Raspberry Pi - Bouton - Relais
Ce tutoriel vous apprend comment utiliser le Raspberry Pi et un bouton pour contrôler le relais. En connectant le relais à une serrure à solénoïde, une ampoule, une bande LED, un moteur ou un actionneur..., nous pouvons utiliser un bouton pour les contrôler. Nous apprendrons deux applications différentes :
Application 1 - L'état du relais est synchronisé avec l'état du bouton. En détail :
- Le Raspberry Pi active le relais lorsque le bouton est pressé.
- Le Raspberry Pi désactive le relais lorsque le bouton n'est pas pressé.
Application 2 - L'état du relais est basculé chaque fois que le bouton est pressé. Plus précisément :
- Si le Raspberry Pi détecte que le bouton a été pressé (passant d'un état HAUT à un état BAS), il allumera le relais s'il est actuellement ÉTEINT, ou éteindra le relais s'il est actuellement ALLUMÉ.
- Relâcher le bouton n'affecte pas l'état du relais.
Dans l'Application 2, nous devons désamorcer le bouton pour nous assurer qu'il fonctionne correctement. Nous verrons pourquoi c'est important en comparant le comportement du relais lorsque nous utilisons le code Raspberry Pi avec et sans désamorçage du bouton.
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Diagramme de câblage
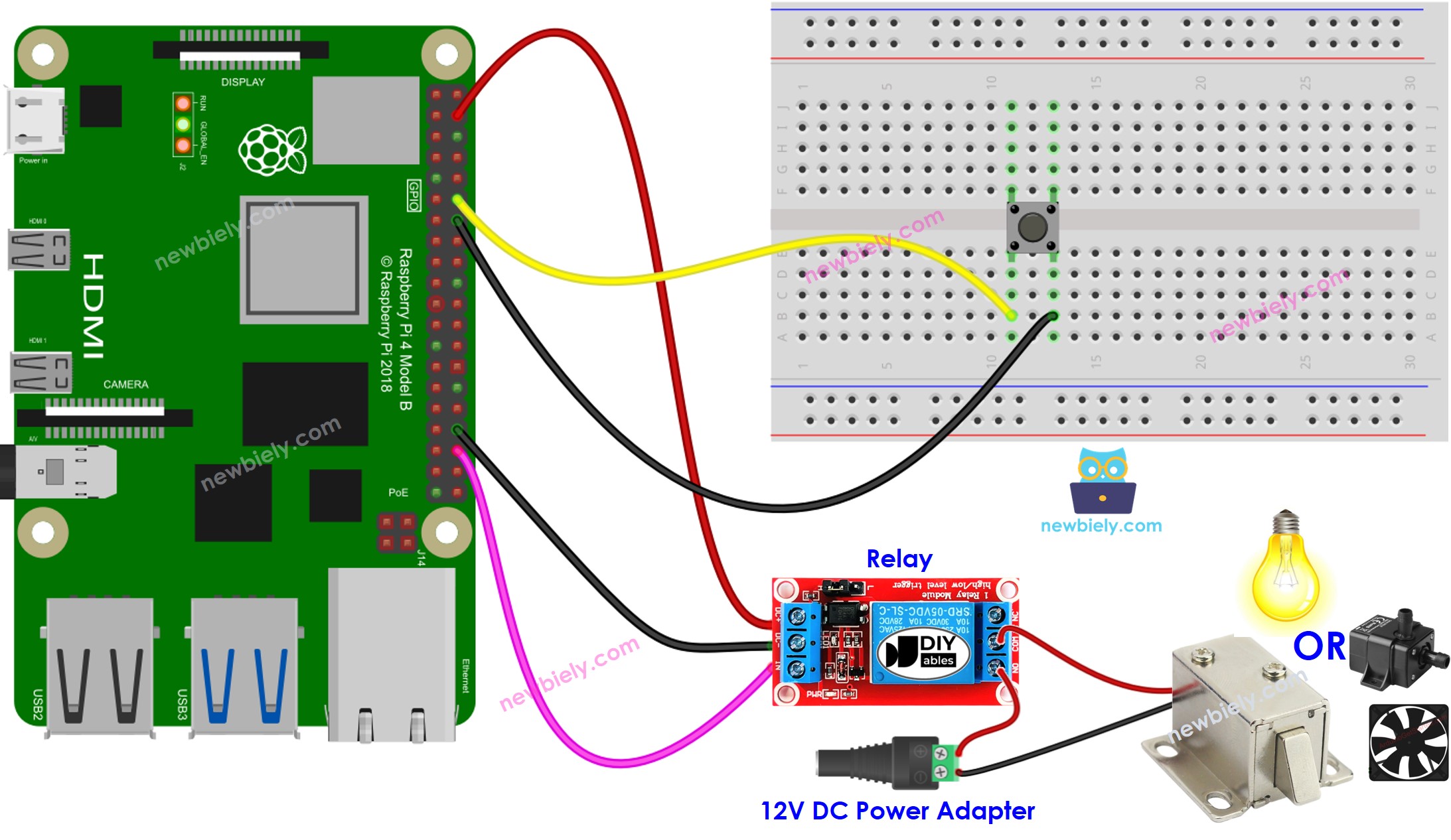
This image is created using Fritzing. Click to enlarge image
Pour simplifier et organiser votre câblage, nous vous recommandons d'utiliser un Screw Terminal Block Shield pour Raspberry Pi. Ce shield garantit des connexions plus sûres et plus faciles à gérer, comme illustré ci-dessous :
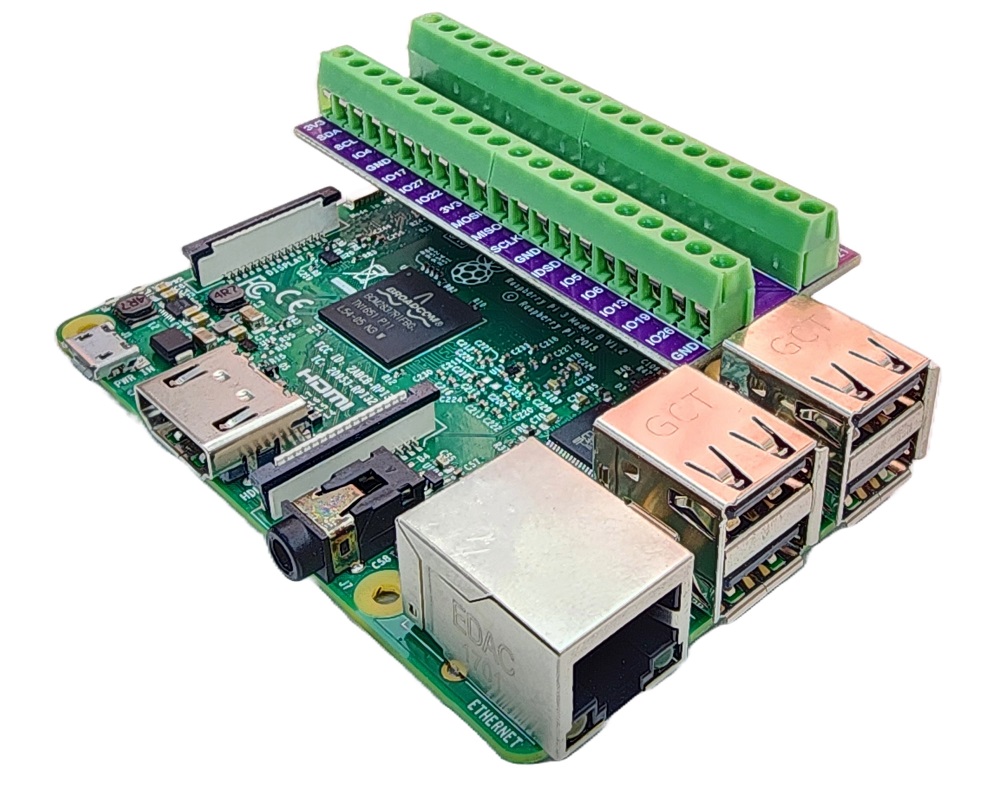
Application 1 - L'état du relais est synchronisé avec l'état du bouton
Étapes rapides
- Assurez-vous d'avoir Raspbian ou tout autre système d'exploitation compatible Raspberry Pi installé sur votre Pi.
- Assurez-vous que votre Raspberry Pi est connecté au même réseau local que votre PC.
- Assurez-vous que votre Raspberry Pi est connecté à Internet si vous avez besoin d'installer des bibliothèques.
- Si c'est la première fois que vous utilisez Raspberry Pi, consultez Installation du logiciel - Raspberry Pi..
- Connectez votre PC au Raspberry Pi via SSH en utilisant le client SSH intégré sur Linux et macOS ou PuTTY sur Windows. Consultez comment connecter votre PC au Raspberry Pi via SSH.
- Assurez-vous que vous avez la bibliothèque RPi.GPIO installée. Si ce n'est pas le cas, installez-la en utilisant la commande suivante :
- Créez un fichier de script Python button_relay.py et ajoutez le code suivant :
- Enregistrez le fichier et exécutez le script Python en tapant la commande suivante dans le terminal :
- Appuyez sur le bouton et maintenez-le enfoncé pendant quelques secondes.
- Observez le changement dans l'état du relais.
Vous verrez que l'état du relais est synchronisé avec l'état du bouton.
Le script s'exécute en boucle infinie jusqu'à ce que vous appuyiez sur Ctrl + C dans le terminal.
Explication du code
Consultez l'explication ligne par ligne contenue dans les commentaires du code source !
Application 2 - Le bouton active/désactive le relais
Étapes rapides
- Créez un fichier de script Python button_toggle_relay.py et ajoutez le code suivant :
- Enregistrez le fichier et exécutez le script Python en exécutant la commande suivante dans le terminal :
- Appuyez et relâchez le bouton plusieurs fois.
- Observez le changement d'état du relais. Vous verrez que l'état du relais change chaque fois que vous appuyez sur le bouton.