Raspberry Pi - Feu de circulation
Dans ce tutoriel, nous allons apprendre à utiliser Raspberry Pi pour contrôler le module de feu de circulation. En détail, nous allons apprendre :
- Comment connecter le module de feu de circulation au Raspberry Pi
- Comment programmer le Raspberry Pi pour contrôler le module de feu de circulation RVB
- Comment programmer le Raspberry Pi pour contrôler le module de feu de circulation RVB sans utiliser la fonction delay()
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos du module de feux de circulation
Brochage
Un module de feu de circulation comprend 4 broches :
- Broche GND : La broche de masse, connectez cette broche au GND du Raspberry Pi.
- Broche R : La broche pour contrôler la lumière rouge, connectez cette broche à une sortie numérique du Raspberry Pi.
- Broche Y : La broche pour contrôler la lumière jaune, connectez cette broche à une sortie numérique du Raspberry Pi.
- Broche G : La broche pour contrôler la lumière verte, connectez cette broche à une sortie numérique du Raspberry Pi.
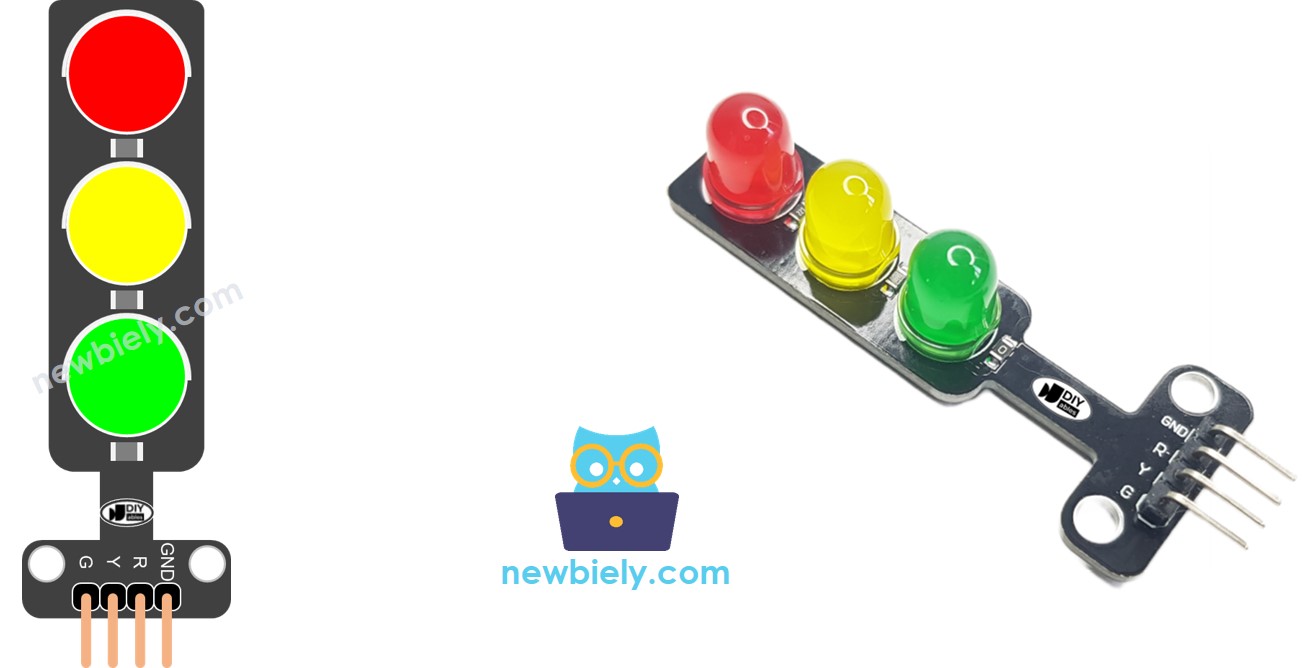
Comment ça marche
Diagramme de câblage
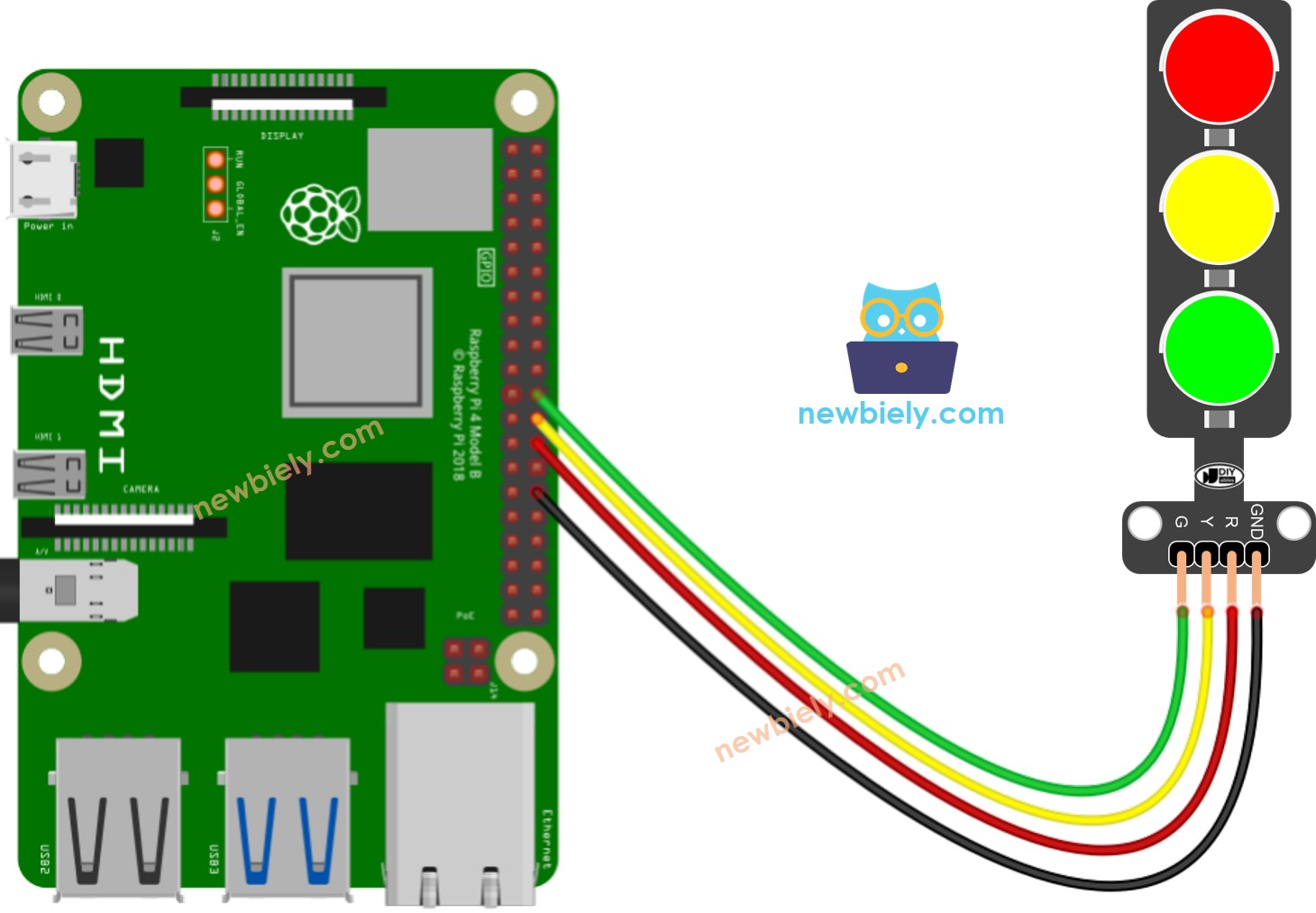
This image is created using Fritzing. Click to enlarge image
Pour simplifier et organiser votre câblage, nous vous recommandons d'utiliser un Screw Terminal Block Shield pour Raspberry Pi. Ce shield garantit des connexions plus sûres et plus faciles à gérer, comme illustré ci-dessous :
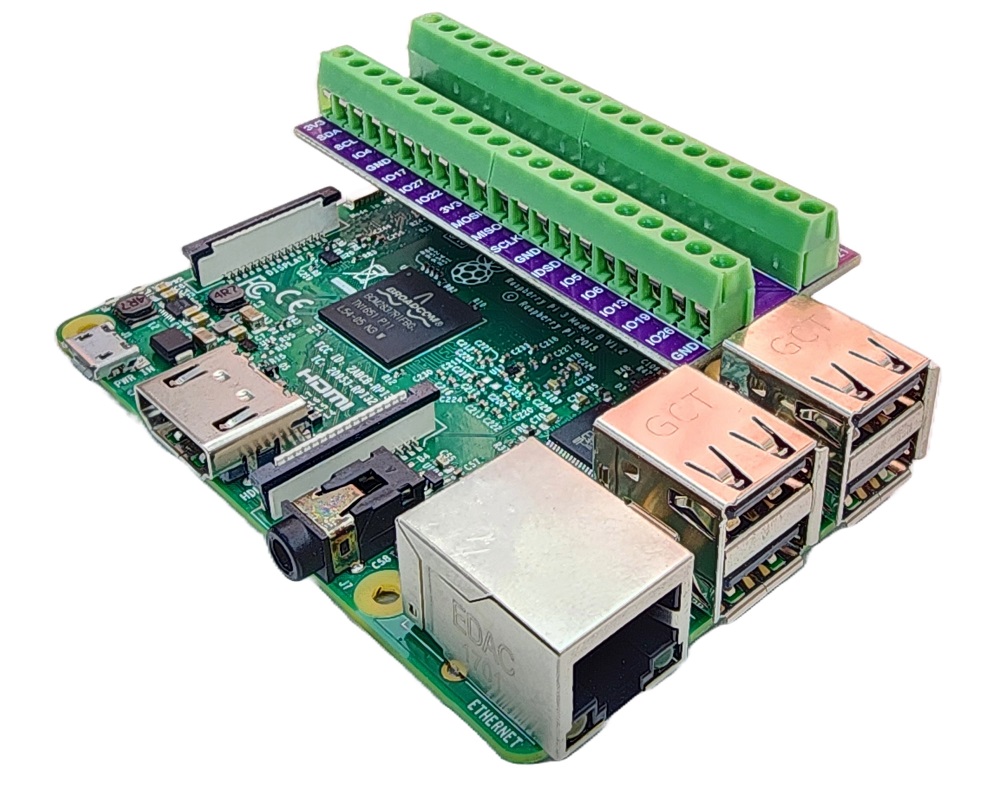
Code Raspberry Pi
Étapes rapides
- Assurez-vous d'avoir installé Raspbian ou tout autre système d'exploitation compatible avec Raspberry Pi sur votre Pi.
- Assurez-vous que votre Raspberry Pi est connecté au même réseau local que votre PC.
- Assurez-vous que votre Raspberry Pi est connecté à Internet si vous devez installer des bibliothèques.
- Si c'est la première fois que vous utilisez Raspberry Pi, consultez Installation du logiciel - Raspberry Pi..
- Connectez votre PC au Raspberry Pi via SSH en utilisant le client SSH intégré sur Linux et macOS ou PuTTY sur Windows. Consultez comment connecter votre PC au Raspberry Pi via SSH.
- Assurez-vous que vous avez installé la bibliothèque RPi.GPIO. Sinon, installez-la en utilisant la commande suivante :
- Créez un fichier de script Python traffic_light.py et ajoutez le code suivant :
- Enregistrez le fichier et exécutez le script Python en exécutant la commande suivante dans le terminal :
Le script s'exécute en boucle infinie continuellement jusqu'à ce que vous appuyiez sur Ctrl + C dans le terminal.
- Consultez le module de feu tricolore
Il est important de noter que le fonctionnement exact d'un feu de signalisation peut varier en fonction du design spécifique et de la technologie utilisés dans différentes régions et intersections. Les principes décrits ci-dessus fournissent une compréhension générale de la manière dont les feux de signalisation fonctionnent pour gérer le trafic et améliorer la sécurité sur les routes.
Le code ci-dessus démontre le contrôle individuel de la lumière. Maintenant, améliorons le code pour une meilleure optimisation.
Optimisation du Code du Raspberry Pi
- Améliorons le code en implémentant une fonction pour le contrôle de l'éclairage.
- Améliorons le code en utilisant une boucle for.
- Améliorons le code en utilisant la fonction millis() au lieu de delay().