ESP8266 - Requête HTTP
Ce tutoriel vous explique comment utiliser l'ESP8266 pour effectuer une requête HTTP vers un serveur web, une API ou un service web. En détail, vous apprendrez :
- Comment utiliser ESP8266 pour effectuer une requête HTTP (GET et POST)
- Comment inclure les données du capteur dans une requête HTTP
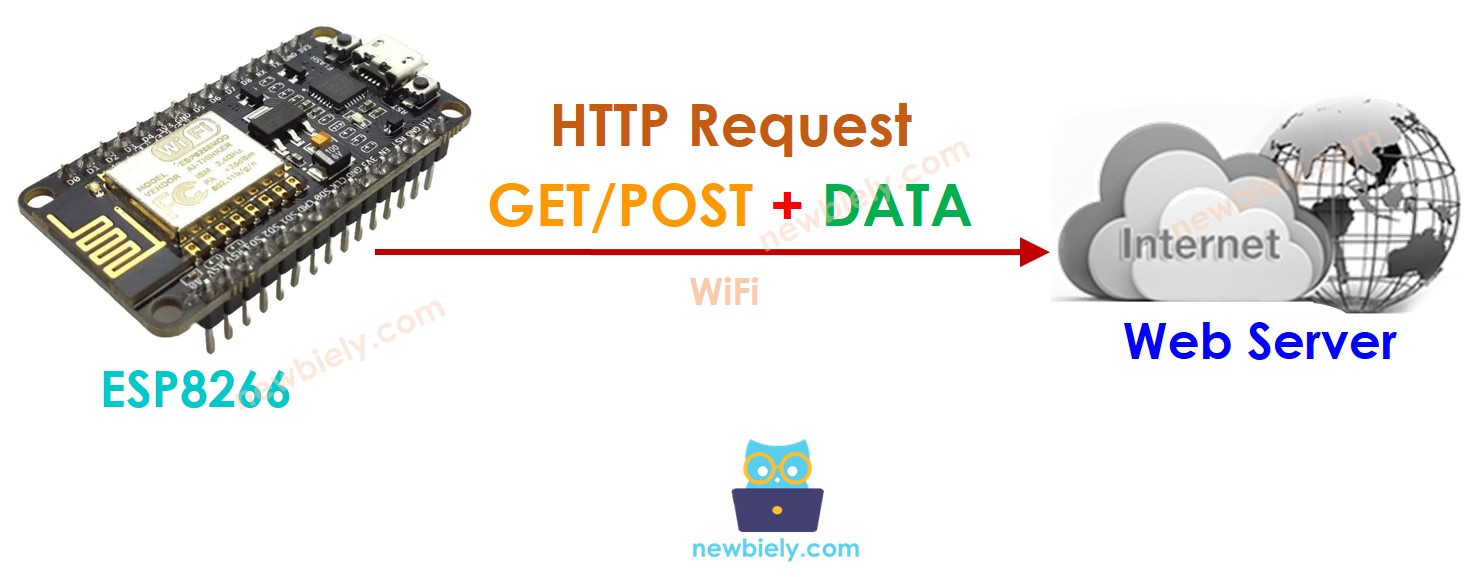
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Concepts de base du client Web et du serveur Web
Il existe quelques concepts de base du web tels que : adresse web (URL), nom d'hôte, chemin d'accès, chaîne de requête, requête HTTP... Vous pouvez en apprendre davantage à leur sujet dans le tutoriel HTTP
Comment effectuer une requête HTTP
- Inclure les bibliothèques
- Déclarez le SSID et le mot de passe WiFi
- Déclarez nom d'hôte, chemin d'accès, chaîne de requête
- Déclarer un objet client HTTP
- Si connecté au serveur, envoyez une requête HTTP. Par exemple, HTTP GET.
- Lisez les données de réponse du serveur web.
Comment inclure des données dans une requête HTTP
Nous pouvons envoyer des données au serveur web en incluant des données dans la requête HTTP. Le format des données dépend de la méthode de requête HTTP :
- Pour une requête HTTP GET
- Les données peuvent être envoyées uniquement dans la chaîne de requête de l'URI.
- Requête HTTP POST
- Les données peuvent être envoyées NON SEULEMENT sous forme de chaîne de requête MAIS AUSSI dans tout autre format tel que Json, XML, image...
- Les données sont placées dans le corps de la requête HTTP.
- Créer une chaîne de requête
- GET HTTP : ajoutez chaîne de requête au chemin d'accès
- POST HTTP : placez la chaîne de requête dans le corps HTTP.
- Pour les méthodes GET et POST, lisez les données de réponse du serveur web.
Apprenons à envoyer des données au format de chaîne de requête pour les méthodes HTTP GET et POST.
Code complet ESP8266 pour effectuer une requête HTTP
Le code ESP8266 complet pour effectuer une requête HTTP GET/POST est le suivant.