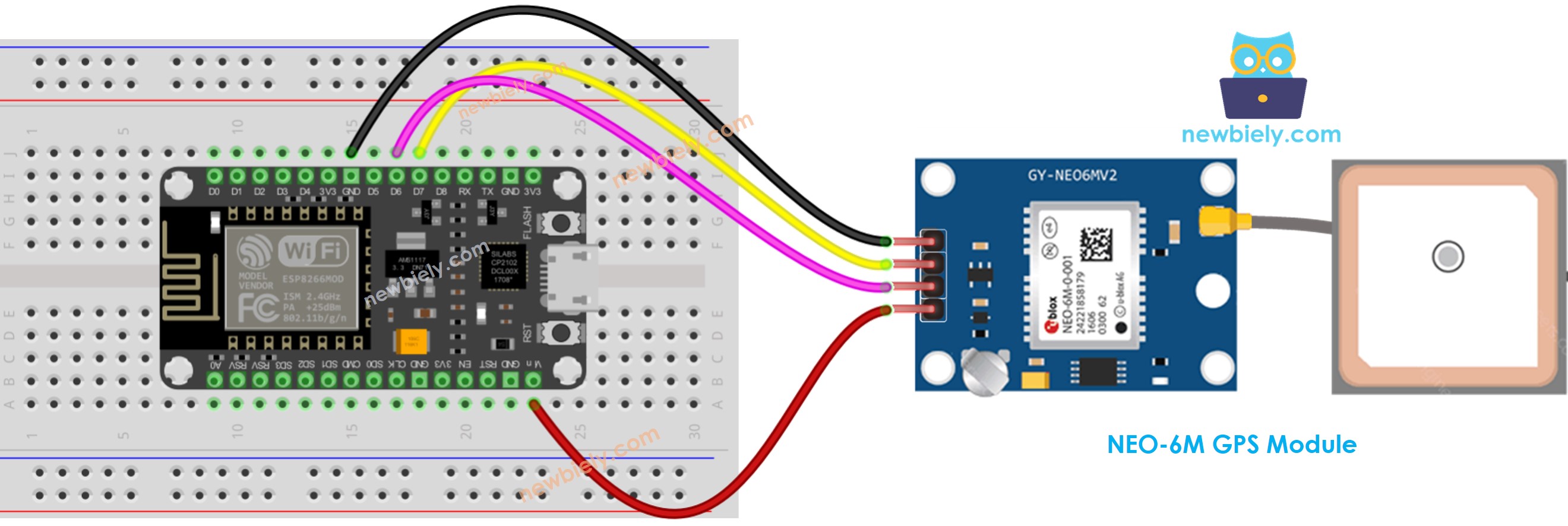
This image is created using Fritzing. Click to enlarge image
Voir plus dans Brochage ESP8266. et Comment alimenter l'ESP8266..
Code ESP8266
Lecture des coordonnées GPS, de la vitesse (km/h) et de la date_heure.
Étapes rapides
Pour commencer avec l'ESP8266 sur Arduino IDE, suivez ces étapes :
- Consultez le tutoriel Installation du logiciel ESP8266. si c'est votre première utilisation de l'ESP8266.
- Connectez les composants comme indiqué dans le schéma.
- Connectez la carte ESP8266 à votre ordinateur à l'aide d'un câble USB.
- Ouvrez Arduino IDE sur votre ordinateur.
- Choisissez la bonne carte ESP8266, comme (par exemple NodeMCU 1.0 (Module ESP-12E)), et son port COM respectif.
- Lancez Arduino IDE
- Cliquez sur l'icône Libraries dans la barre latérale gauche de l'Arduino IDE.
- Recherchez "TinyGPSPlus", puis trouvez la bibliothèque TinyGPSPlus de Mikal Hart
- Cliquez sur le bouton Install pour installer la bibliothèque TinyGPSPlus.
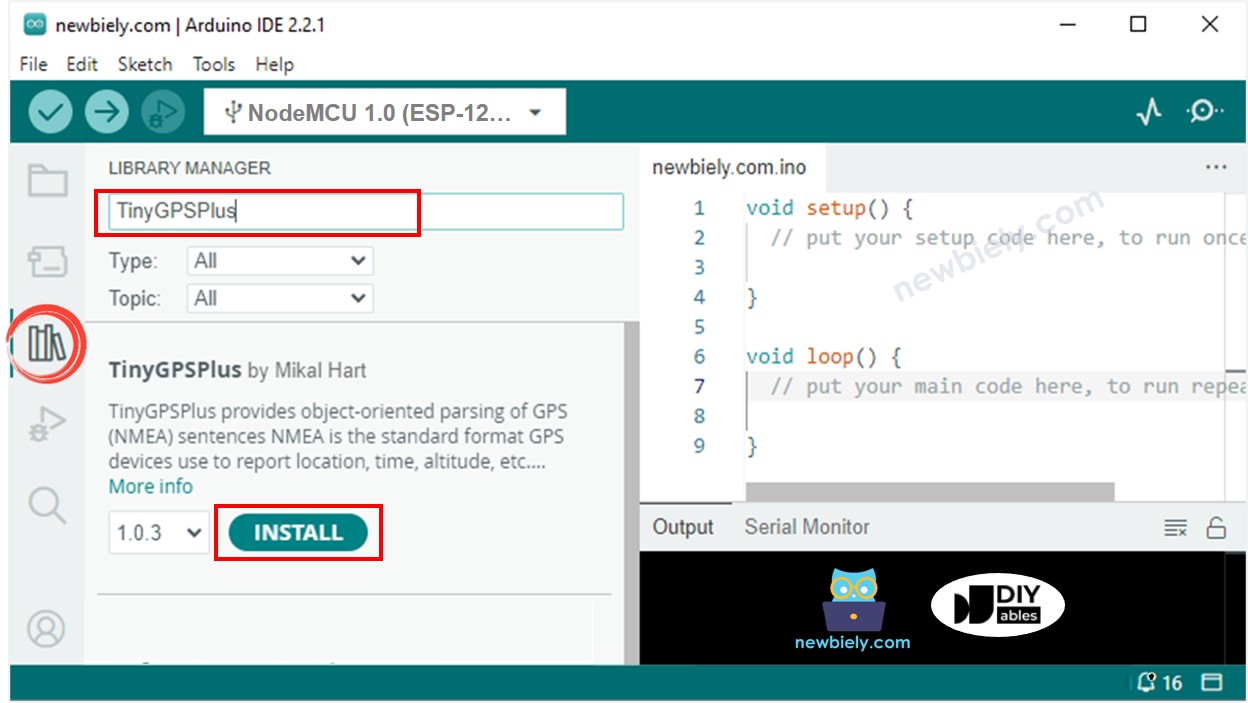
- Copiez le code fourni et ouvrez-le dans l'IDE Arduino.
- Cliquez sur le bouton Upload dans l'IDE Arduino pour envoyer le code à l'ESP8266.
- Consultez le résultat sur le moniteur série.
Calcul de la distance entre la position actuelle et un lieu prédéfini
Ce code calcule la distance entre l'emplacement actuel et Londres (latitude : 51.508131, longitude : -0.128002).
Étapes rapides
- Câblez les composants comme indiqué sur le schéma.
- Connectez la carte ESP8266 à votre ordinateur à l'aide d'un câble USB.
- Ouvrez Arduino IDE sur votre ordinateur.
- Choisissez la bonne carte ESP8266, telle que (par exemple NodeMCU 1.0 (Module ESP-12E)), et son port COM respectif.
- Copiez le code et ouvrez-le dans l'Arduino IDE.
- Cliquez sur le bouton Upload dans l'Arduino IDE pour envoyer le code à l'ESP8266.
- Consultez le résultat sur le moniteur série.