ESP8266 - Horloge temps réel
Ce tutoriel vous explique comment utiliser l'ESP8266 pour lire la date et l'heure à partir d'un module RTC. En détail, nous apprendrons :
- Comment connecter le module d'horloge temps réel DS3231 à l'ESP8266.
- Comment programmer l'ESP8266 pour lire la date et l'heure (seconde, minute, heure, jour de la semaine, jour du mois, mois et année) à partir du module RTC DS3231.
- Comment programmer l'ESP8266 pour créer des plannings quotidiens.
- Comment programmer l'ESP8266 pour créer des plannings hebdomadaires.
- Comment programmer l'ESP8266 pour créer des plannings à une date spécifique.
Préparation du matériel
1 | × | ESP8266 NodeMCU | |
1 | × | Micro USB Cable | |
1 | × | Real-Time Clock DS3231 Module | |
1 | × | CR2032 battery | |
1 | × | Jumper Wires | |
1 | × | (Optional) 5V Power Adapter for ESP8266 | |
1 | × | (Optional) ESP8266 Screw Terminal Adapter |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos du module d'horloge en temps réel DS3231
ESP8266 possède certaines fonctions liées au temps, telles que millis() et micros(). Cependant, elles ne fournissent pas la date et l'heure (secondes, minutes, heures, jour, date, mois et année). Pour obtenir ces informations, nous devons utiliser un module d'Horloge Temps Réel (RTC), tel que DS3231 ou DS1370. Le module DS3231 est plus précis que le DS1370. Pour plus d'informations, veuillez consulter DS3231 vs DS1307.
Le brochage du module RTC
Le module d'horloge temps réel DS3231 dispose de 10 broches :
- 32K : Cette broche fournit une horloge de référence stable et précise.
- SQW : Cette broche produit une onde carrée à 1Hz, 4kHz, 8kHz ou 32kHz et peut être gérée par programmation. Elle peut également être utilisée comme une interruption d'alarme dans diverses applications dépendantes du temps.
- SCL : Ceci est la broche d'horloge série pour l'interface I2C.
- SDA : Ceci est la broche de données série pour l'interface I2C.
- VCC : Cette broche alimente le module. Elle peut être entre 3,3V et 5,5V.
- GND : Ceci est la broche de masse.
Pour un fonctionnement régulier, quatre broches sont nécessaires : VCC, GND, SDA et SCL.
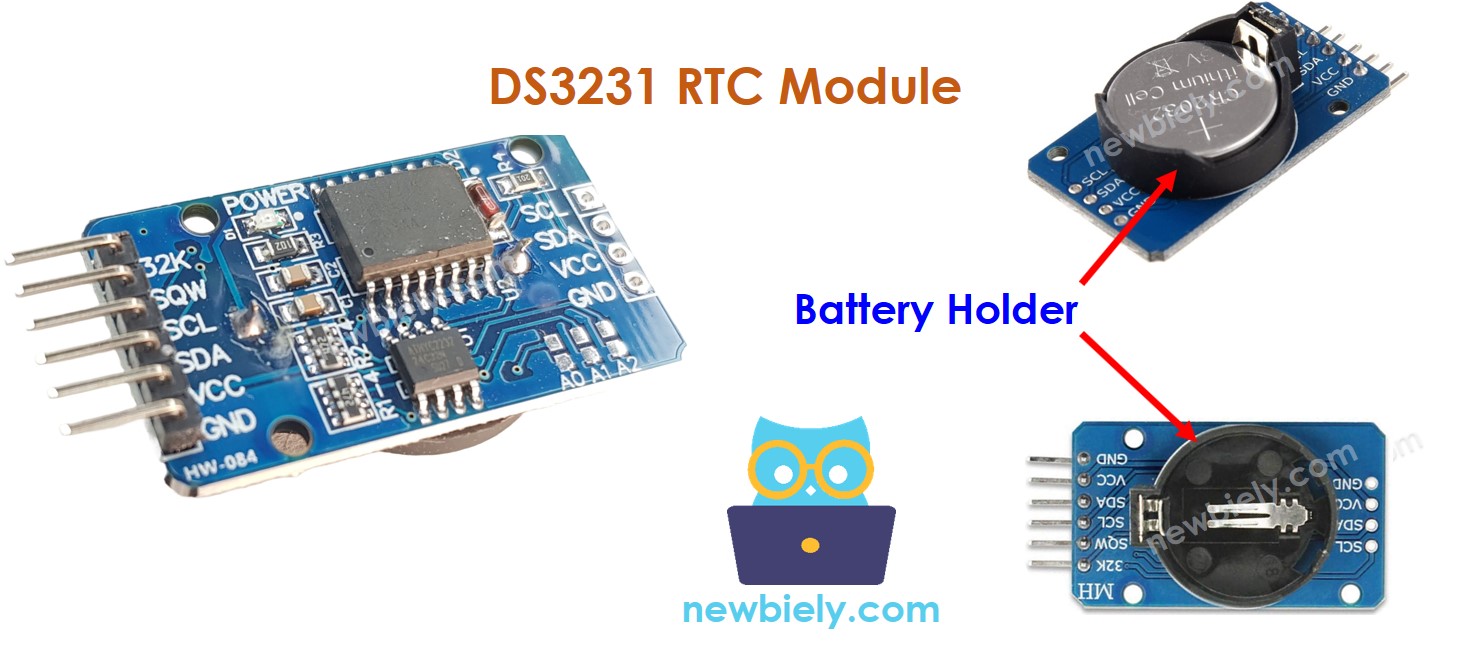
Le module DS3231 est équipé d'un support de batterie qui, lorsqu'une batterie CR2032 y est insérée, permet de maintenir l'heure à jour même lorsque l'alimentation principale est coupée. Sans la batterie, les informations horaires seront perdues si l'alimentation principale est déconnectée et devront être réinitialisées.
Diagramme de câblage
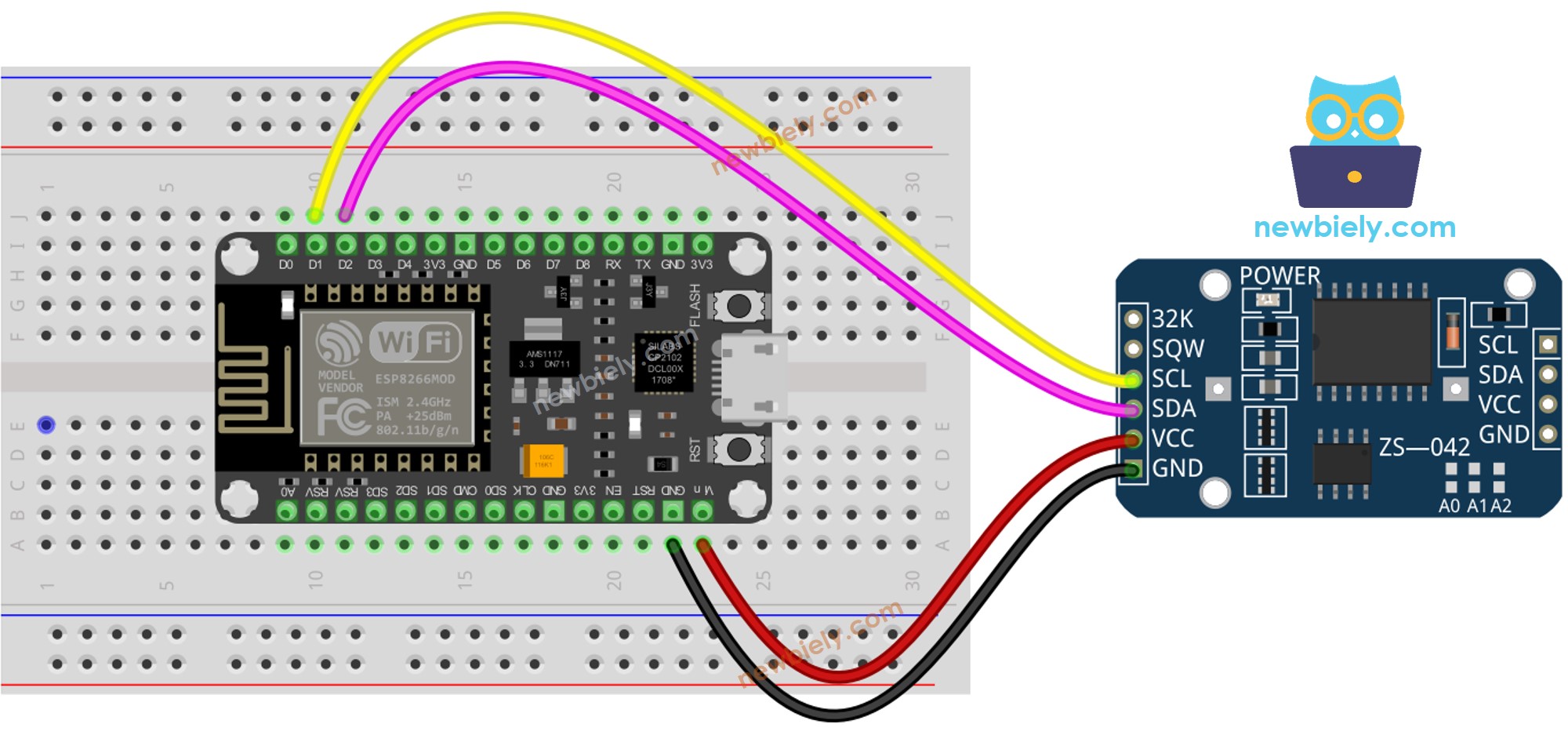
This image is created using Fritzing. Click to enlarge image
Voir plus dans l'agencement des broches de l'ESP8266 et comment alimenter l'ESP8266 et d'autres composants.
ESP8266 - Module RTC DS3231
DS3231 RTC Module | ESP8266 |
---|---|
Vin | 3.3V |
GND | GND |
SDA | GPIO4 |
SCL | GPIO5 |
Comment programmer le module RTC DS3231
- Incluez la bibliothèque.
- Créer un objet RTC :
- Commencez le processus de configuration du RTC.
- Réglez l'horloge RTC sur la date et l'heure de l'ordinateur lorsque le sketch a été compilé pour la première fois.
- Récupère les données de date et d'heure du module RTC.
Code ESP8266 - Comment obtenir des données et l'heure
Étapes rapides
Pour commencer avec l'ESP8266 sur Arduino IDE, suivez ces étapes :
- Consultez le tutoriel comment configurer l'environnement pour ESP8266 sur Arduino IDE si c'est votre première utilisation de l'ESP8266.
- Connectez les composants comme indiqué dans le schéma.
- Connectez la carte ESP8266 à votre ordinateur via un câble USB.
- Ouvrez l'Arduino IDE sur votre ordinateur.
- Choisissez la bonne carte ESP8266, comme (par exemple, NodeMCU 1.0 (Module ESP-12E)), et son port COM respectif.
- Cliquez sur l'icône Libraries dans la barre latérale gauche de l'Arduino IDE.
- Recherchez “RTClib” et trouvez la bibliothèque RTC de Adafruit.
- Appuyez sur le bouton Install pour installer la bibliothèque RTC.
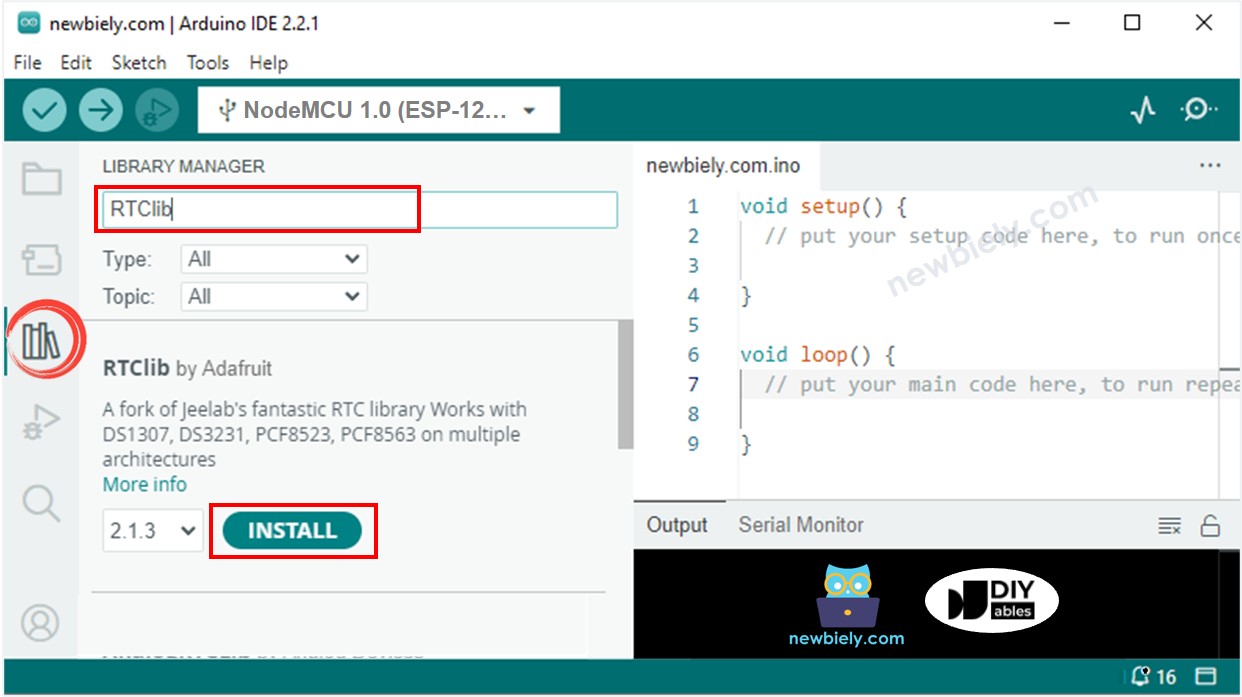
- Copiez le code et ouvrez-le avec l'IDE Arduino.
- Cliquez sur le bouton Upload dans l'IDE pour l'envoyer à l'ESP8266.
- Ouvrez le moniteur série.
- Vérifiez la sortie sur le moniteur série.