ESP8266 - Compteur Bouton - OLED
Ce tutoriel vous explique comment utiliser l'ESP8266 et un bouton pour compter les événements de pression, puis afficher la valeur sur un OLED. En détail :
- L'ESP8266 compte combien de fois un bouton est pressé.
- L'ESP8266 affiche le nombre de pressions sur un OLED.
- L'ESP8266 aligne automatiquement le nombre de pressions au centre de l'écran OLED, horizontalement et verticalement.
Dans ce tutoriel, nous allons déparasiter un bouton sans utiliser la fonction delay(). Pour plus d'informations sur la nécessité du déparasitage, veuillez consulter Pourquoi avons-nous besoin de déparasiter ?
Vous pouvez adapter cela pour fonctionner avec différents capteurs au lieu du bouton.
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos de l'OLED et du bouton
Si vous n'êtes pas familier avec les OLED et les boutons (brochage, fonctionnalités, programmation...), les tutoriels suivants peuvent vous aider :
Diagramme de câblage
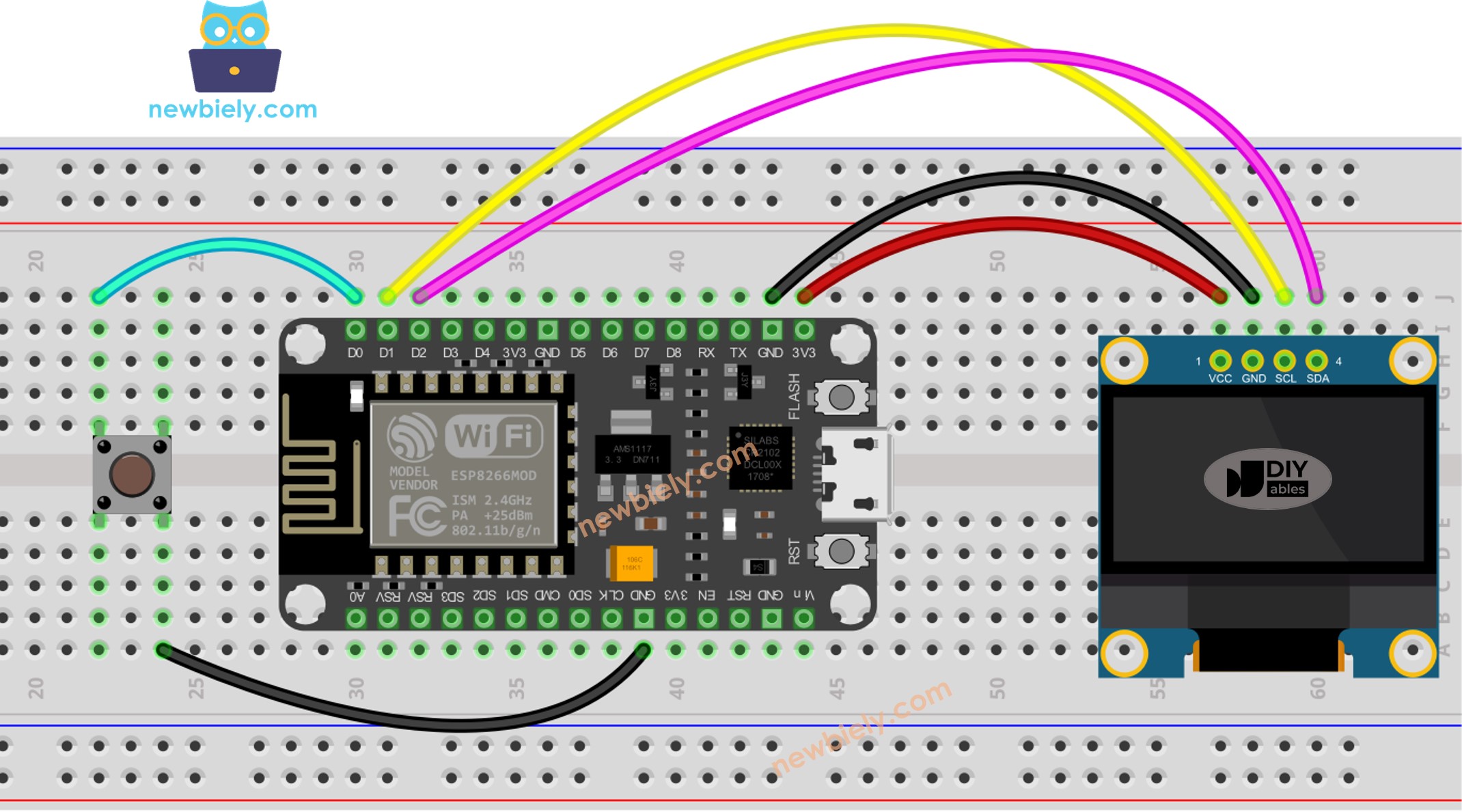
This image is created using Fritzing. Click to enlarge image
Voir plus dans Brochage ESP8266. et Comment alimenter l'ESP8266..
Code ESP8266 - affichage du comptage des boutons sur OLED
Étapes rapides
Pour commencer avec l'ESP8266 sur Arduino IDE, suivez ces étapes :
- Consultez le tutoriel Installation du logiciel ESP8266. si c'est la première fois que vous utilisez ESP8266.
- Câblez les composants comme indiqué sur le schéma.
- Connectez la carte ESP8266 à votre ordinateur à l'aide d'un câble USB.
- Ouvrez Arduino IDE sur votre ordinateur.
- Choisissez la bonne carte ESP8266, comme (par exemple NodeMCU 1.0 (Module ESP-12E)), et son port COM respectif.
- Cliquez sur l'icône Libraries dans la barre de gauche de l'Arduino IDE.
- Recherchez “ezButton”, puis localisez la bibliothèque de boutons fournie par ArduinoGetStarted.
- Appuyez sur le bouton Install pour installer la bibliothèque ezButton.
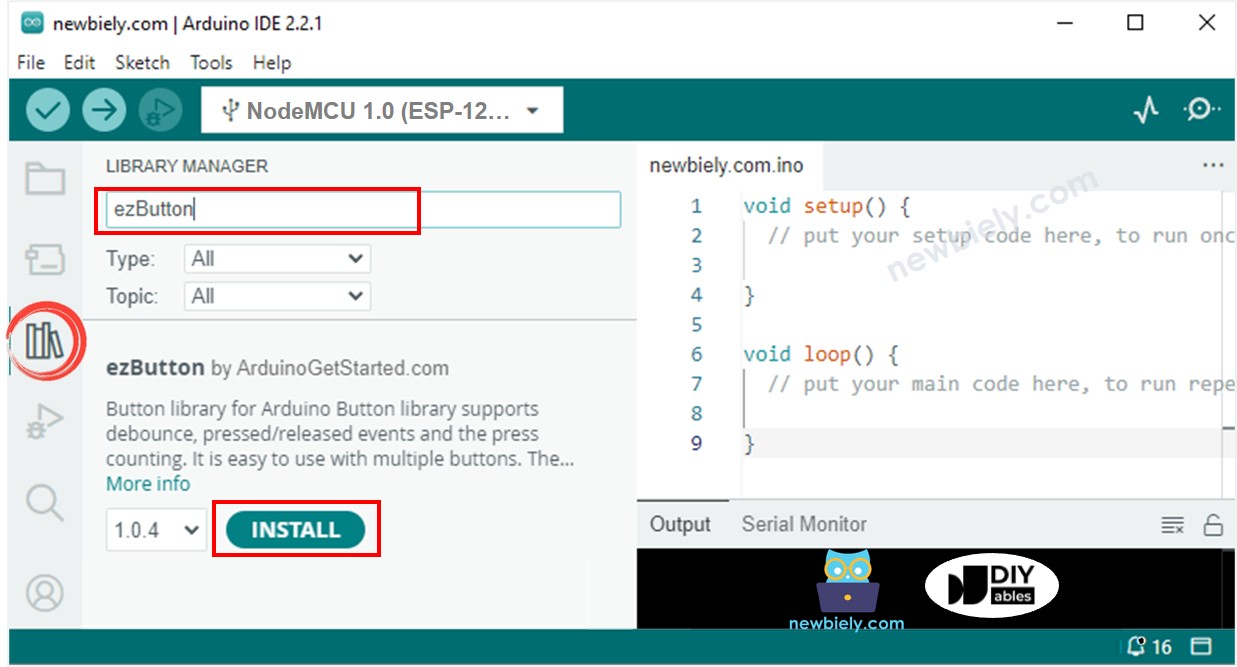
- Recherchez “SSD1306” et localisez la bibliothèque SSD1306 créée par Adafruit.
- Cliquez sur le bouton Install pour ajouter la bibliothèque.
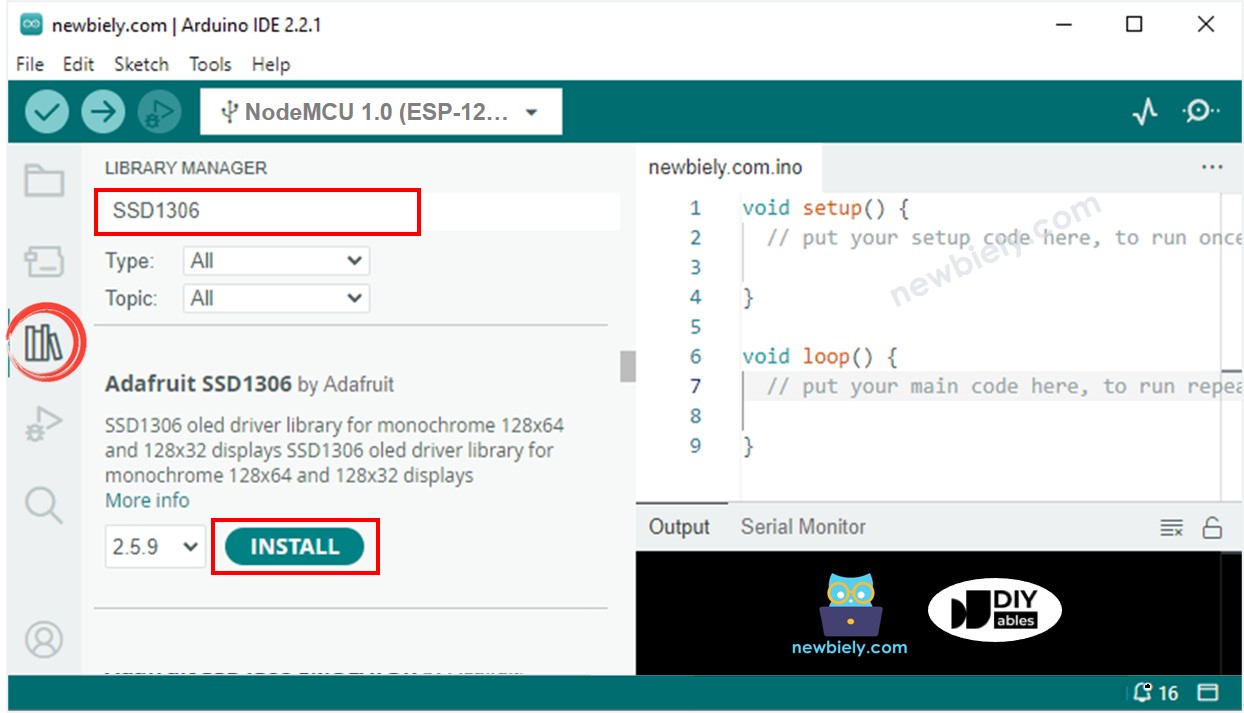
- Vous serez invité à installer des dépendances de bibliothèque supplémentaires.
- Pour les installer toutes, cliquez sur le bouton Install All.
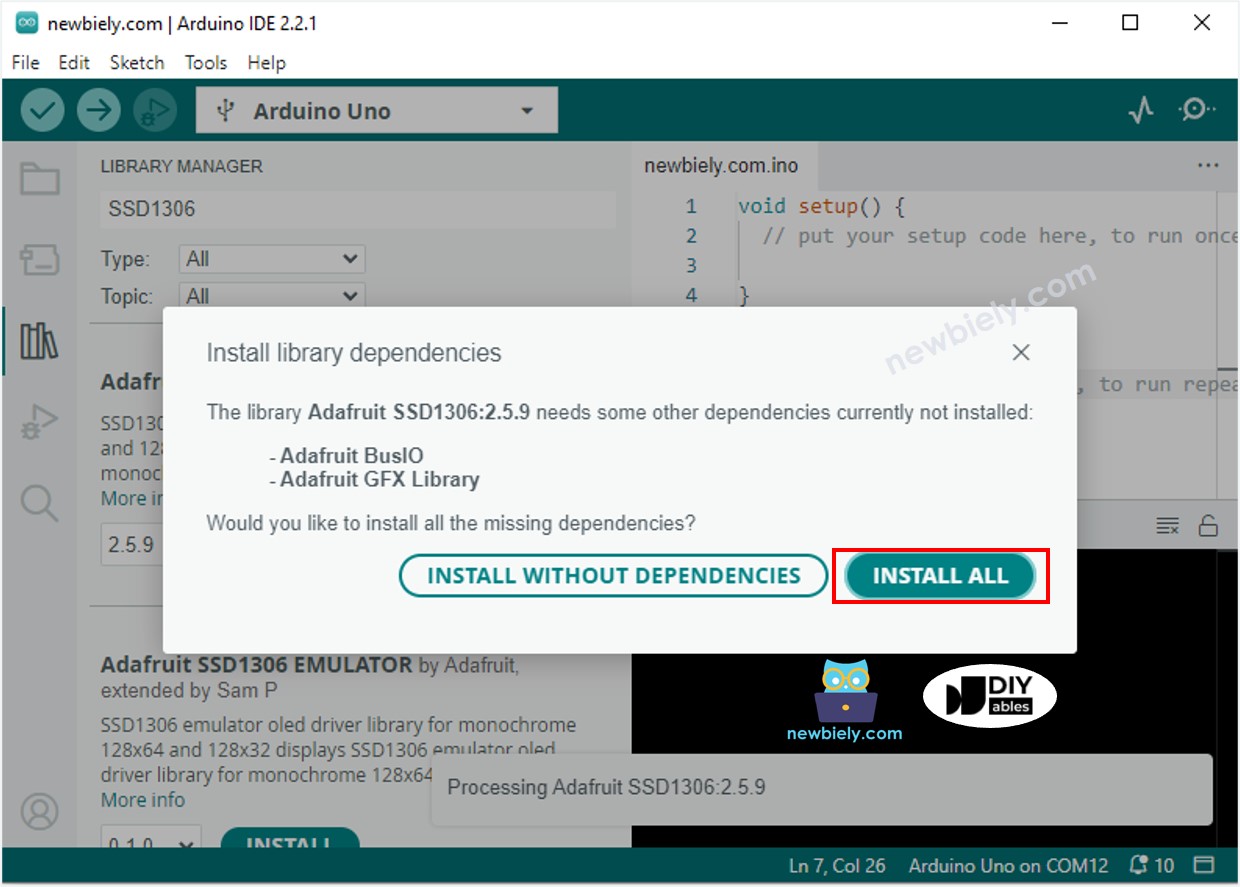
- Copiez le code et ouvrez-le avec l'IDE Arduino.
- Cliquez sur le bouton Upload de l'IDE Arduino pour compiler et téléverser le code vers l'ESP8266.
- Appuyez plusieurs fois sur le bouton.
- Observez le nombre qui change sur l'OLED.
Le code ci-dessus indique le nombre de pressions sur le bouton dans le coin supérieur gauche. Faisons un changement pour le centrer !
Code ESP8266 - Alignement vertical et horizontal au centre sur OLED
※ Note:
Ce code permet de centrer le texte horizontalement et verticalement sur un affichage OLED. Pour plus d'informations, veuillez consulter Comment centrer verticalement/horizontalement sur OLED.