ESP8266 - Potentiomètre Buzzer Piézo
Ce tutoriel vous explique comment utiliser l'ESP8266 et un potentiomètre pour contrôler un buzzer piézo. En détail :
- L'ESP8266 détermine si la valeur analogique du potentiomètre est au-dessus ou en dessous d'un seuil, et émet un son en conséquence.
- L'ESP8266 détermine si la tension de sortie du potentiomètre est au-dessus ou en dessous d'un seuil, et émet un son en conséquence.
- Si la tension de sortie du potentiomètre est supérieure à un seuil, l'ESP8266 peut également jouer une mélodie d'une chanson.
Préparation du matériel
1 | × | ESP8266 NodeMCU | |
1 | × | Micro USB Cable | |
1 | × | Potentiometer | |
1 | × | Piezo Buzzer | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Optional) 5V Power Adapter for ESP8266 | |
1 | × | (Optional) ESP8266 Screw Terminal Adapter |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos du buzzer piézoélectrique et du potentiomètre
Si vous n'êtes pas familier avec le brochage, le fonctionnement et la programmation des buzzers piézoélectriques et des potentiomètres, les tutoriels suivants peuvent vous aider :
Diagramme de câblage
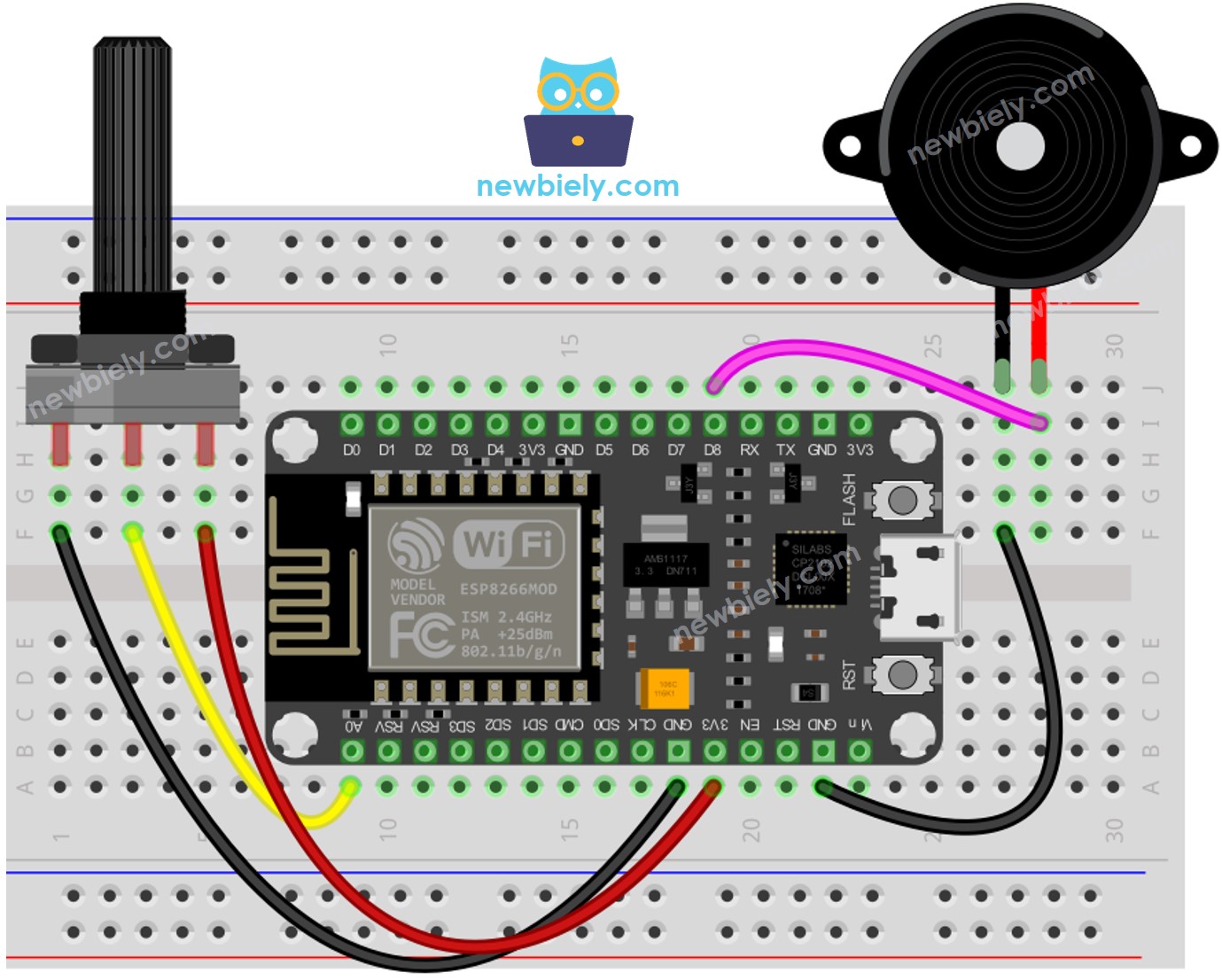
This image is created using Fritzing. Click to enlarge image
Voir plus dans l'agencement des broches de l'ESP8266 et comment alimenter l'ESP8266 et d'autres composants.
Code ESP8266 - Son Simple - Seuil Analogique
Étapes rapides
Pour commencer avec ESP8266 sur Arduino IDE, suivez ces étapes :
- Consultez le tutoriel comment configurer l'environnement pour ESP8266 sur Arduino IDE si c'est la première fois que vous utilisez un ESP8266.
- Câblez les composants comme indiqué dans le schéma.
- Connectez la carte ESP8266 à votre ordinateur à l'aide d'un câble USB.
- Ouvrez l'Arduino IDE sur votre ordinateur.
- Choisissez la bonne carte ESP8266, par exemple (e.g. NodeMCU 1.0 (Module ESP-12E)), et son port COM respectif.
- Connectez le NodeMCU ESP8266 à l'ordinateur à l'aide d'un câble USB.
- Ouvrez l'Arduino IDE, sélectionnez la bonne carte et le bon port.
- Copiez le code et ouvrez-le dans l'Arduino IDE.
- Cliquez sur le bouton Upload dans l'Arduino IDE pour envoyer le code à l'ESP8266.
- Tournez le potentiomètre.
- Écoutez le son émis par le buzzer piézo.
Explication du code
Découvrez l'explication détaillée ligne par ligne contenue dans les commentaires du code source !
Code ESP8266 - Son Simple - Seuil de Tension
La valeur analogique d'un potentiomètre est convertie en une valeur de tension. Ensuite, cette tension est comparée à un seuil de tension, qui activera le buzzer piézoélectrique si le seuil est dépassé.
Code ESP8266 - Mélodie - Seuil de Tension
Étapes rapides
- Câblez les composants comme indiqué dans le schéma.
- Connectez la carte ESP8266 à votre ordinateur à l'aide d'un câble USB.
- Ouvrez Arduino IDE sur votre ordinateur.
- Choisissez la bonne carte ESP8266, comme par exemple le NodeMCU 1.0 (Module ESP-12E), et son port COM respectif.
- Copiez le code et ouvrez-le avec l'Arduino IDE.
- Créez le fichier pitches.h sur Arduino IDE en :
- Cliquant soit sur le bouton juste en dessous de l'icône du moniteur série et choisissez Nouvel Onglet, soit utilisez les touches Ctrl+Shift+N.
- Donnez le nom de fichier pitches.h et cliquez sur le bouton OK
- Copiez le code ci-dessous et collez-le dans le fichier créé pitches.h.
- Cliquez sur le bouton Upload dans l'IDE Arduino pour compiler et téléverser le code vers l'ESP8266.
- Tournez le potentiomètre.
- Écoutez la mélodie du buzzer piézo.
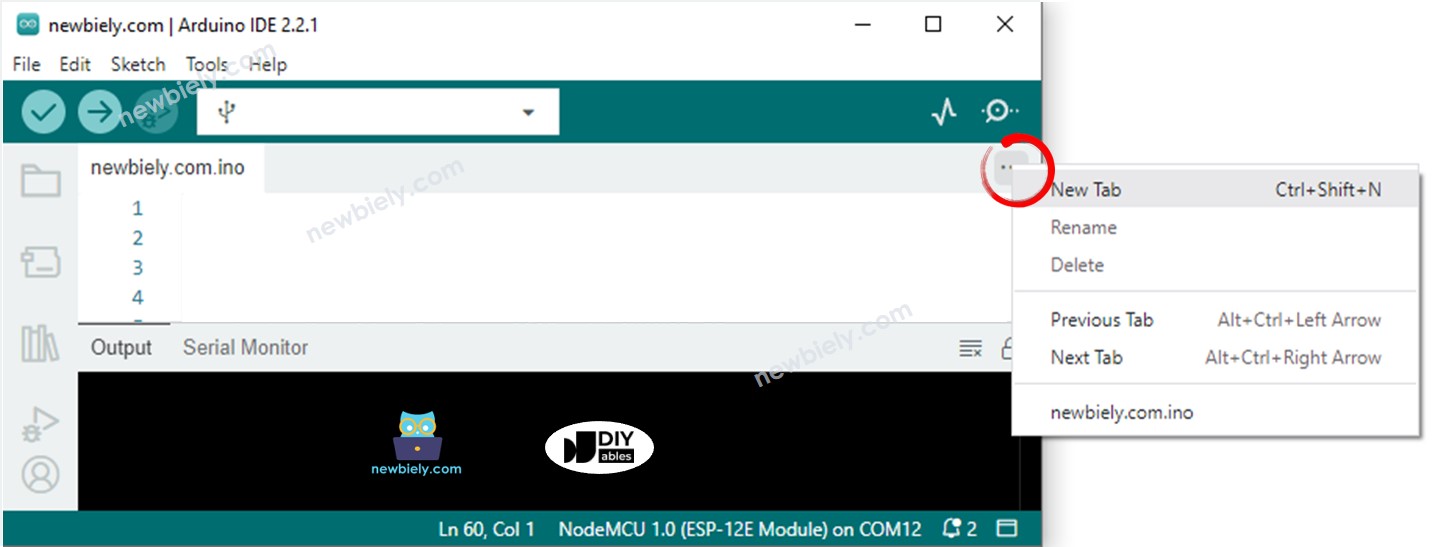
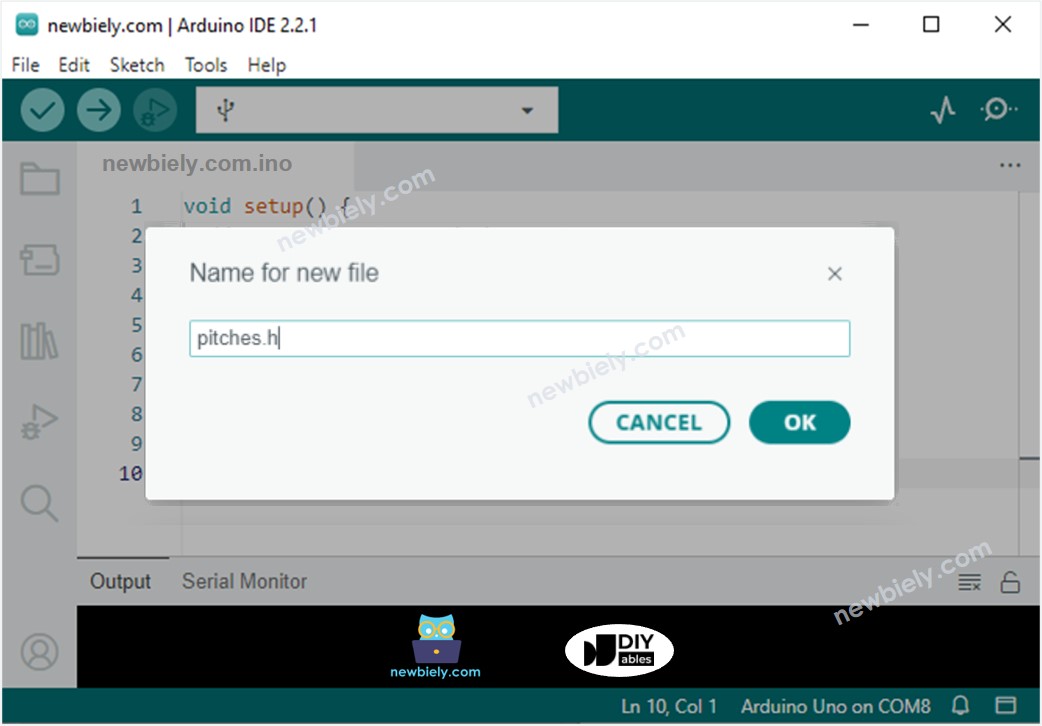
Explication du code
Découvrez l'explication ligne par ligne contenue dans les commentaires du code source !
※ NOTE THAT:
Le code ci-dessus utilise la fonction delay(). Cela a pour effet de bloquer l'exécution d'autres codes pendant la lecture d'une mélodie. Pour éviter cela, on peut utiliser la bibliothèque ezBuzzer à la place. Cette bibliothèque a été spécifiquement conçue pour permettre à un buzzer de bipper ou de jouer une mélodie sans bloquer les autres codes.