Raspberry Pi - OLED
Ce didacticiel vous explique comment utiliser Raspberry Pi avec un écran OLED. En détail, nous apprendrons :
- Comment connecter un écran OLED avec un Raspberry Pi.
- Comment programmer le Raspberry Pi pour afficher du texte et des chiffres sur l'OLED.
- Comment programmer le Raspberry Pi pour aligner le texte et les chiffres verticalement et horizontalement au centre sur l'OLED.
- Comment programmer le Raspberry Pi pour dessiner sur l'OLED.
- Comment programmer le Raspberry Pi pour afficher une image sur l'OLED.
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos de l'affichage OLED
Il existe différents types d'écrans OLED disponibles. L'OLED le plus couramment utilisé avec Raspberry Pi est l'écran SSD1306 I2C OLED 128x64 et 128x32.
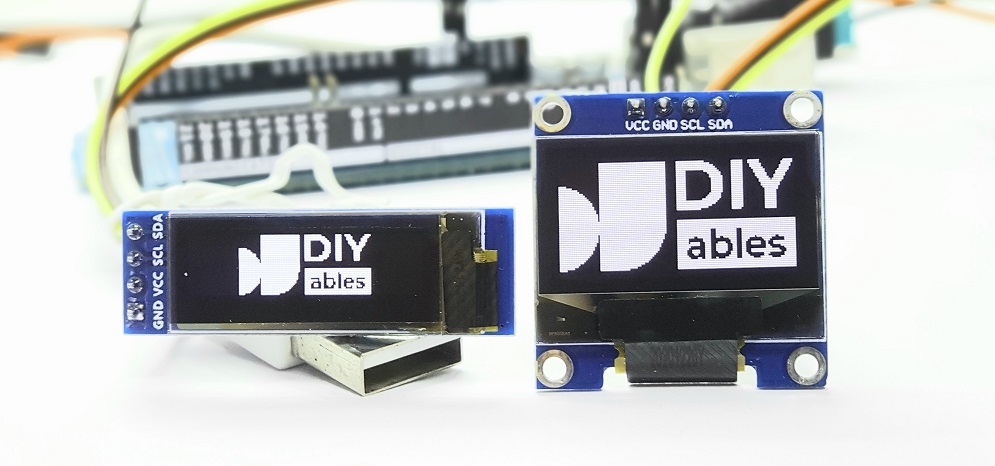
I2C OLED Afficheur Brochage
- Broche GND : doit être connectée à la masse du Raspberry Pi.
- Broche VCC : est l'alimentation de l'écran qui doit être connectée à la broche 5 volts du Raspberry Pi.
- Broche SCL : est une broche d'horloge série pour l'interface I2C.
- Broche SDA : est une broche de données séries pour l'interface I2C.
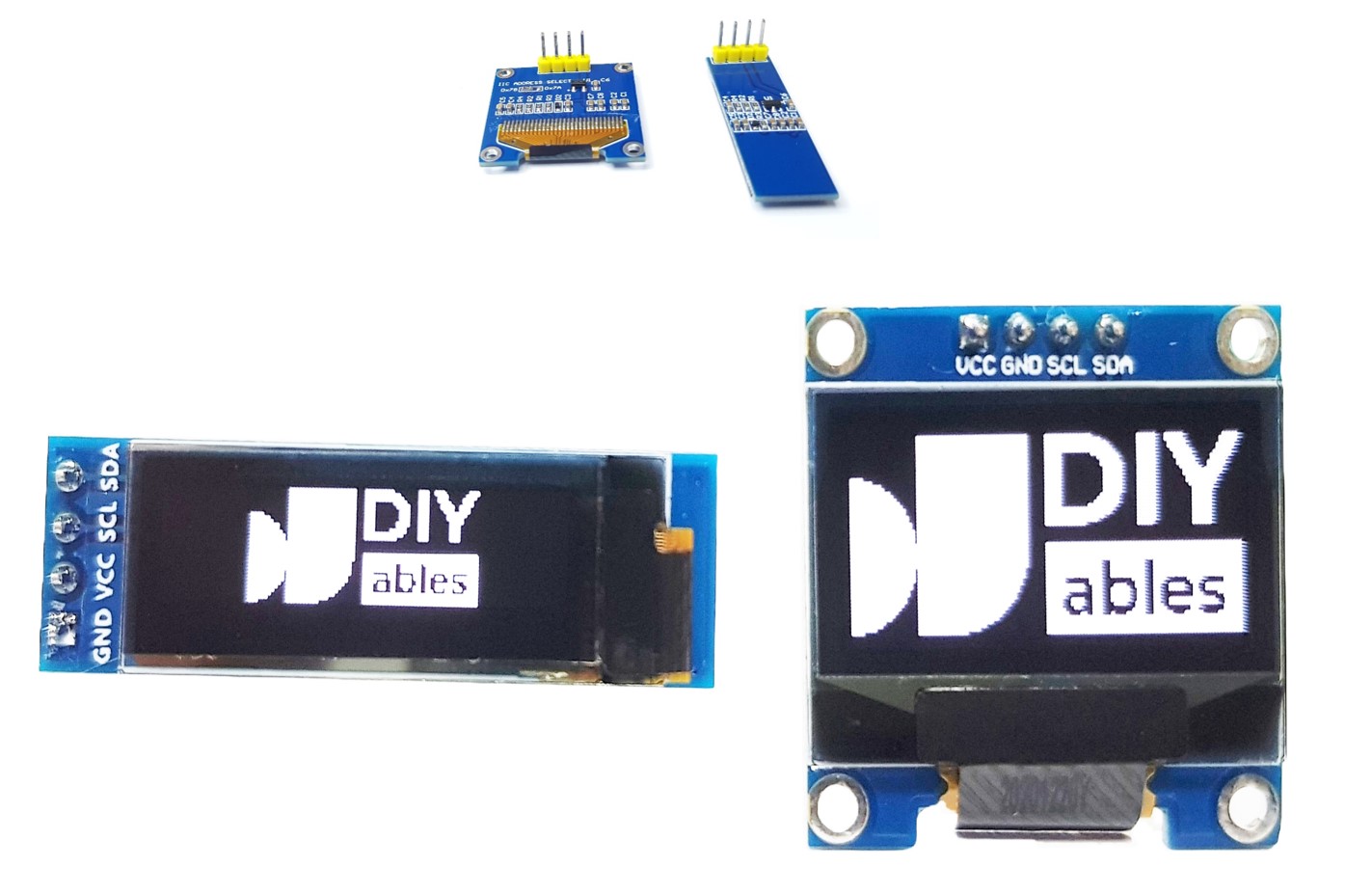
※ Note:
- Les broches d'un module OLED peuvent être disposées différemment selon le fabricant et le type de module. Il est IMPÉRATIF de toujours se référer aux étiquettes imprimées sur le module OLED. Faites très attention !
- Ce tutoriel utilise un écran OLED qui utilise le pilote I2C SSD1306. Nous l'avons testé avec l'écran OLED de DIYables et il fonctionne parfaitement.
Diagramme de câblage
- Schéma de câblage entre Raspberry Pi et OLED 128x64
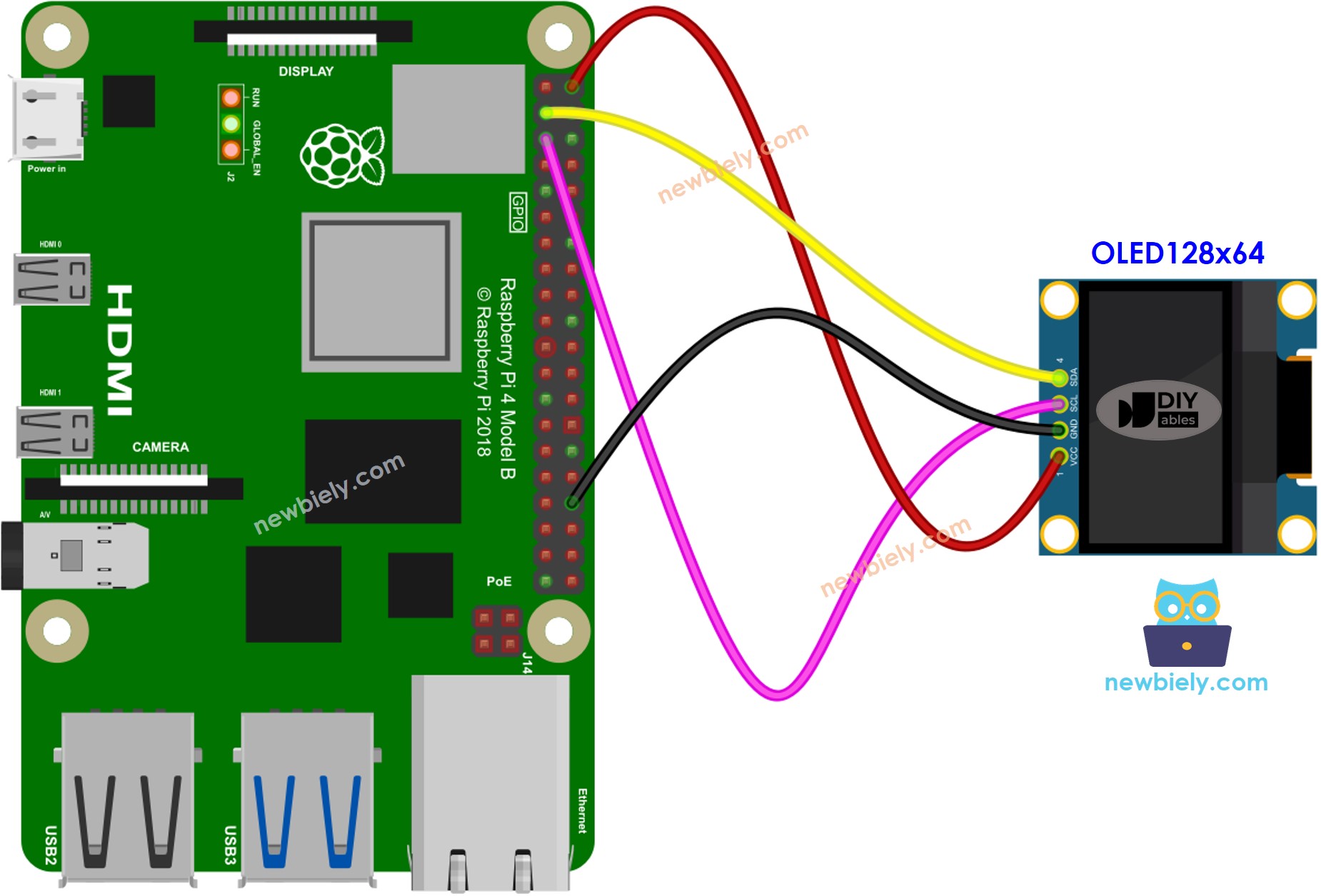
This image is created using Fritzing. Click to enlarge image
- Schéma de câblage entre Raspberry Pi et OLED 128x32
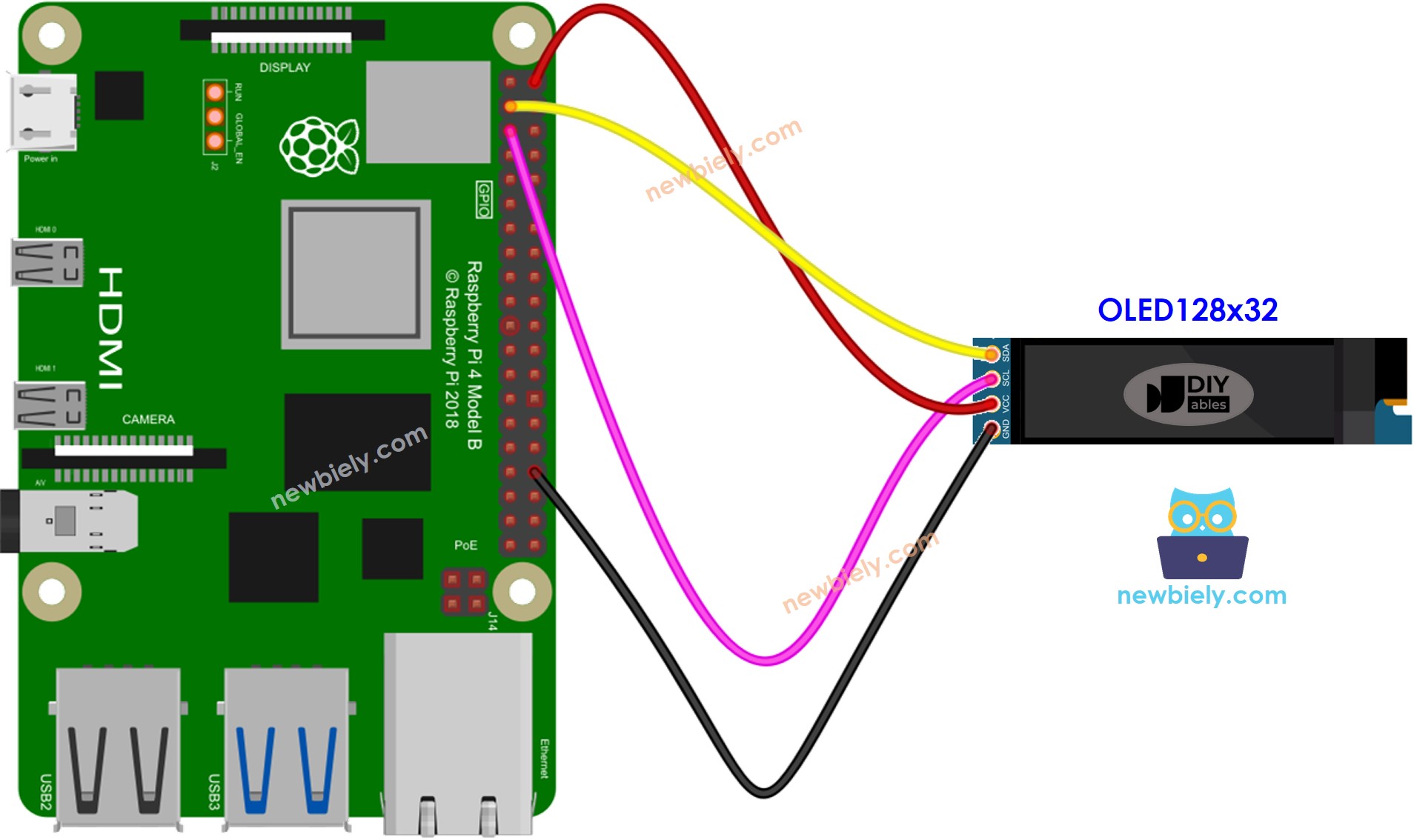
This image is created using Fritzing. Click to enlarge image
Pour simplifier et organiser votre câblage, nous vous recommandons d'utiliser un Screw Terminal Block Shield pour Raspberry Pi. Ce shield garantit des connexions plus sûres et plus faciles à gérer, comme illustré ci-dessous :
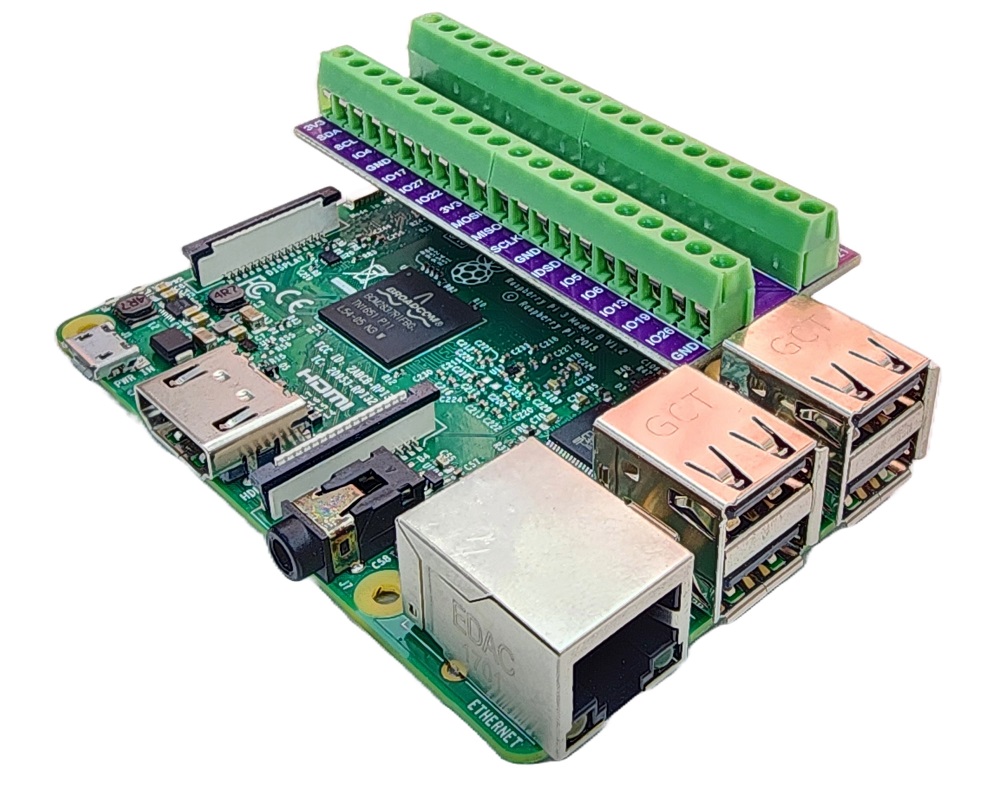
Le tableau de câblage entre le Raspberry Pi et l'écran OLED :
OLED Module | Raspberry Pi |
---|---|
Vin | 5V |
GND | GND |
SDA | GPIO2 (pin 3) |
SCL | GPIO3 (pin 5) |
Code Raspberry Pi - Afficher du texte sur OLED
Étapes rapides
- Assurez-vous d'avoir Raspbian ou tout autre système d'exploitation compatible avec Raspberry Pi installé sur votre Pi.
- Assurez-vous que votre Raspberry Pi est connecté au même réseau local que votre PC.
- Assurez-vous que votre Raspberry Pi est connecté à Internet si vous avez besoin d'installer certaines bibliothèques.
- Si c'est la première fois que vous utilisez Raspberry Pi, voyez Installation du logiciel - Raspberry Pi.
- Connectez votre PC au Raspberry Pi via SSH en utilisant le client SSH intégré sur Linux et macOS ou PuTTY sur Windows. Voir comment connecter votre PC au Raspberry Pi via SSH.
- Assurez-vous que vous avez la bibliothèque RPi.GPIO installée. Sinon, installez-la en utilisant la commande suivante :
- Avant d'utiliser l'écran OLED avec un Raspberry Pi, nous devons activer l'interface I2C sur le Raspberry Pi. Voir Comment activer l'interface I2C sur Raspberry Pi
- Installez la bibliothèque OLED en exécutant la commande suivante :
- Créez un fichier de script Python oled.py et ajoutez le code suivant :
- Enregistrez le fichier et exécutez le script Python en entrant la commande suivante dans le terminal :
- Vérifiez l'affichage OLED.
Le script s'exécute en boucle infinie jusqu'à ce que vous appuyiez sur Ctrl + C dans le terminal.
Code Raspberry Pi – Afficher une image
Pour afficher une image sur OLED, nous devons d'abord la convertir (quel que soit le format) en un tableau bitmap. Cela peut être fait en utilisant cet outil en ligne. Veuillez vous référer à l'image ci-dessous pour les instructions sur la façon de procéder. J'ai déjà converti l'icône Raspberry Pi en un tableau bitmap.
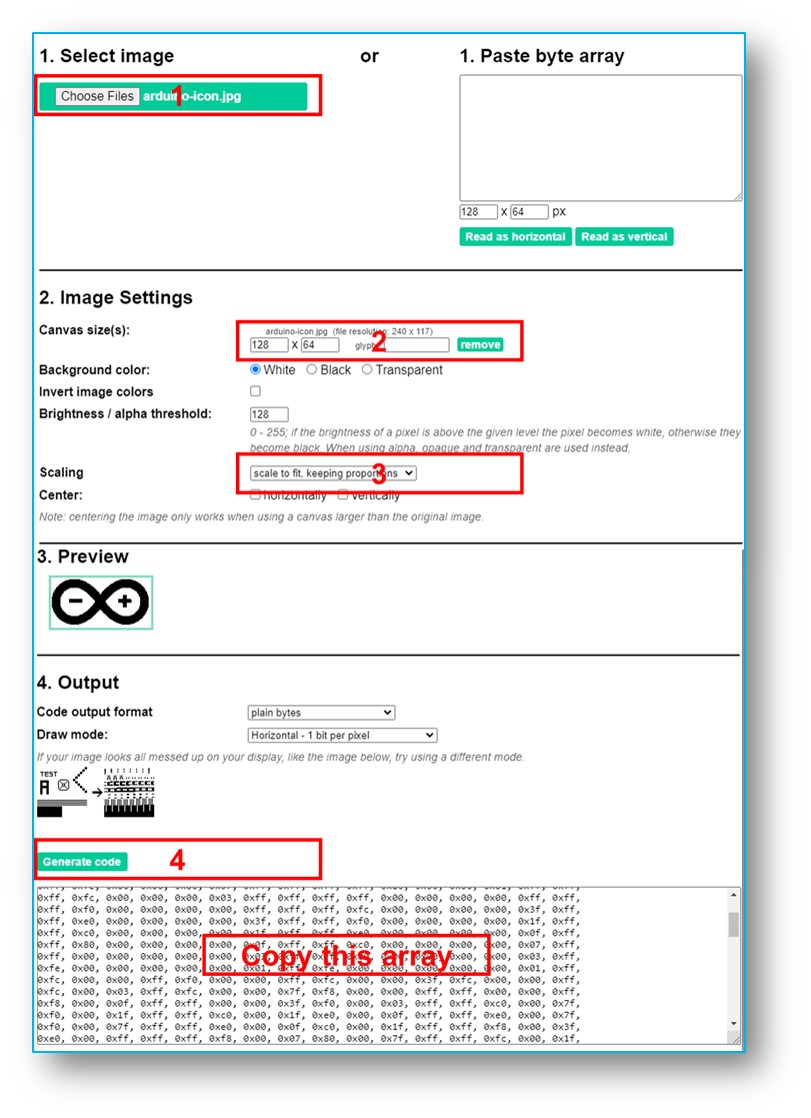
Une fois la conversion terminée, prenez le code du tableau et remplacez le code du tableau ArduinoIcon existant dans l'extrait suivant.
※ Note:
- La taille de l'image ne doit pas dépasser la taille de l'écran.
- Si vous souhaitez utiliser le code pour un OLED 128x32, vous devez redimensionner l'image et modifier les paramètres de largeur et de hauteur dans la fonction oled.drawBitmap();.