Arduino Nano - LED - Clignotement Sans Délai
Imaginons que l'Arduino Nano ait deux tâches à accomplir : faire clignoter une LED et surveiller l'état d'un bouton qui peut être pressé à tout moment. Si nous utilisons la fonction delay() (comme discuté dans Arduino Nano - LED.), l'Arduino Nano pourrait ignorer certaines des pressions sur le bouton. En d'autres termes, l'Arduino Nano n'est pas capable de mener à bien la deuxième tâche.
Ce tutoriel vous explique comment faire clignoter une LED avec un Arduino Nano et détecter l'état d'un bouton sans manquer aucun événement de pression.
Nous passerons en revue trois exemples et comparerons les différences entre eux :
- Arduino Nano faisant clignoter une LED avec la fonction delay()
- Arduino Nano faisant clignoter une LED avec la fonction millis()
- Arduino Nano faisant clignoter une LED avec la bibliothèque ezLED
Cette méthode ne se limite pas seulement à faire clignoter une LED et à vérifier l'état du bouton. En général, elle permet à l'Arduino Nano d'exécuter plusieurs tâches simultanément sans se bloquer mutuellement.
Préparation du matériel
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
À propos des LED et des boutons
Si vous ne connaissez pas les LED et les boutons (y compris le brochage, les fonctionnalités et la programmation), les tutoriels suivants peuvent vous aider :
Diagramme de câblage
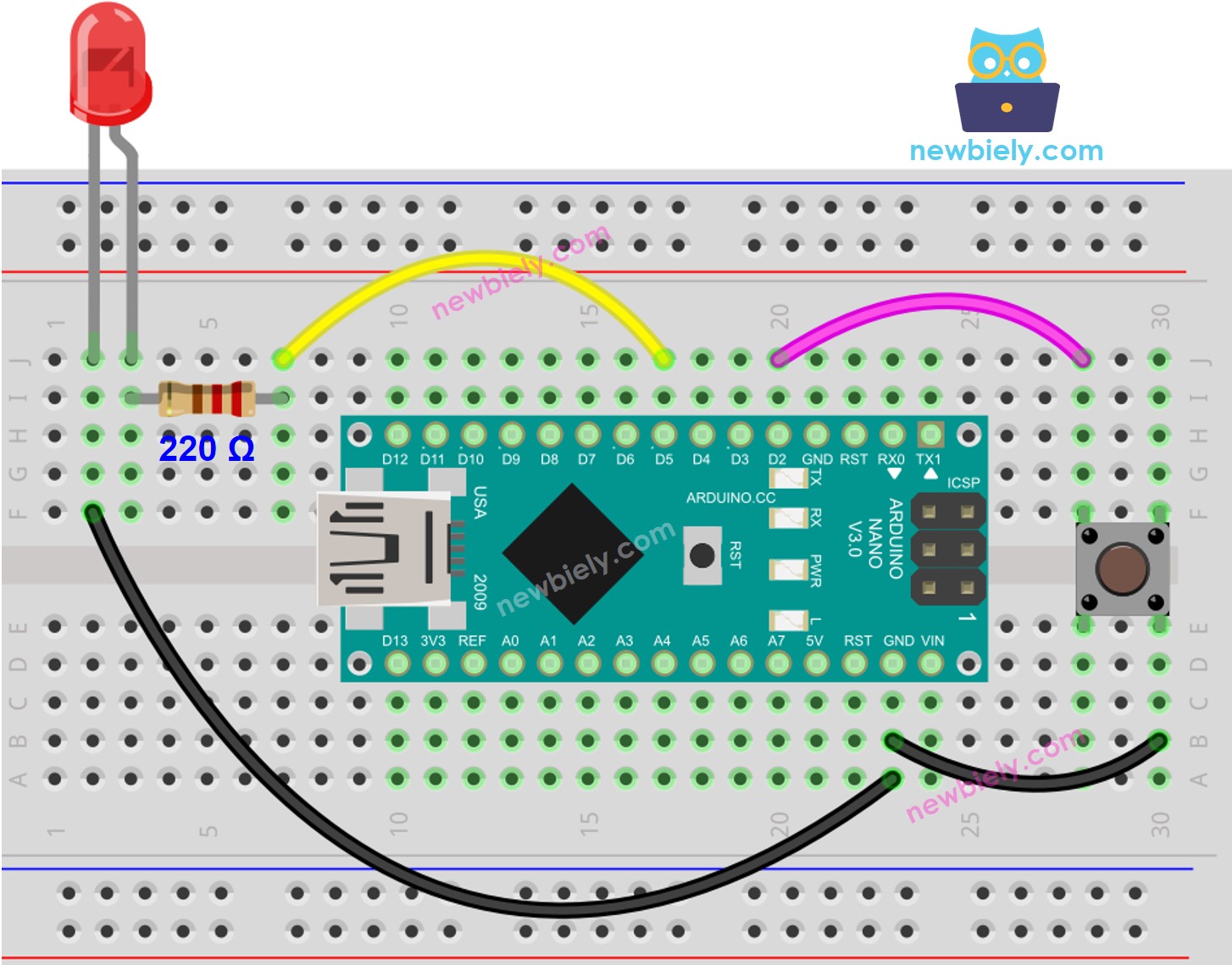
This image is created using Fritzing. Click to enlarge image
Code Arduino Nano - Avec Délai
Étapes rapides
- Connectez votre Arduino Nano à votre ordinateur via un câble USB.
- Lancez l'IDE Arduino, sélectionnez la carte et le port appropriés.
- Copiez le code et ouvrez-le dans l'IDE Arduino.
- Cliquez sur le bouton Upload dans l'IDE Arduino pour compiler et téléverser le code sur l'Arduino Nano.
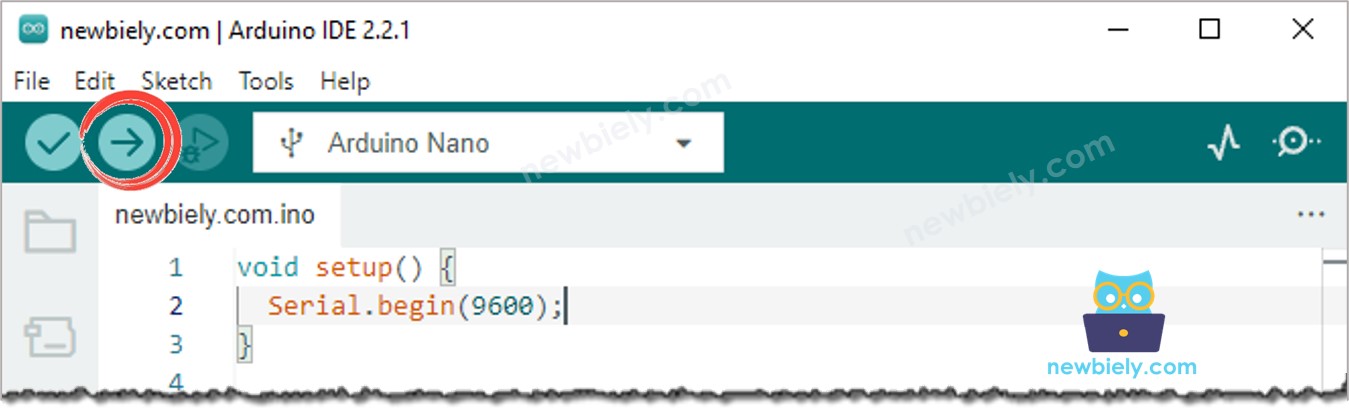
- Ouvrez le moniteur série.
- Appuyez quatre fois sur le bouton.
- Observez la LED ; elle alternera entre être allumée et éteinte toutes les secondes.
- Vérifiez la sortie dans le moniteur série.
- Sur le moniteur série, certains temps de pression n'ont pas été enregistrés. Cela est dû au fait que pendant le temps de délai, l'Arduino Nano est incapable d'effectuer des actions. En conséquence, il ne peut pas détecter l'événement de pression.
Code Arduino Nano - Sans Délai
Étapes rapides
- Exécutez le code et appuyez sur le bouton quatre fois.
- Observez la LED ; elle basculera entre ON et OFF à des intervalles d'une seconde.
- Vérifiez la sortie dans le moniteur série.
- Tous les cas de situations urgentes ont été identifiés.
Explication du code
Découvrez l'explication ligne par ligne contenue dans les commentaires du code source !
Ajout de tâches supplémentaires
Le code Arduino Nano ci-dessous fait :
- Fait clignoter deux LED à des intervalles différents.
- Vérifie l'état du bouton.
Vidéo
Extensibilité
Cette méthode peut être utilisée pour permettre à l'Arduino Nano d'exécuter plusieurs tâches simultanément, sans qu'une tâche n'empêche la progression des autres. Par exemple, envoyer une requête sur Internet et attendre la réponse, tout en faisant clignoter simultanément quelques indicateurs LED et en surveillant le bouton d'annulation.