ESP8266 - OLED
Ce tutoriel vous explique comment utiliser l'ESP8266 avec un affichage OLED. En détail, nous allons apprendre :
- Comment connecter un écran OLED avec ESP8266.
- Comment programmer l'ESP8266 pour afficher du texte et des nombres sur OLED.
- Comment programmer l'ESP8266 pour aligner centralement le texte et les nombres verticalement et horizontalement sur OLED.
- Comment programmer l'ESP8266 pour dessiner sur OLED.
- Comment programmer l'ESP8266 pour afficher une image sur OLED.
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos de l'écran OLED
Il existe différents types d'écrans OLED disponibles. L'OLED le plus couramment utilisé avec ESP8266 est l'OLED I2C SSD1306 de 128x64 et 128x32.
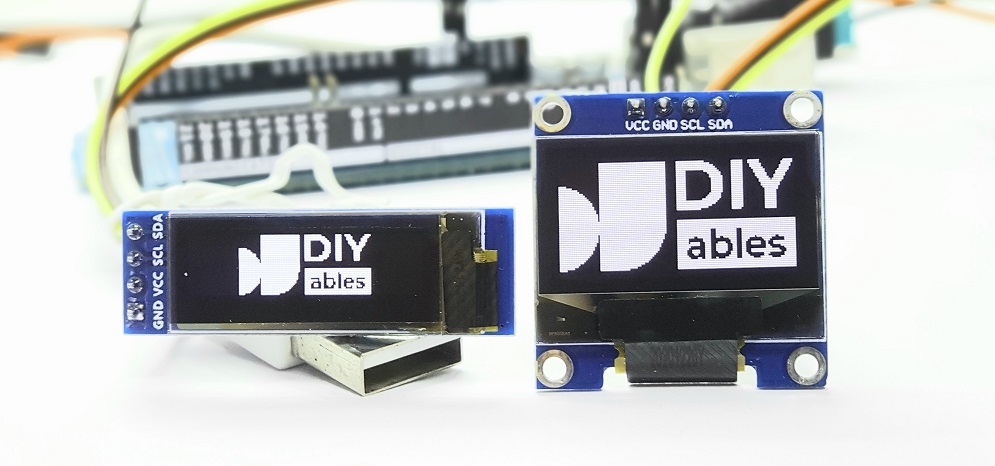
Brochage d'affichage OLED I2C
- Broche GND : doit être connectée à la masse de l'ESP8266.
- Broche VCC : est l'alimentation pour l'affichage qui doit être connectée à 3.3V ou 5V.
- Broche SCL : est une broche d'horloge série pour l'interface I2C.
- Broche SDA : est une broche de données série pour l'interface I2C.
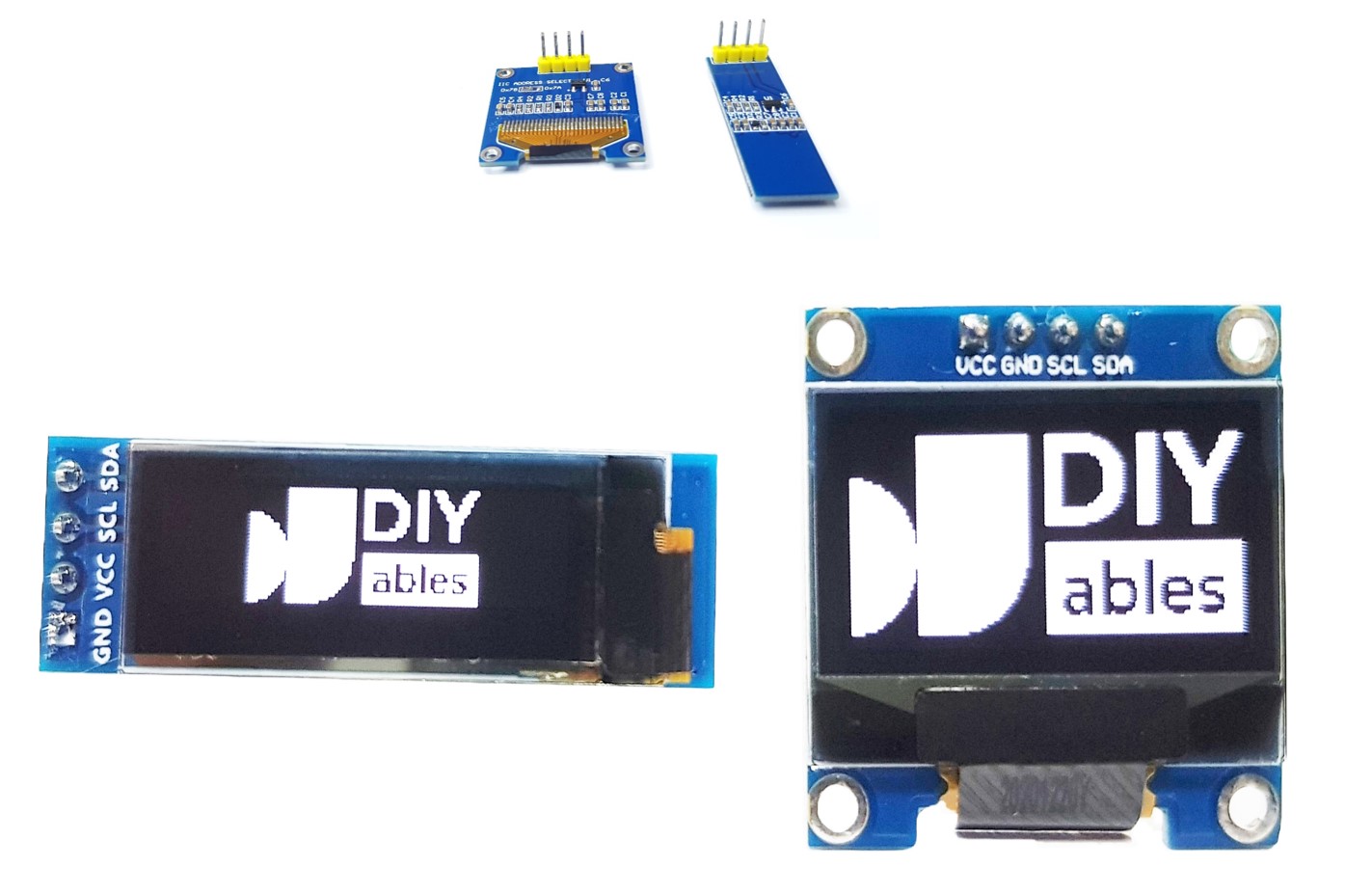
※ Note:
- Les broches d'un module OLED peuvent varier selon les fabricants et les types de modules. Il est essentiel de toujours se référer aux étiquettes imprimées sur le module OLED. Faites attention !
- Ce tutoriel utilise l'affichage OLED qui possède le pilote I2C SSD1306. Nous l'avons testé avec l'affichage OLED de DIYables et il fonctionne parfaitement.
Diagramme de câblage
- Schéma de câblage entre ESP8266 et OLED 128x64
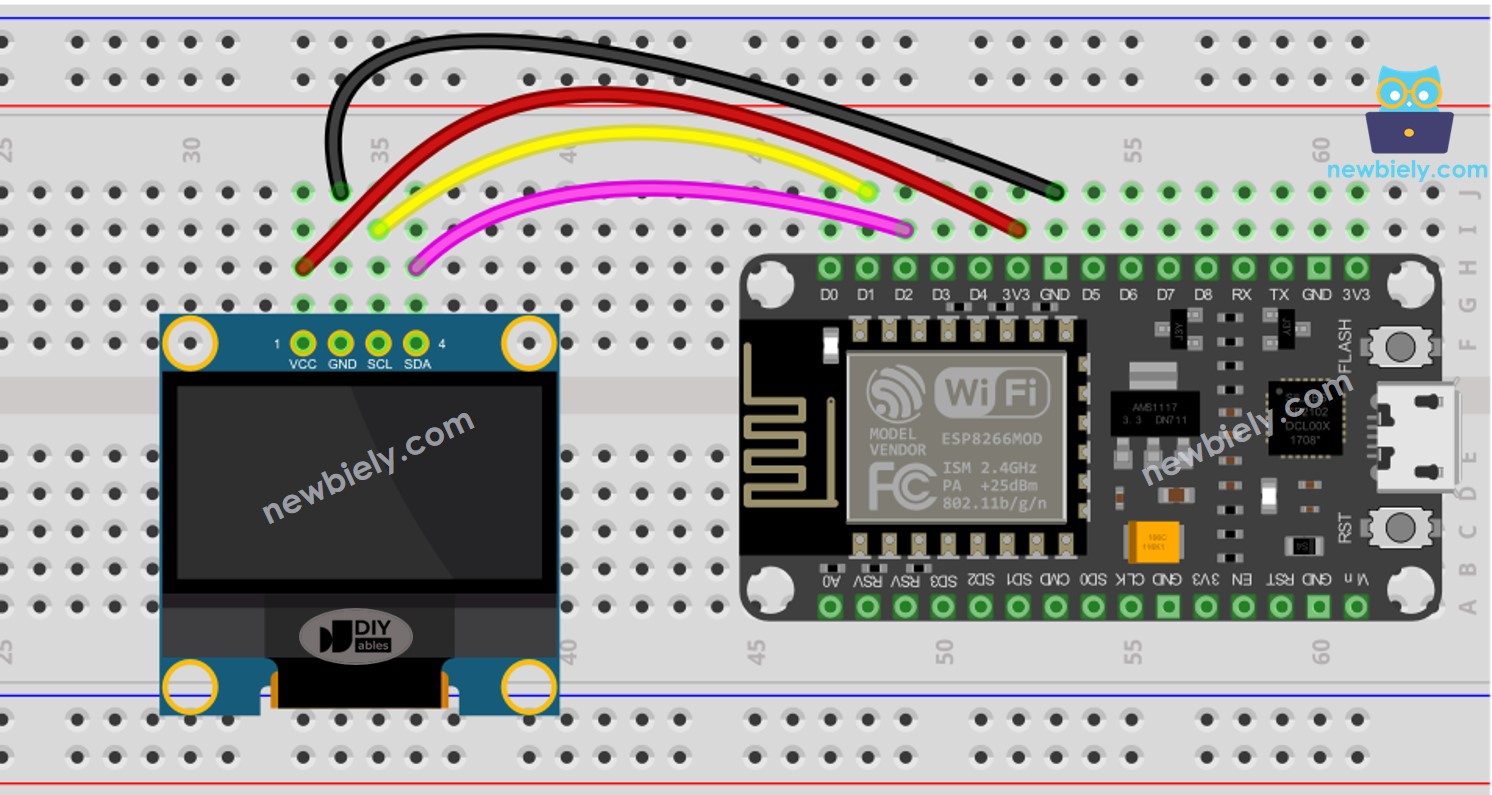
This image is created using Fritzing. Click to enlarge image
Voir plus dans Brochage ESP8266. et Comment alimenter l'ESP8266..
- Schéma de câblage entre ESP8266 et OLED 128x32
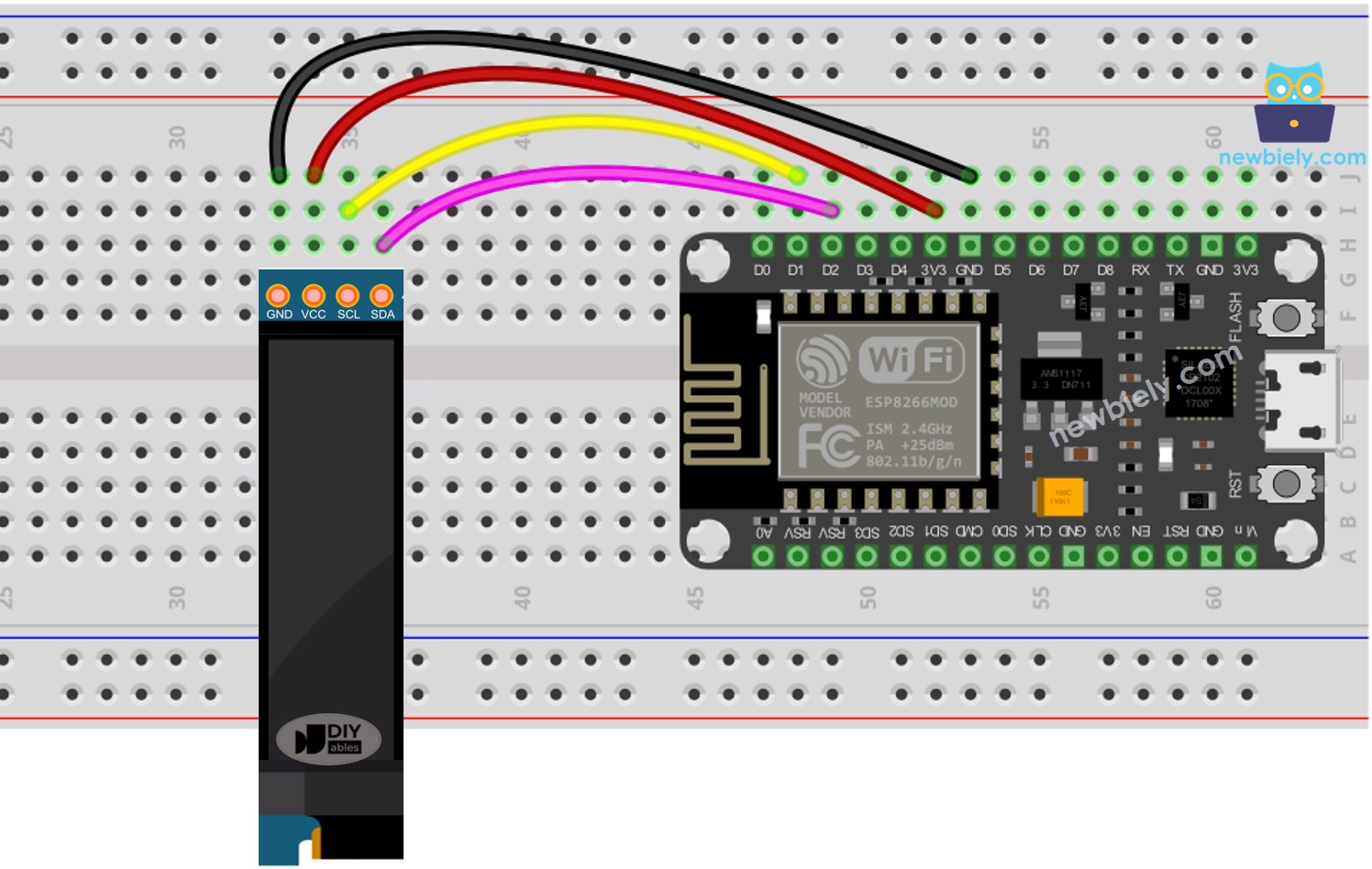
This image is created using Fritzing. Click to enlarge image
Le tableau de câblage entre ESP8266 et l'affichage OLED:
OLED Module | ESP8266 |
---|---|
Vin | 3.3V |
GND | GND |
SDA | D2 (GPIO4) |
SCL | D1 (GPIO5) |
Comment utiliser OLED avec ESP8266
Installez la bibliothèque OLED SSD1306
- Cliquez sur l'icône Libraries dans la barre latérale gauche de l'IDE Arduino.
- Recherchez "SSD1306" et localisez la bibliothèque SSD1306 d'Adafruit.
- Ensuite, appuyez sur le bouton Install pour terminer l'installation.
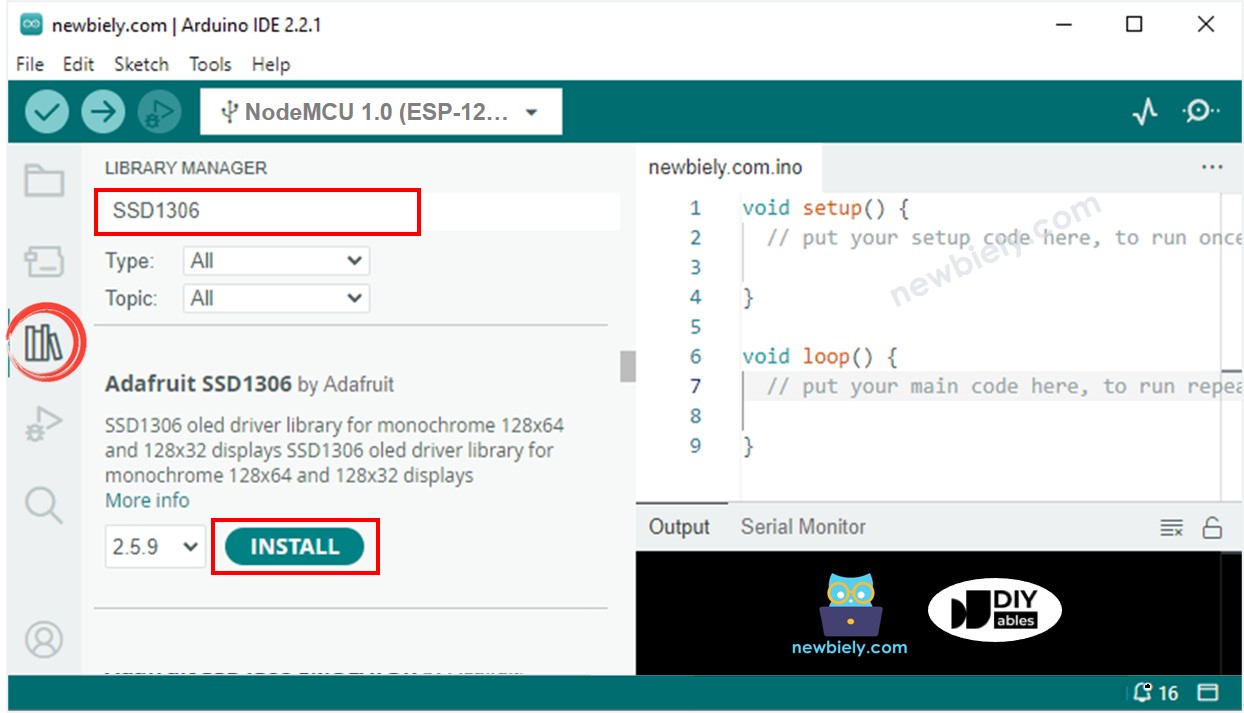
- Vous serez invité à installer d'autres dépendances de bibliothèques.
- Pour les installer toutes, cliquez sur le bouton Install All.
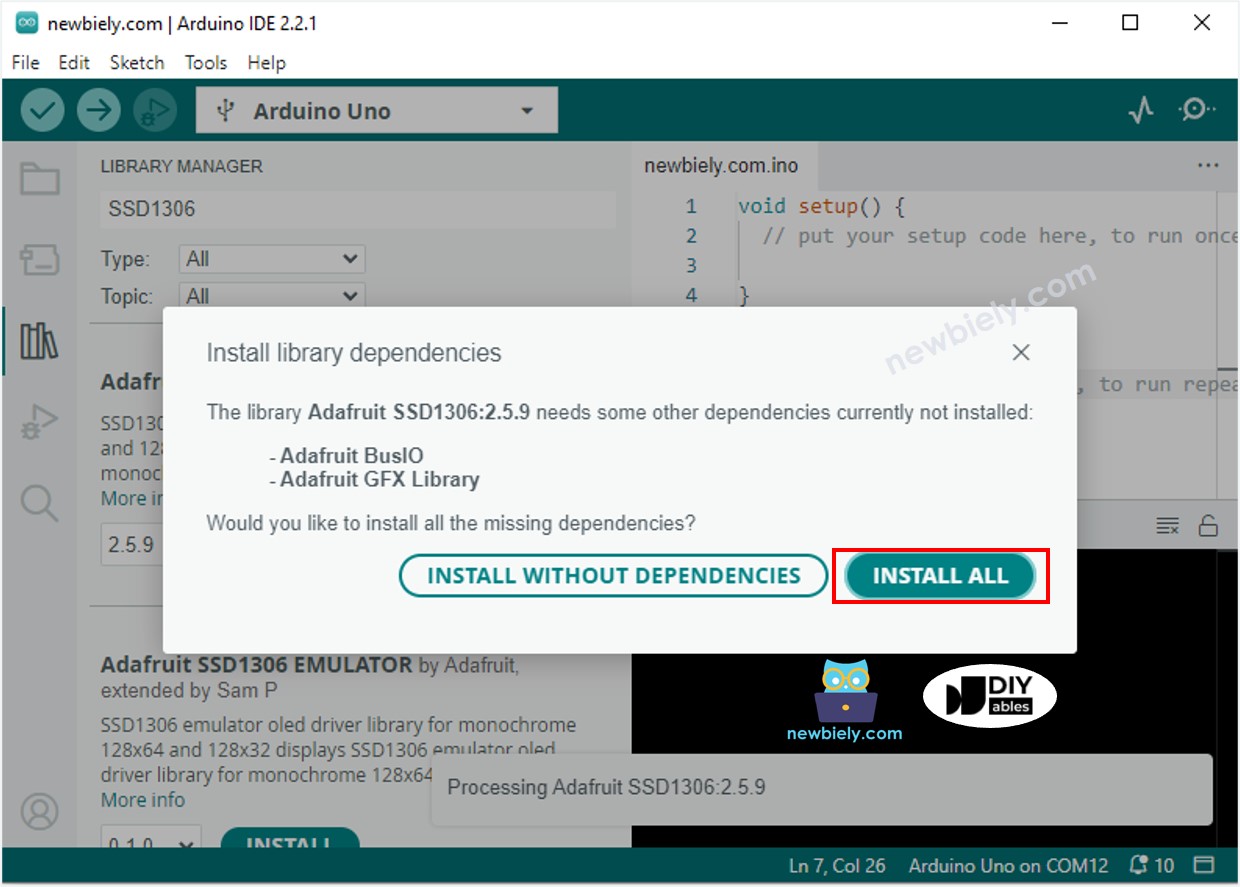
Comment programmer pour OLED
- Inclure la bibliothèque
- Spécifiez les dimensions de l'écran OLED comme 128 x 64.
- Spécifiez les dimensions d'un écran OLED qui est de 128x32.
- Créez un objet de type SSD1306 OLED.
- Dans la fonction setup(), initialisez l'écran OLED.
- Ensuite, vous pouvez afficher du texte, des images et créer des lignes...
À partir de maintenant, tous les codes sont pour OLED 128x64, mais ils peuvent être adaptés pour OLED 128x32 en modifiant la taille de l'écran et en ajustant les coordonnées si nécessaire.
Code ESP8266 - Afficher du texte sur OLED
Voici quelques fonctions qui peuvent être utilisées pour afficher du texte sur l'OLED :
- oled.clearDisplay() : tous les pixels sont éteints.
- oled.drawPixel(x,y, color) : trace un pixel aux coordonnées x,y.
- oled.setTextSize(n) : définit la taille de la police, prend en charge les tailles de 1 à 8.
- oled.setCursor(x,y) : définit les coordonnées pour commencer à écrire du texte.
- oled.setTextColor(WHITE) : définit la couleur du texte.
- oled.setTextColor(BLACK, WHITE) : définit la couleur du texte, couleur de fond.
- oled.println("message") : imprime les caractères.
- oled.println(number) : imprime un nombre.
- oled.println(number, HEX) : imprime un nombre au format hexadécimal.
- oled.display() : appelez cette méthode pour que les modifications prennent effet.
- oled.startscrollright(start, stop) : fait défiler le texte de gauche à droite.
- oled.startscrollleft(start, stop) : fait défiler le texte de droite à gauche.
- oled.startscrolldiagright(start, stop) : fait défiler le texte du coin inférieur gauche au coin supérieur droit.
- oled.startscrolldiagleft(start, stop) : fait défiler le texte du coin inférieur droit au coin supérieur gauche.
- oled.stopscroll() : arrête le défilement.
Comment centrer verticalement et horizontalement du texte/un nombre sur un OLED.
Pour en savoir plus sur le centrage du texte et des chiffres sur OLED, veuillez consulter Comment centrer verticalement/horizontalement sur OLED. Si vous souhaitez savoir comment centrer du texte et des chiffres sur OLED, consultez Comment centrer verticalement/horizontalement sur OLED.
Code ESP8266 - Dessin sur OLED
Code ESP8266 – Afficher une image
Afin d'afficher une image sur l'OLED, nous devons d'abord la convertir de son format original en un tableau de bitmap. Cela peut être fait en utilisant l'outil en ligne fourni. L'image suivante montre comment convertir une image en tableau de bitmap. À titre d'exemple, j'ai converti l'icône Arduino en un tableau de bitmap.
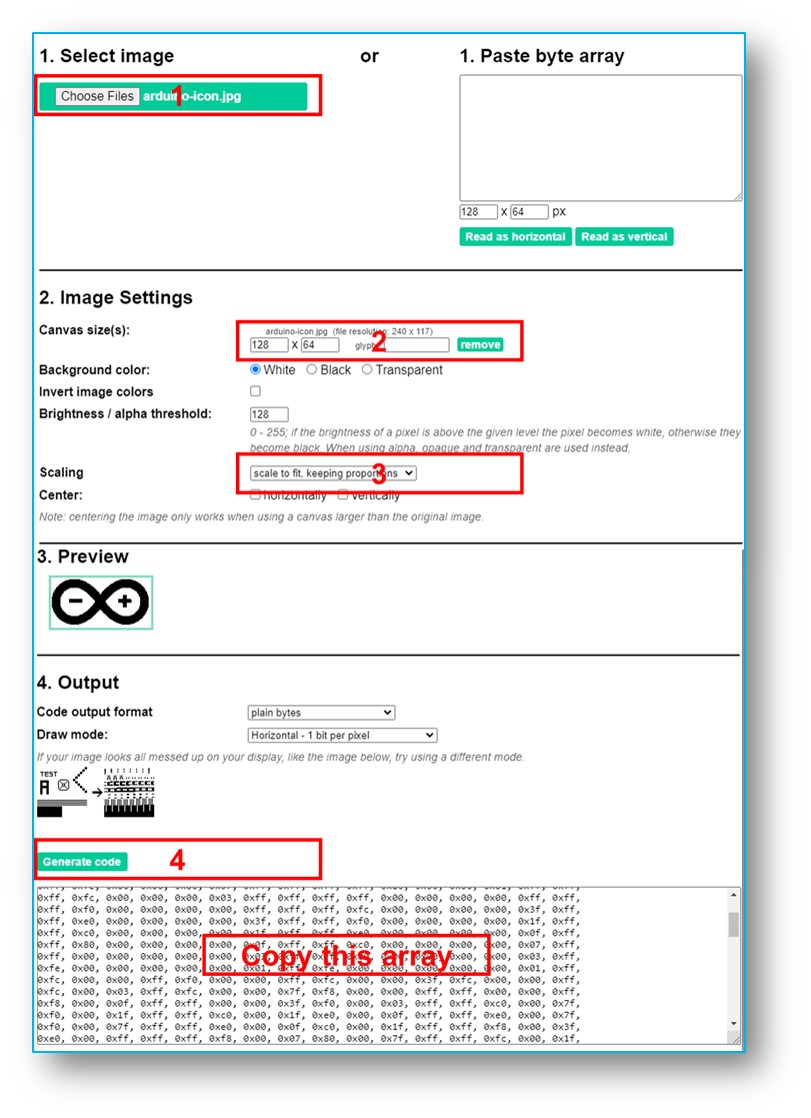
Une fois la conversion terminée, prenez le code du tableau et remplacez le tableau ArduinoIcon dans le code ci-dessous par celui-ci.
※ Note:
- La taille de l'image ne doit pas dépasser la taille de l'écran.
- Si vous souhaitez utiliser le code pour un OLED 128x32, vous devez redimensionner l'image et modifier la largeur et la hauteur dans la fonction oled.drawBitmap();.
Dépannage OLED
Vérifiez que l'OLED fonctionne correctement en suivant ces étapes :
- Assurez-vous que votre câblage est correct.
- Confirmez que votre OLED I2C utilise le pilote SSD1306.
- Utilisez le code du Scanner d'Adresse I2C sur ESP8266 pour vérifier l'adresse I2C de l'OLED.
La sortie affichée sur le moniteur série est : . Le moniteur série affiche le résultat suivant :